How to Render Raw HTML With ReactJS
- Online HTML to JSX Converter
-
Use the Inbuilt
ReactDOM.render()
Method -
Use the
dangerouslySetInnerHTML
Method - Conclusion
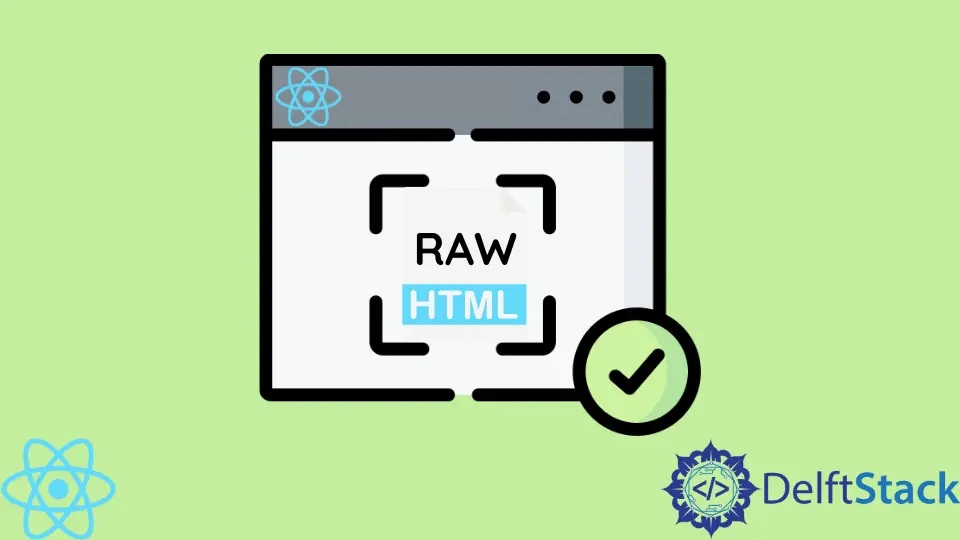
The versatility and flexibility of the React framework cannot be over-emphasized. You don’t even need to learn to React too deeply before you can pick it up as a developer, thanks to its ability that allows users to render HTML codes inside React.
For those who have written lots of code in HTML and are looking for the fastest way to bring it over to React, we’ll visit different means to do this in this tutorial.
Online HTML to JSX Converter
This method brings memories of when I first discovered online media converting websites when movies and songs were difficult to download.
The website ("https://transform.tools/html-to-jsx"
) converts pure HTML code into JSX (JavaScript Syntax Extension), so it becomes usable inside the react framework.
Code Snippet - App.js
:
import logo from './logo.svg';
import './App.css';
function App() {
return (
<div className="App">
<>
<meta charSet="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" type="text/css" href="goog.css" />
<title>Google</title>
<header>
<nav className="navi">
<div id="left">
<ul>
<li>
<a href="#">About</a>
</li>
<li>
<a href="#">Stores</a>
</li>
</ul>
</div>
<div id="right">
<ul>
<li>
<a href="#">Gmail</a>
</li>
<li>
<a href="#">Images</a>
</li>
<li>
<a href="#">Social</a>
</li>
<li>
<button type="submit">Sign In</button>
</li>
</ul>
</div>
</nav>
</header>
<main id="content">
<div className="google-logo">
<img
src="googlelogo_color_272x92dp.png"
alt="google-logo"
id="logo"
width={272}
height={92}
/>
</div>
<div id="search-bar">
<input type="text" placeholder="Search or Type a URL" />
</div>
<div id="buttons">
<button type="submit">Google Search</button>
<button type="submit">I'm Feeling Lucky</button>
</div>
</main>
<footer>
<nav className="footer">
<div id="left-foot">
<ul>
<li>
<a href="#">Advertising</a>
</li>
<li>
<a href="#">Business</a>
</li>
<li>
<a href="#">How Search Works</a>
</li>
</ul>
</div>
<div id="right-foot">
<ul>
<li>
<a href="#">Privacy</a>
</li>
<li>
<a href="#">Terms</a>
</li>
<li>
<a href="#">Settings</a>
</li>
</ul>
</div>
</nav>
</footer>
</>
</div>
);
}
export default App;
We wrap the codes inside the .App
div, and then we ensure to wrap it inside fragments because it contains multiple elements.
Use the Inbuilt ReactDOM.render()
Method
This method lets you use the HTML codes in their purest form. The ReactDOm.render()
allows us to display the HTML codes we have specified.
Also, the ReactDOM.render()
is preinstalled once we create a new React App successfully, so there is no need for extra dependencies. The ReactDOM.remder()
is an ideal method for creating light react apps.
We head inside the index.js
file of the react project and declare a const myelement
.
Code Snippet - index.js
:
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
const myelement = (
<>
<title>Google</title>
<body>
<header>
<nav class="navi">
<div id="left">
<ul>
<li><a href="#">About</a></li>
<li><a href="#">Stores</a></li>
</ul>
</div>
<div id="right">
<ul>
<li><a href="#">Gmail</a></li>
<li><a href="#">Images</a></li>
<li><a href="#">Social</a></li>
<li><button type="submit">Sign In</button></li>
</ul>
</div>
</nav>
</header>
<main id="content">
<div class="google-logo">
<img src="googlelogo_color_272x92dp.png" alt="google-logo" id="logo" height="92" width="272"/>
</div>
<div id="search-bar">
<input type="text" placeholder="Search or Type a URL"/>
</div>
<div id="buttons">
<button type="submit">Google Search</button>
<button type="submit">I'm Feeling Lucky</button>
</div>
</main>
<footer>
<nav class="foot">
<div id="left-foot">
<ul>
<li><a href="#">Advertising</a></li>
<li><a href="#">Business</a></li>
<li><a href="#">How Search Works</a></li>
</ul>
</div>
<div id="right-foot">
<ul>
<li><a href="#">Privacy</a></li>
<li><a href="#">Terms</a></li>
<li><a href="#">Settings</a></li>
</ul>
</div>
</nav>
</footer>
</body>
</>
);
ReactDOM.render(myelement, document.getElementById('root'));
After we have declared our element, we come below to add the element inside the ReactDOM.render()
and ensure that we wrap the codes in fragment tags.
Use the dangerouslySetInnerHTML
Method
This is the most popular method to render HTML in a raw state on the react framework. This method alerts the user to the security vulnerability of this method.
The dangerouslySetInnerHTML
method allows attackers to store malicious scripts inside the data sent from the search of form submitting websites.
Code Snippet - App.js
:
import logo from './logo.svg';
import './App.css';
const App = () => {
const data =
<body>
<header>
<nav class="navi">
<div id="left">
<ul>
<li><a href="#">About</a></li>
<li><a href="#">Stores</a></li>
</ul>
</div>
<div id="right">
<ul>
<li><a href="#">Gmail</a></li>
<li><a href="#">Images</a></li>
<li><a href="#">Social</a></li>
<li><button type="submit">Sign In</button></li>
</ul>
</div>
</nav>
</header>
<main id="content">
<div class="google-logo">
<img src="googlelogo_color_272x92dp.png" alt="google-logo" id="logo" height="92" width="272">
</div>
<div id="search-bar">
<input type="text" placeholder="Search or Type a URL">
</div>
<div id="buttons">
<button type="submit">Google Search</button>
<button type="submit">I'm Feeling Lucky</button>
</div>
</main>
<footer>
<nav class="foot">
<div id="left-foot">
<ul>
<li><a href="#">Advertising</a></li>
<li><a href="#">Business</a></li>
<li><a href="#">How Search Works</a></li>
</ul>
</div>
<div id="right-foot">
<ul>
<li><a href="#">Privacy</a></li>
<li><a href="#">Terms</a></li>
<li><a href="#">Settings</a></li>
</ul>
</div>
</nav>
</footer>
</body>
;
return (
<div
dangerouslySetInnerHTML={{ __html: data }}
/>
);
}
export default App;
We first declare our component using const
and then wrap the HTML codes inside backticks. Then we declare the dangerouslySetInnerHTML
and wrap our data
component inside its curly braces.
Conclusion
The methods create an exciting path to becoming familiar with React without having to go through rigorous courses. Yet the downside of such an approach is that it results in stretchy codes, which somehow defeats the real reason for creating the react framework in the first place.
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn