History Object in React Router
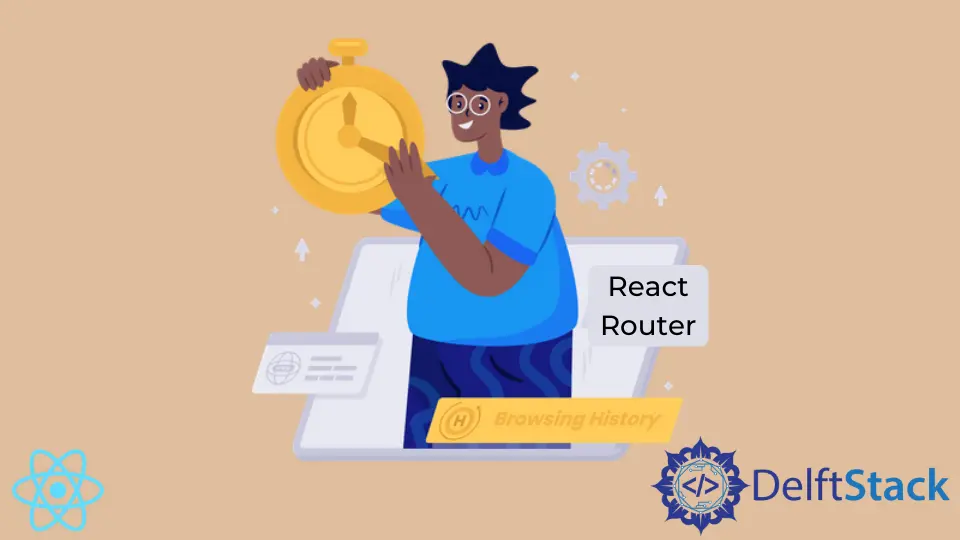
Navigation is an essential part of any modern application. JavaScript-based frameworks usually rely on default history object to be a foundation for their different navigation solutions. This object is available as a property of the DOM’s Window object.
When developing applications in React, developers can use React Router library. It provides all the navigation functionalities, including a history
package, an improved version of the browser history interface. React Router history
object includes many properties and methods that we can use to configure navigation, such as action
, location
, .push()
, or .replace()
. We’ll review how to best use these properties and methods to handle key navigation needs in the sections below.
React Router History
The history
package (or just history
for short) is the key tool required for managing session history in the JS. As mentioned before, it includes many methods, all of which are useful in their own way. Still, the history.push()
method is arguably the most important and will be the main focus of our guide.
History Stack
Browsers keep track of different URLs visited by users. This session history is called history stack, and it’s necessary for the browsers’ back or forward buttons to work.
History objects have properties and methods that can affect a history stack. For instance, the .replace()
method replaces the most recent path on the history stack. The .length
property gives us the number of entries in the stack.
The .push()
method is probably the most important and widely used. Developers use it to push an entry into the stack, redirecting users to another page. This method is essential, and we’ll discuss it in detail in later parts of the guide.
location
The location object shows the information about the current (and sometimes past) pathname of the app. React Router makes this object available multiple times. For instance, it can be accessed in the Route
component as a property of the props
object. Learn more on the official React Router documentation.
The history
object also has location
property. However, the history
object is mutable, so using the value of the location
property is not recommended. Instead, you can access the location
through the props of <Route>
. This way, you can be sure that you’re accessing the correct location
information.
React Router History for Redirects
When developing an application, you often need to change the user’s path after they perform an action. To do this, you need to set up an event handler and use the .push()
method to redirect to a specific pathname.
history.push()
in React Router V5
Since the introduction of hooks, functional components have become a lot more useful. With the release of v5, react-router-dom
also started to provide a useHistory()
hook to access history object more easily. Let’s look at a practical example:
import { useHistory } from "react-router-dom";
function Homepage() {
let historyObj = useHistory();
function handleClick() {
historyObj.push("/");
}
return (
<div>
...
<button onClick={() => handleClick()}>
Go to Homepage
</button>
...
</div>
);
}
In this example, we have a simple Homepage
component. First, we import the useHistory
hook from the react-router-dom
package. You must install version 5 of react-router-dom
(or higher) for this to work. The hook returns the component’s history
object, stored in the historyObj
variable.
We can use the aforementioned .push(string)
method in the handler function, which accepts one string argument. It pushes the string to the history stack and takes the user to the specified path.
history.push()
in React Router V4
Version 4 of React Router doesn’t include a useHistory
hook, so you’ll have to pass down the history
object via props
. This is also the only way to access history
in Class Components, which aren’t compatible with hooks.
You can navigate to another path by using a .push()
method in the handler function. Let’s take a look at this example:
function handleClick() {
this.props.history.push('/')
}
To access the history
object through props, you must ensure that your component has access to it. There are two ways to do so.
- Use the
component
attribute on<Route>
to link it with a specific component. For instance:
<Route path="/" component={Homepage} />
In this case, the Homepage
component will have access to the history
object via props.
- Use the
render
attribute to define a function. Provide props and use the following syntax:
<Route path="/" render={(props) => <Homepage {...props} />}/>
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn