How to Make an API Call With Token in Python
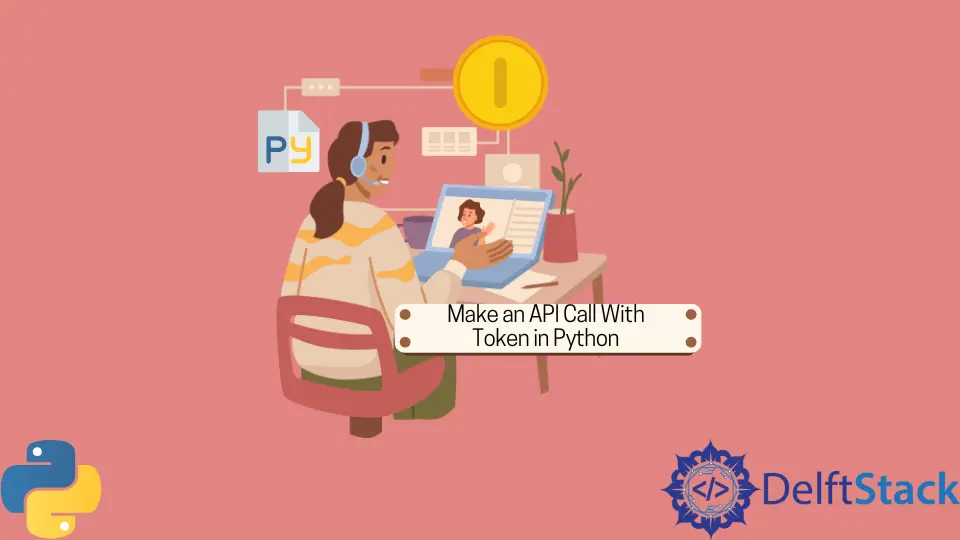
When we first came across the question of how to call an API in Python, our first thought was, “what is an API?”.
An API is an acronym for Application Programming Interface; it lets you access the software’s data without necessarily accessing it. For instance, if a cryptocurrency API is called, we will get access to crypto prices directly into our app without visiting the crypto website or app.
When we call an API in Python, we can do it with a token, and without a token, when we do it without a token, the API isn’t secure, but in the case of using tokens, it serves as a password and username to access the tokens.
We will look at examples of both cases.
Make API Call Without Token in Python
To kickstart, we will need to first install a Python library to handle this request; inside the terminal, type in pip install requests
, create a new file and name it new.py
. Then we will input these codes:
new.py
:
import requests
import json
class MakeApiCall:
def get_data(self, api):
response = requests.get(f"{api}")
if response.status_code == 200:
print("call successful")
self.formatted_print(response.json())
else:
print(f"error {response.status_code} in fetching request")
def get_user_data(self, api, parameters):
response = requests.get(f"{api}", params=parameters)
if response.status_code == 200:
print("data fetch success")
self.formatted_print(response.json())
else:
print(f"error {response.status_code} in fetching request")
def formatted_print(self, obj):
text = json.dumps(obj, sort_keys=True, indent=4)
print(text)
def __init__(self, api):
parameters = {"site": "stackoverflow"}
self.get_user_data(api, parameters)
if __name__ == "__main__":
api_call = MakeApiCall("https://api.stackexchange.com/2.2/info")
The first snippet handles the call; once the call is successful, it prints the result, then after the data has been fetched successfully, it prints the result; otherwise, it prints the error, then it should print the data that is fetched from the API.
Then the last line is the API link from which we are fetching the data. Once you click run, wait a few moments, and you will see the data fetched inside the terminal.
Make API Call With Token in Python
As we mentioned, making API with tokens means we make a secure connection to the API we are attempting to access. We need to install a few dependencies from pip
.
We will first need to generate a token and deploy jwt
for that. Inside the terminal, type pip install jwt
.
Open a new file, name it jwt.py
and type in the below code to generate a token.
jwt.py
:
import jwt
encoded_jwt = jwt.encode({"some": "payload"}, "secret", algorithm="HS256")
print(encoded_jwt)
Next, we will install requests
to handle API call requests. We will type in pip install requests
inside the terminal.
Then we create a file, name it new.py
and type in these codes:
new.py
:
import requests
BASE_URL = "https://www.boredapi.com/api/activity"
token = "your jwt token"
headers = {"Authorization": "Bearer {}".format(token)}
auth_response = requests.get(BASE_URL, headers=headers)
print(auth_response.json())
Click run, and you will see the data fetched from the API inside the terminal.
Conclusion
Ideally, when we want to do a more serious project, we should call API with a token for added protection. While jwt
offers a straightforward way to generate tokens, there are other ways we can generate tokens.
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn