How to Get URL Parameter Values From Query String in React
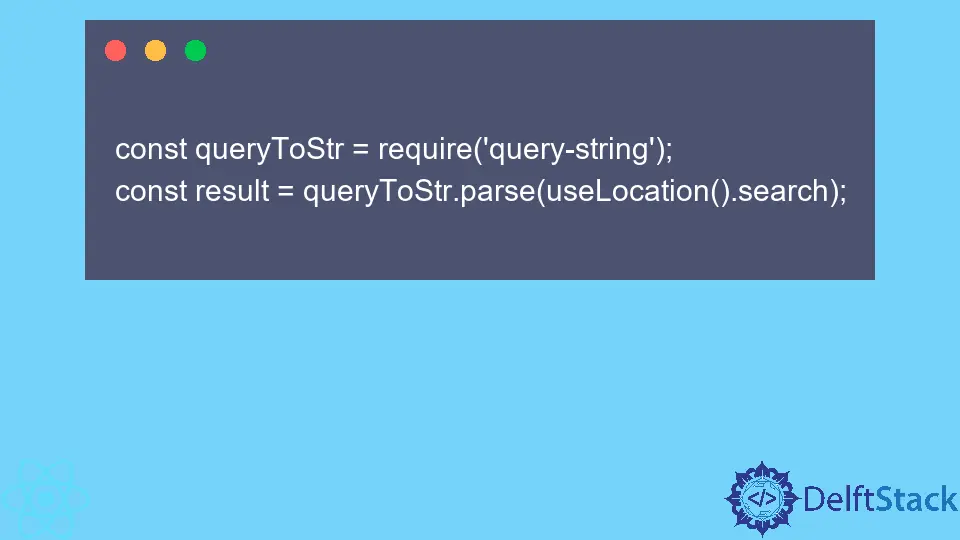
Throughout the process of creating a React app, you might occasionally need to extract a parameter value from the query string. This will allow you to reference the parameter
value throughout your app.
With the release of React Router v4 in 2017, the parsed this.props.location.query
property was removed. Developers had to find a new approach to read the parameter value from query strings. In this article, we’ll take a look at the solutions for old and newer versions of React.
React Router V3
Extracting the parameter value from the query
string in React Router v3 is easy. This version of React does all the work for you and provides the parsed location as a prop. If you check the value of the following object:
this.props.location.query
You’ll find that it contains the key
-value
pairs of all parameters in your query. (Query is the part of your URL that comes after ?
sign)
If the query
object doesn’t include the parameters that you’re looking for, then you should also check out path
parameter values, which are accessible at:
this.props.match.params.redirectParam
As long as you’re running an app with React Router v3 installed, your components accept props
, you can get parameter values from query
strings and path
.
Starting from v4, this.props.location.query
object was removed. The main reason for its removal was to allow developers to create unique ways to store query
strings.
React Router V4 and React Router V5
queries
in React Router v4 are still accessible, but developers must convert it into a string on their own. Libraries like qs
and query-string
were created to solve this exact problem.
In newer React Router versions, you can parse your query
object using the updated interface and the query-string
library.
const queryToStr = require('query-string');
const result = queryToStr.parse(this.props.location.search);
for Class Components
The above example applies to class components in React.
Using the libraries to parse this.props.location.search
can return a parsed query
object, with key
-value
pairs of parameters and their values.
for Functional Components
For the past few years, React community has started to give up class components in favor of functional components. The main reason behind the switch was the functional components’ new ability to use React hooks.
When extracting parameter values from a query
string, instead of using the value of this.props.location.search
, use the instance of useLocation()
hook.
The hook does not return parsed query
object. You still need to parse the instance of the hook to get key
-value
pairs of parameters and their values.
If you run the application with v4 (or higher) version of React-Router, the following code should give you the desired result:
const queryToStr = require('query-string');
const result = queryToStr.parse(useLocation().search);
Thanks to the useLocation()
hook, this code looks much cleaner.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn