How to Respond to Window Resize Event in React
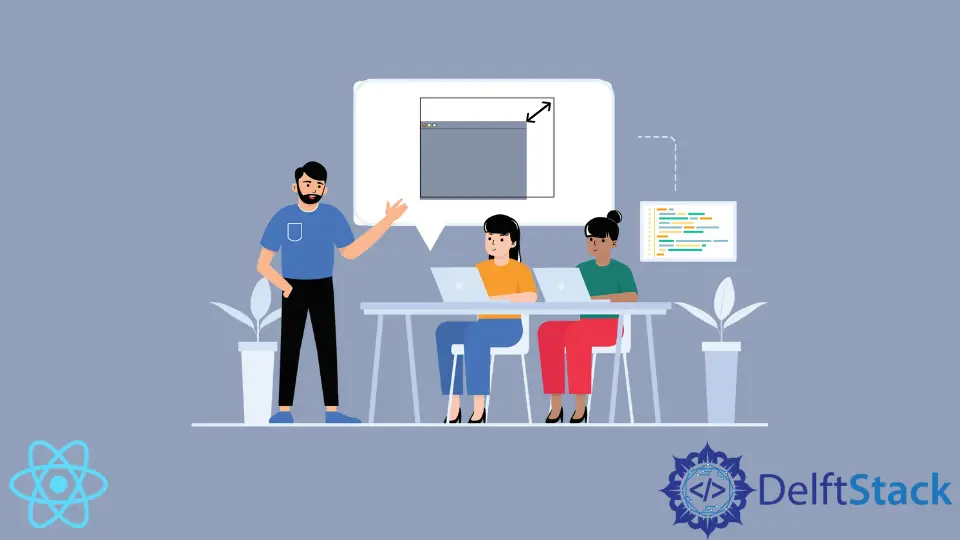
In this article, we will teach you how to set up a React component to change website content when the window dimension changes.
Window Resize in React
Sometimes React developers also need to respond whenever the user resizes the window. The response can be different, like adjusting element size, font size, changing website content, or aspect of its appearance.
In some cases, it may be necessary to re-render components in response to window resizing.
Re-render Functional Components on Window Resize
Since React updated version 16.8, functional components can use hooks. We can create a custom hook that will be called every time it detects the resize
event.
The custom hook would look something like the following.
import React, { useLayoutEffect, useState } from 'react';
function useWindowSize() {
const [windowSize, setWindowSize] = useState([0, 0]);
useLayoutEffect(() => {
function updateWindowSize() {
setWindowSize([window.innerWidth, window.innerHeight]);
}
window.addEventListener('resize', updateWindowSize);
updateWindowSize();
return () => window.removeEventListener('resize', updateWindowSize);
}, []);
return windowSize;
}
You can use this custom hook to store window dimensions as state values. Therefore, state values will update whenever window size changes, triggering the re-render.
Let’s look at a possible way to use the useWindowSize
hook.
Code Example:
import "./styles.css";
import React, { useLayoutEffect, useState } from "react";
export default function App() {
const dimensions = useWindowSize();
console.log("re-rendered");
return (
<div className="App">
<h1>{"width: " + dimensions[0] + " height:" + dimensions[1]}</h1>
</div>
);
}
function useWindowSize() {
const [windowSize, setWindowSize] = useState([0, 0]);
useLayoutEffect(() => {
function updateWindowSize() {
setWindowSize([window.innerWidth, window.innerHeight]);
}
window.addEventListener("resize", updateWindowSize);
updateWindowSize();
return () => window.removeEventListener("resize", updateWindowSize);
}, []);
return windowSize;
}
Here, we have a simple web app with the useWindowSize()
hook, defined right after it. This hook returns an array with two items, the first is width, and the second is height.
Therefore, when we call this hook in the App
component, we display these values accordingly.
Output:
Re-render Class Components on Window Resize
You can use the same principle in class components - store window height and width in a state variable. However, in this case, we need to split some of the code from useEffect()
hook between componentDidMount()
and componentDidUpdate()
lifecycle hooks.
When the component is mounted, we add an event listener and tell it to call the event handler we defined in the constructor. We also set the componentDidUpdate()
lifecycle hook to call handleResize()
every time the component is updated or re-rendered.
class App extends Component {
constructor(props) {
super(props);
this.state = {
dimensions: []
}
this.handleResize = this.setState({dimensions: [window.innerWidth, window.innerHeight]})
}
componentDidMount() {
window.addEventListener("resize", handleResize());
}
componentDidUpdate() {
handleResize()
}
render() {
return (
<div>{"width: "+ {this.state.dimensions.width} + " " + "height: " + {this.state.dimensions.height}}</div>
);
}
}
Unlike functional components, here we use the setState()
method to update the state, which takes one argument - the new state object. Hooks are not supported in React Class components.
In JSX, we reference state variables via the this
keyword, which refers to the instance of a class. Otherwise, everything works similarly to functional components.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn