How to Sort List of Dictionaries in Python
-
Use the
sorted()
Function to Sort a List of Dictionaries in Python -
Use the
sort()
Function to Sort a List of Dictionaries in Python
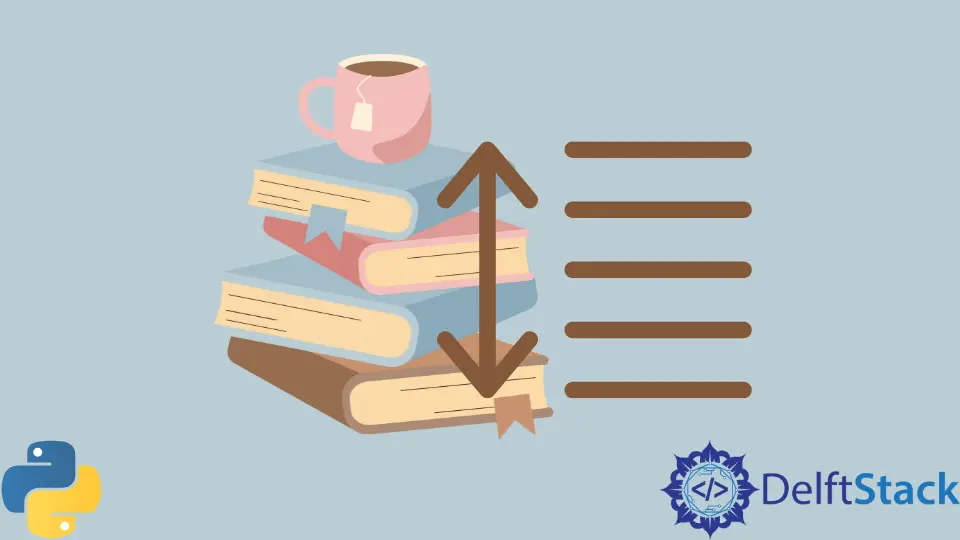
A list is a linear collection object in Python that can emulate arrays by storing every element at a particular index. Lists can also store other objects, like dictionaries that contain key-value pairs.
This tutorial illustrates how to sort a list of dictionaries in Python.
Use the sorted()
Function to Sort a List of Dictionaries in Python
This function takes an existing list and returns a sorted list. It can sort the list in ascending or descending order. We can also specify a key
parameter that provides a function to decide the order of the elements.
We can get an advantage by using the sorted()
function to sort the list of dictionaries. However, it is not simple to compare dictionary instances using the comparison operator; we need to use the key
parameter.
We can sort a list based on the key
’s value. Another condition for this is for that key
to be common in all the dictionaries in the list.
Let us now see an example of this.
lst = [{"n": 1}, {"n": 4}, {"n": 2}]
print(sorted(lst, key=lambda d: d["n"]))
Output:
[{'n': 1}, {'n': 2}, {'n': 4}]
The above example has dictionaries with the same key, n
that we sort based on the value of this key. We assign a lambda
function with the key
parameter of the sorted()
function.
This one-line function tells the parameter to consider the value associated with this key
to sort them. We can also use the operator.itemgetter()
function in place of the lambda
function.
We can use this function to fetch the value from the dictionary and use it for comparison. See the code below.
import operator
lst = [{"n": 1}, {"n": 4}, {"n": 2}]
print(sorted(lst, key=operator.itemgetter("n")))
Output:
[{'n': 1}, {'n': 2}, {'n': 4}]
We can also use the reverse
parameter and set it to True
to sort the list in descending order. For example, see the following code fence.
import operator
lst = [{"n": 1}, {"n": 4}, {"n": 2}]
print(sorted(lst, key=operator.itemgetter("n"), reverse=True))
Output:
[{'n': 4}, {'n': 2}, {'n': 1}]
The above example shows that the list has been sorted in descending order.
Use the sort()
Function to Sort a List of Dictionaries in Python
The sort()
function works similarly to the sorted
method for sorting lists. The main difference is that the sort()
function alters the order of the original list, and the latter creates a new list.
We can use the sort()
method in the same way we used the sorted
function, with the operator.itemgetter()
and lambda
functions.
We will use the key
parameter as we did in the previous examples. We can also use the reverse
parameter to sort the list in descending order.
See the code below.
import operator
lst1 = [{"n": 1}, {"n": 4}, {"n": 2}]
lst2 = [{"n": 5}, {"n": 1}, {"n": 3}]
lst1.sort(key=lambda d: d["n"])
lst2.sort(key=operator.itemgetter("n"), reverse=True)
print(lst1, lst2)
Output:
[{'n': 1}, {'n': 2}, {'n': 4}] [{'n': 5}, {'n': 3}, {'n': 1}]
The above example shows that the original lists have been modified and sorted according to the required key
.
The first list uses the lambda
function. The second one uses the operator.itemgetter
method and sorts the list in descending order with the reverse
parameter.
So, we have two ways to sort a list of dictionaries in Python. First, it used the sorted
and sort
functions. To sort a list of dictionaries, we use the value from a common key
in all the dictionaries.
We create a function that returns this value and specifies it with the key
parameter in both functions. We discussed the lambda
and operator.itemgetter
methods for this.
To get the result in descending order, we can use the reverse
parameter and set it to True
. Bravo! You have successfully learned how to sort a list of dictionaries in Python.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python