How to Use Requests Module to Post Form Data in Python
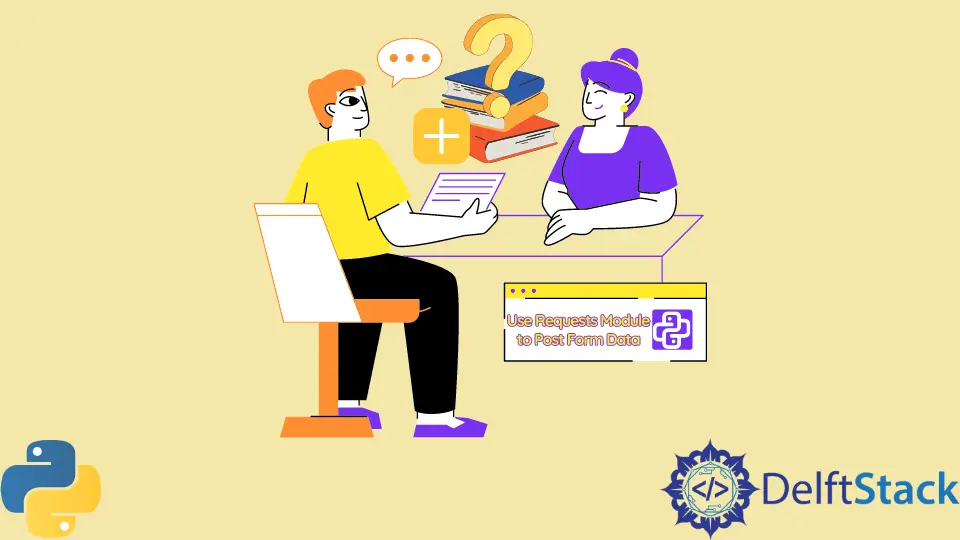
This tutorial describes the Python requests
module and illustrates how we can use this module to post form data in Python.
Use requests
Module to Post Form Data in Python
Python can be used to access webpages and also to post content to the webpages.
Whether there are different modules like httplib
, urllib
, httplib2
etc., the requests
module in Python is the simplest and can write powerful programs involving GET
and POST
methods.
The requests
library is one of the main aspects of Python for creating HTTP requests to a defined URL.
Introduction to POST
Request Method
The World Wide Web HTTP supports the POST
request method. When considering the format, the POST
request method requests that a web server accepts the data included in the body of the request message, apparently storing them.
We often use it to upload a file or submit a finalized web form. For example, the post()
sends a POST
request to a specified URL. We can also use it when we need to send some data to the server.
Key Facts About POST
Request
- It does not have any restraints on data length and is set as needed.
- It does not live in the browser history.
- It is never cached.
- It cannot be a bookmark.
Installation of requests
Module in Python
The requests
module that is needed to use the post()
method can be installed using the following command:
python -m pip install requests
OR
pip install requests
We can use the below command if pipenv
manages Python packages.
pipenv install requests
Once the requests
module gets installed, it can be imported and used in the program as follows:
import requests
Now, how to use requests.post()
method? What is the syntax for it? Let’s learn it below.
Syntax:
requests.post(url, data={key: value}, json={key: value}, args)
Here, args
is equal to 0
or more of the named arguments as the below parameters:
Parameter | Description |
---|---|
url |
The URL of the request is an important part. |
data |
Optional. A dictionary, tuples’ list, bytes or a file object is sent to the specified URL. |
json |
Optional. A JSON object to direct to the specified URL. |
files |
Optional. A directory of files to pass to the specified URL. |
allow_redirects |
Optional. A Boolean to enable or disable redirection. The default value is True , which means allowing redirects. |
auth |
Optional. A tuple to enable a specific HTTP authentication. The default value is None . |
cert |
A string or tuple specifying a cert file or key . Optional where the default value is None . |
cookies |
Cookie’s dictionary to send to the specified URL is optional, and the default value is None . |
headers |
Optional, Default None , a dictionary of HTTP headers to send to the defined URL. |
proxies |
Optional, Default None , a protocol dictionary to the proxy URL. |
stream |
A Boolean indication of the response, whether False (downloaded) or True (Streamed). Optional, Default False . |
timeout |
Optional, a number that indicates the time waited for the client to make a connection and/or send a response. The default value is None . It means the request will continue till the connection closes. |
verify |
A Boolean or string indication to verify the server’s TLS certificate/not. Optional. The default value is True . |
Applications of the post()
Method
The below code fence demonstrates the usage of the post()
method where the user posts data to the httpbin website through the post()
method and gets a response on how it is posted.
import requests
values = {"username": "user", "password": "test"}
result = requests.post("https://httpbin.org/post", data=values)
print(result.text)
Output:
A response object
is received, which contains lots of information. First, the form object
with the keys and values where username
is user
and password
is test
. Then the header object
contains some properties: Next
, JSON
, origin
and URL
.
To post a multipart-encoded file, make a multipart POST
request by sending a file to the server using the file property
of the post()
method.
import requests
files = {"file": open("test.txt", "rb")}
url = "https://httpbin.org/post"
result = requests.post(url, files=files)
print(result.text)
Output:
As the output, an empty data object was returned and got the files object, a file attribute whose value is the file’s content (the content of the test.txt
file made in the working directory that was Test Text
).
To make POST
requests within a session, set a cookie to a URL and then make a request again to assess if the cookie is set. Sessions are also helpful when a user needs to send the same data across all requests. For example:
import requests
s = requests.Session()
s.cookies.update({"month-visit": "July"})
request01 = s.get("http://httpbin.org/cookies")
print(request01.text)
# outputs details on the "month-visit" cookie
request02 = s.get("http://httpbin.org/cookies", cookies={"year-visit": "2022"})
print(request02.text)
# outputs details on "month-visit" and "year-visit" cookie
request03 = s.get("http://httpbin.org/cookies")
print(request03.text)
# outputs details on the "month-visit" cookie
Output:
As in the output, the month-visit
session cookie is passed with all three requests. But the year-visit
cookie is passed only during the second request.
There is no year-visit
cookie in the third request. Therefore, this confirms that the cookies or other data set on individual requests won’t pass with other session requests.
When considering the benefits of the post()
, it is more secure than the GET
method because the user’s entered information is never visible in the URL query string or server logs.
There is a much larger limit on the data amount we can send, and a person can send a text or binary data like uploading a file using the POST
method.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.