How to Programmatically Navigate Using React Router DOM
- Navigate Declaratively vs. Navigate Programmatically Using the React Router DOM in React
-
Use the
history
Object to Navigate Programmatically in React
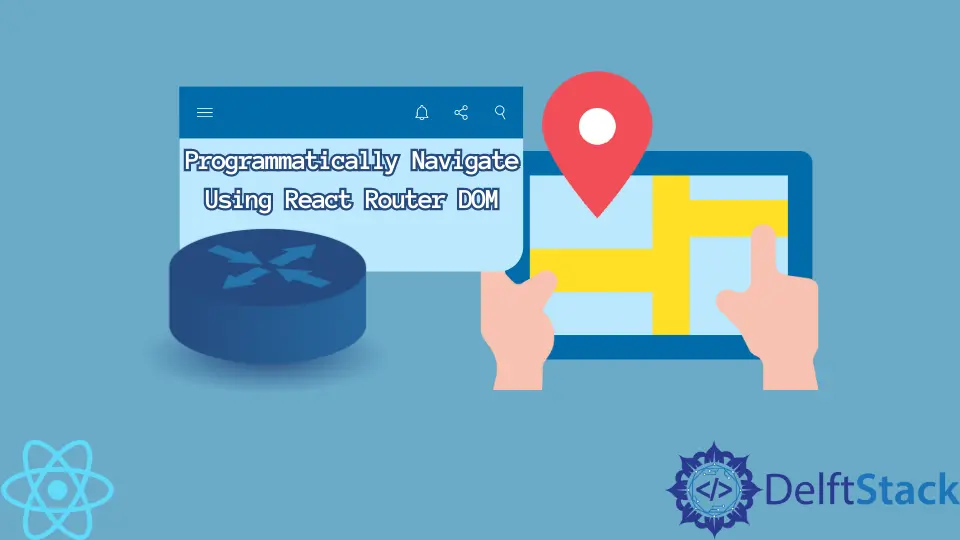
React is famous for its declarative style. Of course, methods play an important role as well.
It’s the combination of abstractions and functionality of methods that makes React so powerful.
Most popular JavaScript libraries today use a declarative approach to building interfaces. React was special because it allows you to build UIs without manipulating the DOM directly.
Despite the high level of abstraction, React still makes room for JavaScript expressions within JSX, the language used to build interfaces.
Navigate Declaratively vs. Navigate Programmatically Using the React Router DOM in React
React Router is the most popular library used to navigate React applications. The library provides a <Link>
component, which allows developers to declaratively create links that allow you to navigate to a specific URL seamlessly.
Most of the time, you want to change the URL after clicking a link. However, sometimes this default behavior is insufficient, and you, the programmer, might need to redirect the user to another URL.
React Router also leaves the door open to programmatic navigation. Developers can navigate programmatically by manipulating the history stack.
The library follows the concept of client-side routing and allows the developers to bypass the browser’s default behavior and change the URL without sending a request to the server.
Use the history
Object to Navigate Programmatically in React
To navigate the React application programmatically, first, you must access the history
object. There are two ways to access this object: receive it as a prop from the BrowserRouter
component, or use the .useHistory()
hook.
The history
object can have many different applications. In this case, we care about the three methods it provides: push()
, pop()
, and replace()
.
React developers can use these to manipulate the URL programmatically.
The .push()
is the most common and useful method for programmatically changing the URL. Let’s know how to perform this operation in different versions of React Router.
Programmatically Change the URL in React-Router 4.0.0+
This version does not include the useHistory
hook, so the only option is to access the history
object through props. You can’t do this in any React class component.
The component must be rendered by a <BrowserRoute>
(often shortened as <Route>
) component to access the history
prop.
Let’s look at an example.
export default class Sample extends Component {
render() {
return (
<Router>
<Route path="/" component={App}></Route>
</Router>
);
}
}
class App extends Component {
render() {
return (
<button onClick={() => this.props.history.push("samplePath")}>
Change the URL
</button>
);
}
}
We have one element in the App
component, a button with an event listener. We access the history
object in our props and call its .push()
method with one argument: the path we want to navigate.
However, our component’s props object only includes the history object because a
You can check out the live demo and click the button on codesandbox.
Programmatically Change the URL in React-Router 5.1.0+
The core React library went through major changes when react-router
version 5.1.0 was released. React version 16.8 introduced powerful hooks that gave functional components many more features.
In response to these changes, the developers behind the react-router
version 5.1.0 introduced the useHistory()
hook, giving functional components easy access to the history
object.
Let’s look at the example.
export default function Sample(props) {
return (
<BrowserRouter>
<Route>{<App></App>}</Route>
</BrowserRouter>
);
}
function App(props) {
let historyReference = useHistory();
return (
<button onClick={() => historyReference.push("boo")}>
Navigate Programmatically in React Router 5.1.0+
</button>
);
}
In this case, the parent Sample
component looks more or less the same as before. It has one child, the functional component App
.
The child is very different from the previous example. It’s a functional component, not a class component like before. And we don’t access the history
object through props; instead, we’re using the useHistory()
hook.
Please note that the history
object will not be accessible even with the hook if the component isn’t a custom <Route>
component child. The useHistory()
hook only changes how we access the history
object.
In the example above, we store the history
object, returned by the hook, in the historyReference
variable. Then we call the .push()
method on the variable and provide an argument to specify the path.
The useHistory()
hook is the easiest way to access the history
object when writing functional components.
If you prefer to stick with class components, you can still use the solution described in the previous section, even if the version of your react-router
installation is 5.1.0 or higher.
You can try editing the code and see what happens when you click the live demo.
Programmatically Change the URL in React-Router 6.0.0+
The latest versions of react-router
contain a new and improved useNavigate()
hook. It returns a function specifically made for programmatic navigation.
The most recent React Router documentation recommends using this hook to change the URL programmatically.
function NavigateApp(){
let navigate = useNavigate();
return (
<button onClick={() => navigate("boo")}>
Navigate Programmatically in React Router 6.1.0+
</button>
);
}
It works mostly the same way as the useHistory()
hook. Unlike the useHistory()
hook, there are some differences: it returns a function, not a reference to an object.
We store the function in the navigate
variable to call it directly instead of calling an object’s .push()
method. It takes the same argument as the method above, a string to specify the destination path.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn