How to Reload Component in React
- What Is the Reload Component in React
- Use Vanilla JavaScript to Reload Components
-
Use
useState
to Reload Components
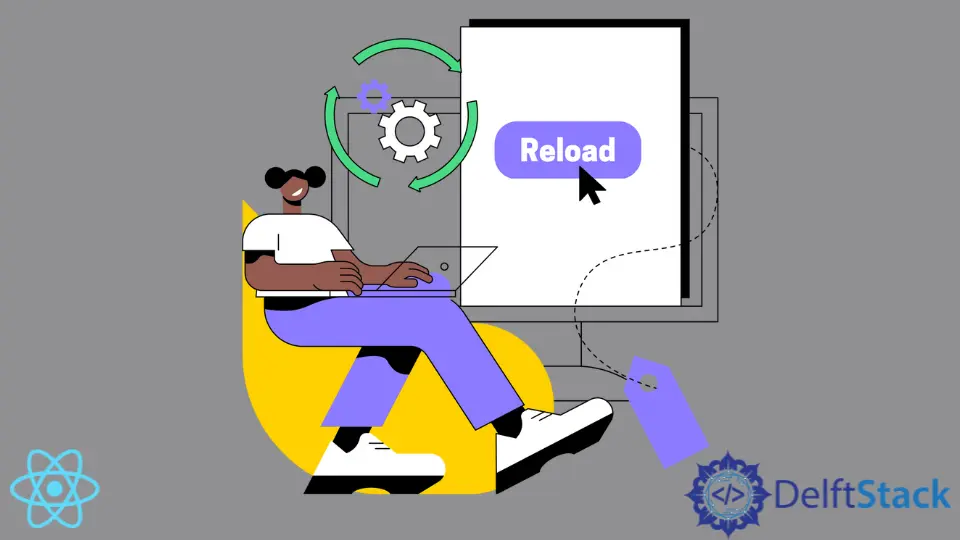
In the article, we will introduce how to reload a component in React with an example. We will also show how to reload a component whenever it is updated with an example.
What Is the Reload Component in React
While working on an extensive application, you may need to reload a specific component on a button click or when a change has been made in a component without completely reloading a whole webpage. React gives us two options in which we can reload a component.
Either we can reload a component using the Vanilla JavaScript
, or we can use the state
to reload the component whenever a change is made in the state of that component.
Use Vanilla JavaScript to Reload Components
First, we will create a new application and style it by importing a stylesheet.
So, let’s create a new application by using the following command.
# react
npx create-react-app my-app
Next, we switch to the application directory.
# react
cd my-app
Run npm-start
to check all dependencies are installed correctly.
# react
npm start
Import the stylesheet inside the app.
# react
import "./styles.css";
Create the App template.
# react
import "./styles.css";
export default function App() {
return (
<div className="App">
<h1>Hello Devs!</h1>
<h2>Click below to see some magic happen!</h2>
<button>
Click to reload!
</button>
</div>
);
}
Next, let’s style the app by adding style.css
.
# react
.App {
font-family: sans-serif;
text-align: center;
}
button{
background: black;
color: white;
border: none;
padding: 10px;
}
button:hover{
background: white;
color: black;
border: 1px solid black;
}
Output
We will use Vanilla JavaScript to reload the component whenever we click on this button.
The reload
method in Vanilla JavaScript tells the browser to reload the current page.
# react
window.location.reload(false);
This method has an optional parameter that, by default, is set to false
. We can also set it to true
if we look for a complete page refresh from the server.
One important thing to notice is that when we use the value as false
it will reload the cached version of the page, but when we set the value to true
, it will not refresh the page from the cached version of the page.
Once this is done, we can create a function reloadComponent
and use Vanilla JavaScript to reload the component and assign this function to the onClick
event of the button we created in the template.
So our code in App.js
will look like below.
import "./styles.css";
export default function App() {
function reloadComponent(){
window.location.reload(false);
}
return (
<div className="App">
<h1>Hello Devs!</h1>
<h2>Click below to see some magic happen!</h2>
<button onClick={reloadComponent}>
Click to reload!
</button>
</div>
);
}
Output
The page is not refreshed when we click on the button, but only the react component is refreshed.
Use useState
to Reload Components
When a user updates the component with some value or with multiple values, and we want to display the values selected or entered by a user in that component in real-time, we need to use a component reload to fetch the data and show it to the user whenever the data is updated.
Here we build a Todo-App
that can add entries to the list without refreshing the page.
We start by creating a new application by using the following command.
# react
npx create-react-app my-app
Next, we switch to the application directory.
# react
cd my-app
Run npm-start
to check all dependencies are installed correctly.
# react
npm start
Import the stylesheet inside the app.
# react
import "./styles.css";
Import React
and useState
in App.js
.
# react
import React, { useState } from "react";
Next, we define our constants todo
and setTodo
using useState
, and in return
, we will create a template that will contain 4 task buttons.
When we click on any task buttons, it will add that task in the task list displayed, and the component will be reloaded. We will add the onClick
function to all buttons addTodo
.
import React, { useState } from "react";
export default function TodoApp() {
const [todo, setTodo] = useState([]);
return (
<div className="app">
<div className="Todoitems">
<button value="Task1" onClick={addTodo}>
{" "}
Do Task 1
</button>
<button value="Task2" onClick={addTodo}>
{" "}
Do Task 2
</button>
<button value="Task3" onClick={addTodo}>
{" "}
Do Task 3
</button>
<button value="Task4" onClick={addTodo}>
{" "}
Do Task 4
</button>
</div>
<div className="todo">
Todo List
<ul></ul>
</div>
</div>
);
}
Create a function addTodo()
to add a task selected by the user.
# react
function addTodo(e) {
const todoItem = e.target.value;
console.log(todoItem);
setTodo([...todo, todoItem]);
}
Map the to-do list inside our div todo
.
<div className="todo">
<h2>Todo List</h2>
<ul>
{todo.map((item) => (
<li key={item}>{item}</li>
))}
</ul>
</div>
Now let’s add some styling in style.css
.
# react
.App {
font-family: sans-serif;
text-align: center;
}
button{
background: black;
color: white;
border: none;
padding: 10px;
margin-left: 10px;
}
button:hover{
background: white;
color: black;
border: 1px solid black;
}
.Todoitems{
text-align: center;
}
Output
As you can see in the above example, when we click on any task button, it reloads the component and updates the values of the to-do list without refreshing.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn