How to Pass Data From Child Component to Parent in React
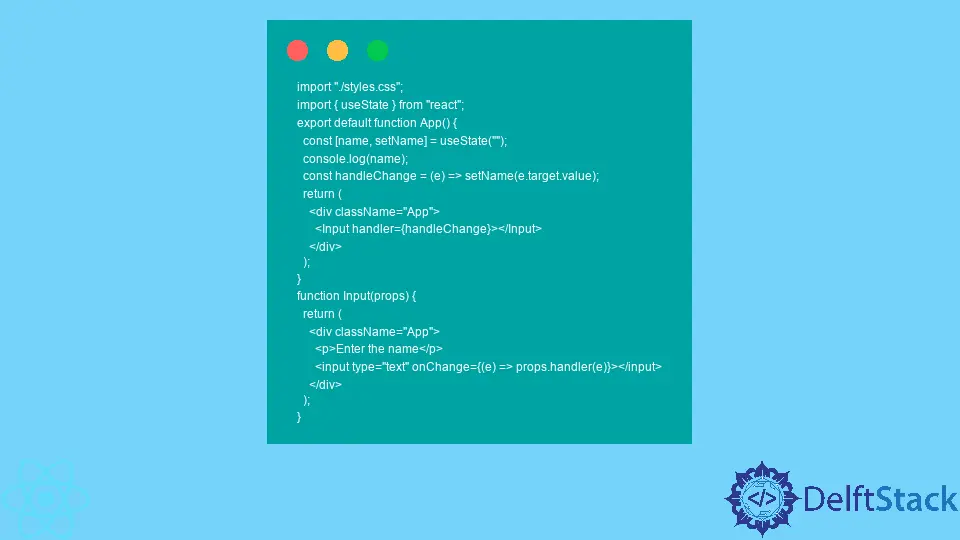
React is the most popular front-end library for multiple reasons. Reusability of components is one of the biggest advantages of React.
The library allows you to break down your web application into very small bits of code, each with its functionality and design. Then you can use these smaller components to build larger components, and so on.
React applications are essentially a component tree, with a parent component at the top. Since the reusability of components is so important, there needs to be a way to share data between React components.
Pass Data From Child Component to Parent in React
In React, it’s good to have a single source of truth - usually the component at the top of the tree. React developers can use props
objects to manually pass down data from the parent to its children.
Children components can access the value passed down to them and pass it down to their children. This way, the data from the parent component can be passed down multiple levels down the component tree.
Sometimes children’s components contain input elements, such as text fields, checkboxes, radios, and dropdowns. If you want to store the values from input elements, you need to get these values from the children’s components to the parent.
Parent components can use props
to pass down callback functions to their children. In other words, you can define a function (usually an event handler) in the parent component and pass it down as a callback function.
In essence, you can use the callback function to handle the event in the child component and pass the value from the input element as an argument to the callback function passed down as a prop
. The function will execute and update the state in the parent component.
Let’s take a look at this example.
import "./styles.css";
import { useState } from "react";
export default function App() {
const [name, setName] = useState("");
console.log(name);
const handleChange = (e) => setName(e.target.value);
return (
<div className="App">
<Input handler={handleChange}></Input>
</div>
);
}
function Input(props) {
return (
<div className="App">
<p>Enter the name</p>
<input type="text" onChange={(e) => props.handler(e)}></input>
</div>
);
}
The live demo shows that the callback is called in the child component, but it executes in the parent component and updates its state.
It is a basic example where we call the useState()
hook to initialize the name
state variable to an empty string. We also create the setName
function to update it.
Our handlechange
function takes one argument, a synthetic event, and passes the current value to the name
state variable in the textbox. The event handler is not called in the parent component.
It is defined there but passed down as a handler
prop to the child Input
element and called there.
We call the console.log()
function in the parent component to log out the state variable. You’ll see in the console that the value of the state variable is updated.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn