How to Render HTML String in React
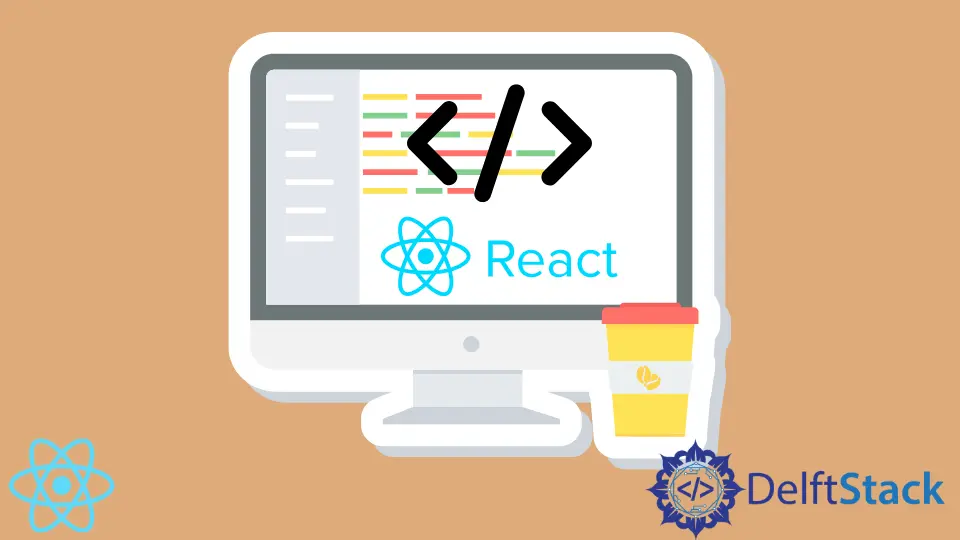
We will introduce how to render HTML string and render escaped HTML string in React.
Render HTML String in React
When working on a big-scale website, we need to reuse HTML components. Or, when working with JSON responses in HTML, we need to render HTML strings in React.
Let’s have an example and render a normal HTML string. First, we will create a div
with an id root
.
# react
<div id="root"></div>
Then, let’s render HTML string to root
.
# react
class App extends React.Component {
constructor() {
super();
this.state = {
TextRender: '<h1>This is a heading</h1><p> This is a paragraph.</p>'
}
}
render() {
return (
<div dangerouslySetInnerHTML={{ __html: this.state.TextRender }} />
);
}
}
ReactDOM.render(<App />, document.getElementById('root'));
Output:
In the example above, we rendered an HTML string. But if we try to render an escaped HTML string, it will give an error.
Let’s try to render an escaped HTML string.
# react
class App extends React.Component {
constructor() {
super();
this.state = {
TextRender: '<h1>This is a heading</h1><p> This is a paragraph.</p>'
}
}
render() {
return (
<div dangerouslySetInnerHTML={{ __html: this.state.TextRender }} />
);
}
}
ReactDOM.render(<App />, document.getElementById('root'));
Output:
As you notice in the example above, HTML tags are not rendered correctly because of the escaped HTML string.
Now, we will create a DecodeHtml
function to convert escaped HTML string to a normal HTML string.
# react
DecodeHtml(input){
var a = document.createElement('div');
a.innerHTML = input;
return a.childNodes.length === 0 ? "" : a.childNodes[0].nodeValue;
}
So, the code should look like this.
# react
class App extends React.Component {
constructor() {
super();
this.state = {
TextRender: '<h1>This is a heading</h1><p> This is a paragraph.</p>'
}
}
htmlDecode(input){
var a = document.createElement('div');
a.innerHTML = input;
return a.childNodes.length === 0 ? "" : a.childNodes[0].nodeValue;
}
render() {
return (
<div dangerouslySetInnerHTML={{ __html: this.htmlDecode(this.state.TextRender) }} />
);
}
}
ReactDOM.render(<App />, document.getElementById('root'));
The output should look like this.
Output:
In this way, we can easily render escaped HTML strings.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn