How to Convert UTC to CST in Python
-
Use the
dateutil.tz
Module to Convert the Date and Time From UTC to CST in Python -
Use the
pytz
Module to Convert the Date and Time From UTC to CST in Python -
Use the
zoneinfo
Module to Convert the Date and Time From UTC to CST in Python
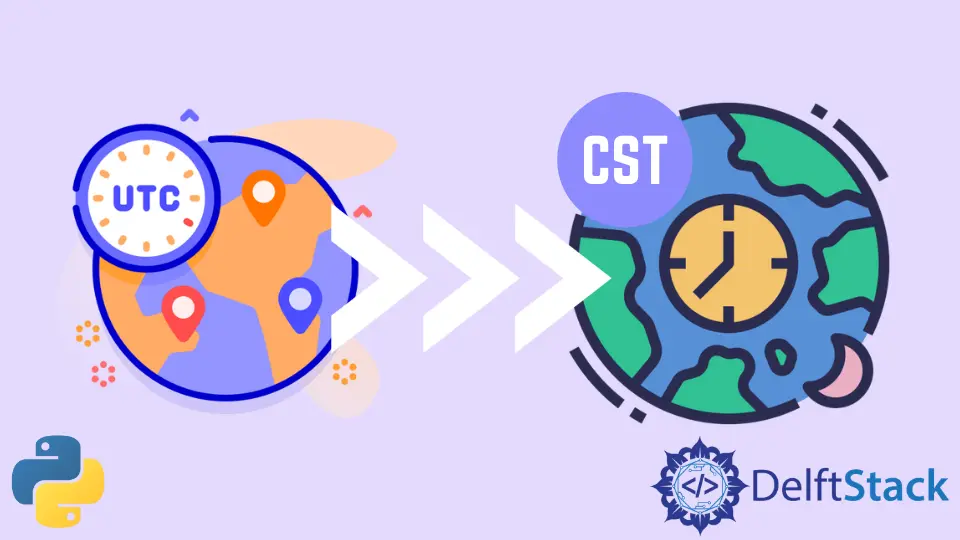
Python stores the date and time in a very sophisticated way. It has a data type specifically for storing this type of data. Moreover, Python also provides a datetime
module that offers several classes to manipulate date and time.
The conversion into different time zones is an essential part of manipulating date and time. In this article, we will discuss two such time zones, namely UTC and CST, and the different ways available to convert the former to the latter.
The datetime
module is a necessity and will need to be imported and used in all the methods mentioned in the article below.
Use the dateutil.tz
Module to Convert the Date and Time From UTC to CST in Python
The dateutil
module powers up the standard datetime
module by providing some significant extensions. The dateutil.tz
module supplies time zone implementations that sub-class the abstract datetime.tzinfo
type.
Here, we will frequently use the tz.gettz()
function, which essentially retrieves an object of the time zone from the given string passed as its argument.
The following code uses the dateutil.tz
module to convert the date and time from UTC to CST in Python.
import datetime
from dateutil import tz
from_zone = tz.gettz("UTC")
to_zone = tz.gettz("America/Chicago")
json_data = {"time": "2021-10-08T08:17:42Z"}
utc = datetime.datetime.strptime(json_data["time"], "%Y-%m-%dT%H:%M:%SZ")
utc = utc.replace(tzinfo=from_zone)
cst = utc.astimezone(to_zone)
print(utc)
print(cst)
The above code provides the following output:
2021-10-08 08:17:42+00:00
2021-10-08 03:17:42-05:00
Use the pytz
Module to Convert the Date and Time From UTC to CST in Python
The pytz
module supports a vast majority of time zones, making it the perfect module to provide date and time conversion functionalities and provide functions that enable simple time zone calculations in the programmer’s python application software.
Moreover, the pytz
module allows the creation of smart datetime
objects or instances that are aware of their time zone. The pytz
module needs to be manually installed and can be done by using the pip
command.
The following code uses the pytz
module to convert the date and time from UTC to CST in Python
from datetime import datetime, timezone
import pytz
fmt = "%Y-%m-%d %H:%M:%S %Z%z"
e = pytz.timezone("US/Central")
time_from_utc = datetime.fromtimestamp(1607020200, tz=timezone.utc)
time_from = time_from_utc.astimezone(e)
time_from.strftime(fmt)
time_to_utc = datetime.fromtimestamp(1609785000, tz=timezone.utc)
time_to = time_to_utc.astimezone(tz=pytz.timezone("US/Central"))
print(time_to_utc)
print(time_to)
The above code provides the following output:
2021-01-04 18:30:10+00:00
2021-01-04 12:30:10-06:00
Although both the dateutil.tz
and the pytz
modules are utilized to implement similar things in Python, they have some differences.
- The
pytz
module supports almost all time zones, significantly more than what thedateutil.tz
module offers. - The library of the
pytz
module is significantly more optimized. - The
pytz
module is historically faster thandateutil.tz
, but the gap has been lessening over the last couple of updates. - The use of
dateutil.tz
module is recommended in the earlier versions of Python when thepytz
module was not stable enough.
Use the zoneinfo
Module to Convert the Date and Time From UTC to CST in Python
With the introduction of Python 3.9 came the zoneinfo
module, which eliminated the need to install any other third-party libraries to manage time zones. The zoneinfo
module deals with everything itself.
The zoneinfo
module is utilized to provide a strong time zone implementation that, as specified in PEP 615
, can support the IANA
time zone database.
The following code uses the zoneinfo
module to convert the date and time from UTC to CST in Python.
# >= Python 3.9
from datetime import datetime
from zoneinfo import ZoneInfo
naive_datetime = datetime(2021, 10, 8, 12, 0, 0)
user_tz_preference = ZoneInfo("US/Central")
user_datetime = naive_datetime.replace(tzinfo=user_tz_preference)
user_datetime = datetime(2011, 10, 26, 12, tzinfo=ZoneInfo("US/Central"))
utc_datetime = user_datetime.astimezone(ZoneInfo("UTC"))
print(user_datetime.isoformat())
print(utc_datetime.isoformat())
The above code provides the following output:
# 2021-10-08T12:00:00-06:00
# 2021-10-08T19:00:00+00:00
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn