The hashRouter Component in React
- What is HashRouter?
- How to Implement HashRouter in Your React Application
- Benefits of Using HashRouter
- Common Use Cases for HashRouter
- Conclusion
- FAQ
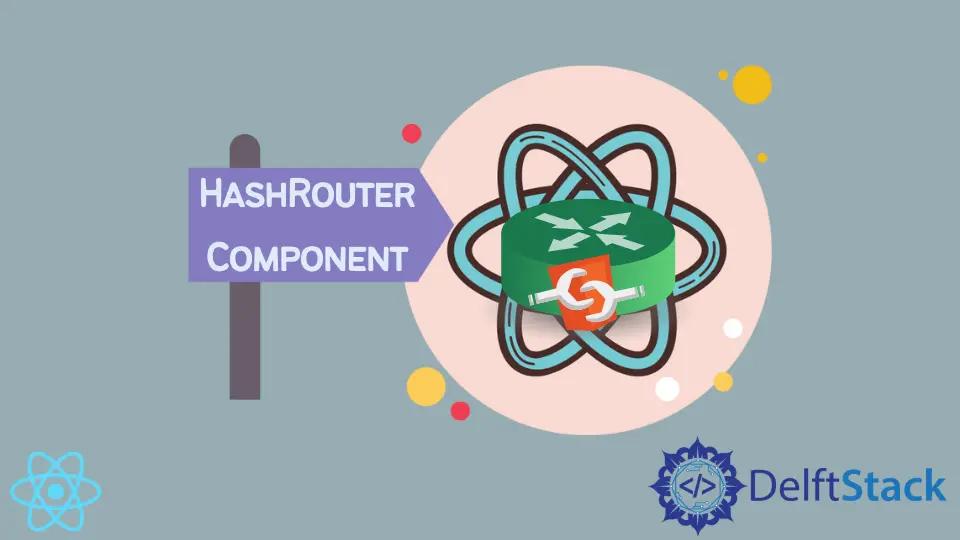
Navigating through a web application can sometimes feel like a maze, especially when the structure gets complex. Fortunately, React Router offers tools that simplify navigation, one of which is the HashRouter component. HashRouter is an essential part of the React Router library, allowing developers to manage routing in single-page applications using hash-based URLs. This component is particularly useful when you want to ensure that your application works seamlessly across different hosting environments, including static file servers that may not support server-side routing.
In this article, we will explore how HashRouter works, its benefits, and how to implement it effectively in your React applications.
What is HashRouter?
HashRouter is a routing component provided by the React Router library. It uses the hash portion of the URL (the part after the # symbol) to keep track of the user’s location within your application. This means that when users navigate through your app, the URL changes after the hash without causing a full page reload. The primary advantage of using HashRouter is its compatibility with static file servers that do not support dynamic routing. This makes it a preferred choice for applications hosted on platforms like GitHub Pages.
How to Implement HashRouter in Your React Application
To get started with HashRouter, you first need to install the React Router library if you haven’t already. You can do this using npm or yarn:
npm install react-router-dom
or
yarn add react-router-dom
Once you have the library installed, you can set up HashRouter in your application. Here’s a basic example:
import React from 'react';
import { HashRouter as Router, Route, Switch } from 'react-router-dom';
import Home from './Home';
import About from './About';
import NotFound from './NotFound';
function App() {
return (
<Router>
<Switch>
<Route path="/" exact component={Home} />
<Route path="/about" component={About} />
<Route component={NotFound} />
</Switch>
</Router>
);
}
export default App;
In this code, we import the necessary components from react-router-dom
. We define a basic App
component that uses HashRouter
to wrap our routes. The Switch
component ensures that only one route matches at a time, making it easier to handle 404 pages, as shown with the NotFound
component.
Output:
Application with HashRouter set up successfully
The Home
and About
components represent different pages in your application. When you navigate to /about
, the URL will update to http://yourdomain.com/#/about
, and the corresponding component will be rendered without a full page reload.
Benefits of Using HashRouter
Using HashRouter comes with several benefits that can enhance the user experience and simplify development. Here are some key advantages:
-
Simplicity: HashRouter is straightforward to implement and requires minimal setup. This makes it an excellent choice for smaller applications or prototypes.
-
Compatibility: Since HashRouter uses the hash portion of the URL, it works seamlessly with static file servers. This is particularly beneficial for developers who deploy their applications on platforms like GitHub Pages, where server-side routing is not available.
-
Client-Side Routing: HashRouter allows for client-side routing, meaning the application can manage navigation without reloading the entire page. This results in a smoother user experience.
-
SEO Considerations: While hash-based URLs are not as SEO-friendly as traditional URLs, they can still be useful for applications that do not require extensive search engine optimization.
-
State Management: HashRouter can help manage the application state as users navigate through different views, allowing for a more dynamic and interactive experience.
Common Use Cases for HashRouter
HashRouter is particularly suited for certain scenarios in web development. Here are some common use cases:
-
Single Page Applications (SPAs): If you’re building a SPA, HashRouter provides a simple way to manage routes without requiring server-side support.
-
Static Hosting: Applications hosted on static file servers can benefit from HashRouter, as it allows for routing without needing server configurations.
-
Prototyping: When building quick prototypes or demo applications, HashRouter’s simplicity makes it an ideal choice.
-
Cross-Browser Compatibility: Because HashRouter relies on the hash portion of the URL, it works consistently across different browsers, ensuring a uniform experience for users.
-
Lightweight Applications: For lightweight applications that do not require complex routing, HashRouter offers a minimalistic approach to navigation.
Conclusion
In summary, the HashRouter component in React is a powerful tool for managing routing in single-page applications, especially when hosting on static servers. Its simplicity, compatibility, and ease of use make it an excellent choice for developers looking to create seamless user experiences. Whether you’re building a small prototype or a larger application, understanding how to implement and utilize HashRouter can significantly enhance your development process. By leveraging HashRouter, you can ensure that your application is not only functional but also user-friendly.
FAQ
-
What is HashRouter in React?
HashRouter is a component from the React Router library that allows navigation using hash-based URLs, which is useful for single-page applications. -
When should I use HashRouter?
You should use HashRouter when hosting applications on static file servers or when you need a simple routing solution without server-side support. -
Is HashRouter SEO-friendly?
HashRouter is not as SEO-friendly as traditional URLs, but it can still be used effectively in applications that do not require extensive search engine optimization. -
Can I use HashRouter with other React Router components?
Yes, HashRouter can be used in conjunction with other React Router components like Route and Switch to manage navigation effectively. -
How does HashRouter handle 404 pages?
HashRouter can handle 404 pages by using the Switch component to render a NotFound component when no other routes match.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn