How to Use the if and if...else Statements in R
-
Syntax of the
if
Statement in R -
Correct Form of the
else
Statement in R -
Use Multiple
if
andelse
Statements in R
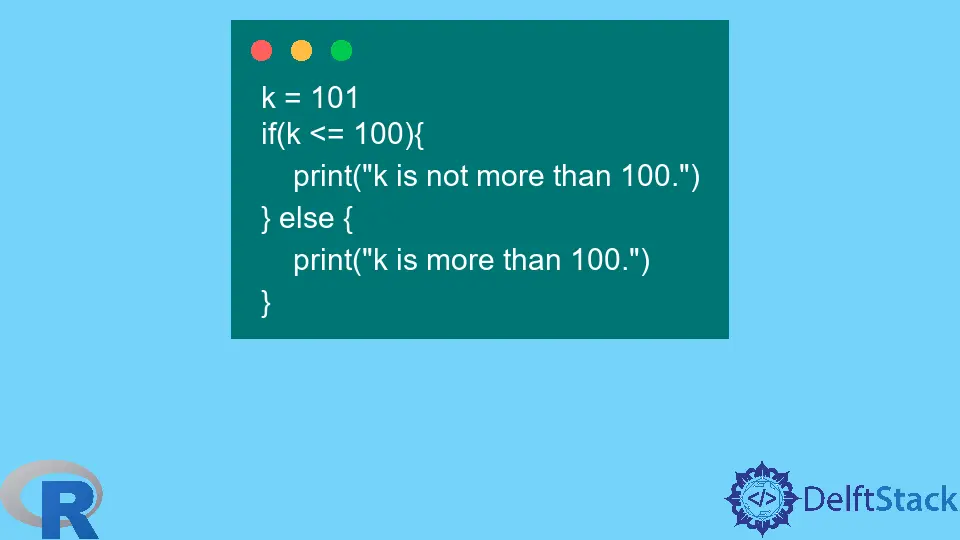
This article describes the correct syntax of R’s if
and if-else
conditional constructs.
Note that only the first element is used in the if
construct if it is based on rows of a dataframe or vector elements.
Syntax of the if
Statement in R
The valid forms of the if
construct are as follows:
if(condition)
statement to execute
if(condition){
statement 1
statement 2
}
Without parentheses, the if
block will only evaluate one statement or expression if the condition is true. The others will get executed even if the condition is false.
Example code:
j = 15
if(j < 12)
print(j+1)
print("This should not print if j >= 12.")
print(j)
Output:
> j = 15
> if(j < 12)
+ print(j+1)
> print("This should not print if j >= 12.")
[1] "This should not print if j >= 12."
> print(j)
[1] 15
The second statement after if
got evaluated because we did not use parentheses.
We need to use parentheses to evaluate multiple statements if the condition is true.
Example code:
j = 53
if(j < 46){
print(j+1)
print("This should not print if j >= 46.")}
print(j)
Output:
> j = 53
> if(j < 46){
+ print(j+1)
+ print("This should not print if j >= 46.")}
> print(j)
[1] 53
Correct Form of the else
Statement in R
The else
keyword must be placed on the same line as the end of the expression that follows if
. The following code raises an error.
Example code:
j = 18
if(j < 28)
print(j)
else
print("Not lower than 28.")
Output:
> j = 18
> if(j < 28)
+ print(j)
[1] 18
> else
Error: unexpected 'else' in "else"
> print("Not lower than 28.")
[1] "Not lower than 28."
The correct form is demonstrated below.
Example code:
j = 8
if(j < 15)
print(j) else
print("Not less than 15.")
Output:
> j = 8
> if(j < 15)
+ print(j) else
+ print("Not less than 15.")
[1] 8
Using parentheses helps make our code more readable and avoids mistakes. Now, place the else
keyword on the same line as the closing parentheses following the if
block.
Example code:
k = 101
if(k <= 100){
print("k is not more than 100.")
} else {
print("k is more than 100.")
}
Output:
> k = 101
> if(k <= 100){
+ print("k is not more than 100.")
+ } else {
+ print("k is more than 100.")
+ }
[1] "k is more than 100."
Use Multiple if
and else
Statements in R
There are several points to consider when using a sequence of conditional constructs. These are common in most languages.
-
When using multiple conditions, the sequence in which we write them is significant.
-
If we use a chain of
if
andelse
statements, ending with anelse
statement, the statements corresponding to the first true condition or theelse
condition will get executed. -
If we use a sequence of
if
statements, more than one condition may evaluate to true. -
If we do not use a last
else
statement, none of the statements may be executed.
Example code:
jk = 155
# Wrong Order
if(jk < 300){
print("Less than 300.")
} else if (jk < 200) {
print("Less than 200.")
} else if (jk < 100) {
print("Less than 100.")
}
# Correct Order
if(jk < 100){
print("Less than 100.")
} else if (jk < 200) {
print("Less than 200.")
} else if (jk < 300) {
print("Less than 300.")
}
# Multiple conditions satisfied. No else statements.
if(jk < 100){
print("Less than 100.")
}
if (jk < 200) {
print("Less than 200.")
}
if (jk < 300) {
print("Less than 300.")
}
# Last else to cover remaining cases.
if(jk < 15){
print("Less than 15.")
} else if (jk < 25) {
print("Less than 25.")
} else if (jk < 35) {
print("Less than 35.")
} else {
print("Not less than 35.")
}
# NO last else; no condition may be met.
if(jk < 15){
print("Less than 15.")
} else if (jk < 25) {
print("Less than 25.")
} else if (jk < 35) {
print("Less than 35.")
}
Output:
> jk = 155
>
> # Wrong Order
> if(jk < 300){
+ print("Less than 300.")
+ } else if (jk < 200) {
+ print("Less than 200.")
+ } else if (jk < 100) {
+ print("Less than 100.")
+ }
[1] "Less than 300."
>
> # Correct Order
> if(jk < 100){
+ print("Less than 100.")
+ } else if (jk < 200) {
+ print("Less than 200.")
+ } else if (jk < 300) {
+ print("Less than 300.")
+ }
[1] "Less than 200."
>
> # Multiple conditions satisfied. No else statements.
> if(jk < 100){
+ print("Less than 100.")
+ }
> if (jk < 200) {
+ print("Less than 200.")
+ }
[1] "Less than 200."
> if (jk < 300) {
+ print("Less than 300.")
+ }
[1] "Less than 300."
>
> # Last else to cover remaining cases.
> if(jk < 15){
+ print("Less than 15.")
+ } else if (jk < 25) {
+ print("Less than 25.")
+ } else if (jk < 35) {
+ print("Less than 35.")
+ } else {
+ print("Not less than 35.")
+ }
[1] "Not less than 35."
>
> # NO last else; no condition may be met.
> if(jk < 15){
+ print("Less than 15.")
+ } else if (jk < 25) {
+ print("Less than 25.")
+ } else if (jk < 35) {
+ print("Less than 35.")
+ }
To use an if
condition on the values of dataframe columns, read about the Vectorized ifelse()
Function of R.