Static Class in Python
- Understanding Static Methods in Python
- Using Class Methods in Python
- When to Use Static and Class Methods
- Conclusion
- FAQ
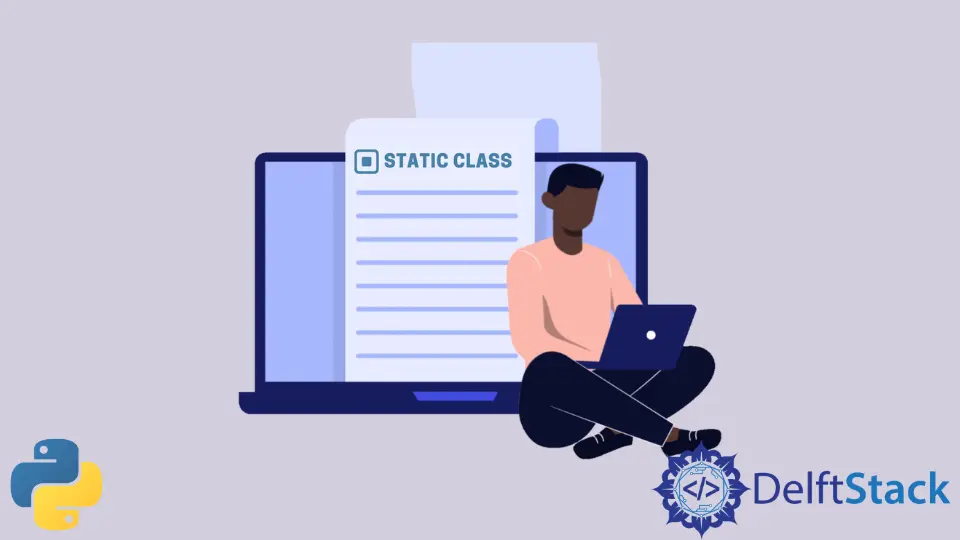
Static classes in Python are a powerful concept that can help you organize your code better and improve its readability. If you’re coming from other programming languages, you might be familiar with the idea of static members and methods. In Python, the term “static class” is somewhat different, as Python does not have static classes in the same way as languages like C# or Java. Instead, Python uses class methods and static methods to achieve similar functionality.
This tutorial will explore how to effectively use static methods in Python classes, providing you with clear examples and explanations along the way.
Understanding Static Methods in Python
Static methods in Python are defined using the @staticmethod
decorator. Unlike instance methods, which require an instance of the class to be called, static methods can be called on the class itself. This makes them ideal for utility functions that don’t need access to any instance-specific data.
Here’s a simple example to illustrate the concept:
class MathOperations:
@staticmethod
def add(x, y):
return x + y
result = MathOperations.add(5, 3)
Output:
8
In this example, we define a class MathOperations
with a static method add
. This method takes two parameters and returns their sum. Notice how we can call add
directly on the class without creating an instance. This is the essence of static methods: they belong to the class rather than any object instance.
Static methods are particularly useful for grouping related functions that don’t require access to instance or class attributes. They can help keep your code organized and maintainable, especially in larger applications.
Using Class Methods in Python
Another related concept in Python is the class method, defined using the @classmethod
decorator. Unlike static methods, class methods take a reference to the class (cls
) as their first parameter. This allows class methods to access class attributes and methods, which can be beneficial in certain scenarios.
Here’s an example to demonstrate class methods:
class Employee:
employee_count = 0
def __init__(self, name):
self.name = name
Employee.employee_count += 1
@classmethod
def get_employee_count(cls):
return cls.employee_count
emp1 = Employee("Alice")
emp2 = Employee("Bob")
count = Employee.get_employee_count()
Output:
2
In this code, we define an Employee
class that keeps track of the number of employees created. The get_employee_count
class method returns the current count of employees. This method can access the class variable employee_count
directly, demonstrating how class methods can be used to interact with class-level data.
Class methods are particularly useful for factory methods, which can return class instances based on different parameters. They enable you to maintain and modify class-level data in a more structured way.
When to Use Static and Class Methods
Deciding when to use static methods versus class methods can be crucial for code clarity and functionality. Static methods are best used when you need a utility function that does not interact with class or instance data. Conversely, class methods are ideal when you need to access or modify class-level data.
For example, if you are creating a utility for mathematical operations, static methods would be appropriate. However, if you are managing a collection of objects and need to keep track of their count or other class-level information, class methods would serve you better.
Here’s a practical example that combines both static and class methods:
class Circle:
pi = 3.14
@staticmethod
def area(radius):
return Circle.pi * (radius ** 2)
@classmethod
def from_diameter(cls, diameter):
radius = diameter / 2
return cls.area(radius)
circle_area = Circle.area(5)
circle_from_diameter = Circle.from_diameter(10)
Output:
78.5
In this example, we have a Circle
class with a static method area
that calculates the area based on the radius. We also have a class method from_diameter
that calculates the area using the diameter. This shows how both types of methods can coexist in a class, providing flexibility and functionality.
Conclusion
Static classes and methods in Python offer a unique way to organize and manage your code. By understanding the differences between static methods and class methods, you can make informed decisions about how to structure your classes for maximum efficiency and clarity. Whether you’re creating utility functions or managing class-level data, these concepts will enhance your programming toolkit. Embrace the power of static and class methods to write cleaner, more maintainable Python code.
FAQ
-
What is a static method in Python?
A static method is a method that belongs to a class rather than an instance of the class. It is defined using the@staticmethod
decorator and does not require access to instance or class data. -
How do class methods differ from static methods in Python?
Class methods are defined with the@classmethod
decorator and take a reference to the class as their first parameter. They can access and modify class-level data, while static methods cannot. -
When should I use static methods?
Use static methods for utility functions that do not require access to instance or class data. They help keep related functions organized within a class. -
Can static methods access instance variables?
No, static methods cannot access instance variables or class attributes because they do not have access to the instance (self
) or class (cls
) context. -
Can I call a static method from an instance of a class?
Yes, you can call a static method from an instance of a class, but it is more common to call it directly from the class itself.