How to Reverse a String in Python
- Reverse a String in Python Using the Slicing Method
- Reverse a String in Python Using the Loop
-
Reverse a String in Python Using the
reversed()
Function - Reverse a String in Python Using the Recursive Method
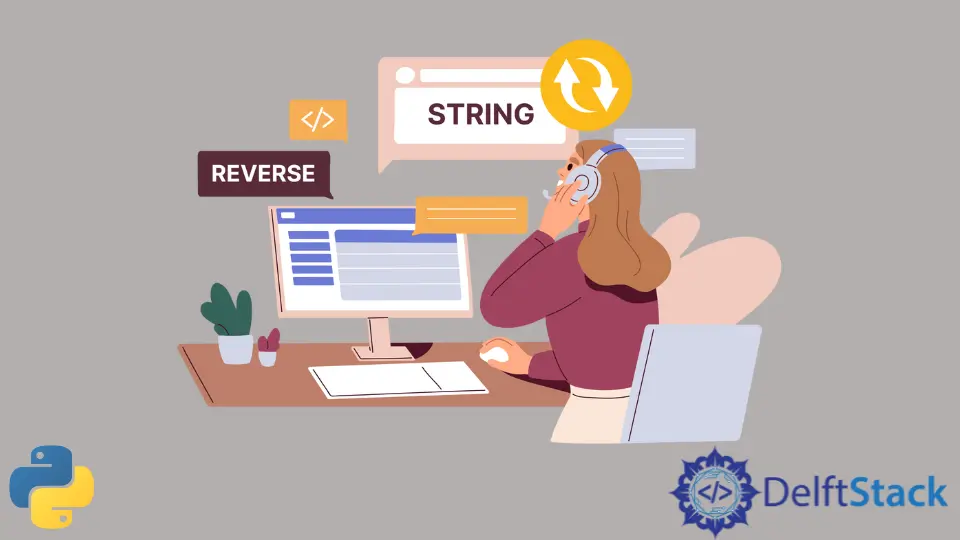
This article will introduce some methods to reverse a string in Python.
Reverse a String in Python Using the Slicing Method
This method uses the slicing operator :
to reverse the string. The start index of the slicing is the last element’s index, and the end index is the first element’s index (it could also be ignored in this case). The step is -1
, indicating that the slicing steps backward at the step of 1.
The complete example code is given below:
str = "LearnPython"
length_str = len(str)
sliced_str = str[length_str::-1]
print("The sliced string is:", sliced_str)
Output:
The sliced string is: nohtyPnraeL
Reverse a String in Python Using the Loop
In this method, first, we create an empty string to store the reversed string. The length of the given string is saved in the i
variable as an index. The while
loop continues until the index is greater than 0.
The complete example code is given below.
str = "LearnPython"
string_reversed = []
i = len(str)
while i > 0:
string_reversed += str[i - 1]
i = i - 1 # decrement index
print("The Reversed String is", string_reversed)
The string_reversed += str[i-1]
statement save the value of str[i-1]
in the reversed string. i=i-1
decrements the index until it reaches the start of the string.
Output:
The Reversed String is ['n', 'o', 'h', 't', 'y', 'P', 'n', 'r', 'a', 'e', 'L']
Reverse a String in Python Using the reversed()
Function
The reversed()
function gives the reversed iterator of the given string. Its elements are joined by the empty string using the join()
method.
The complete example code is given below.
str = "LearnPython"
reversed_string = "".join(reversed(str))
print("The Reversed String is", reversed_string)
The join()
method merges all of the characters resulting from the reversed iteration into a new string.
Output:
The Reversed String is nohtyPnraeL
Reverse a String in Python Using the Recursive Method
We can use a recursive function to reverse the string. The base condition is that the string is returned if the string length is equal to 0. If not equal to 0, the reverse function is called recursively, except for the first character, to slice the string section and concatenate the first character to the end of the sliced string.
The complete example code is given below.
def reverse_string(string):
if len(string) == 0:
return string
else:
return reverse_string(string[1:]) + string[0]
string = "LearnPython"
print("The original string is : ", end="")
print(string)
print("The reversed string is : ", end="")
print(reverse_string(string))
Output:
The original string is : LearnPython
The reversed string(using loops) is : nohtyPnraeL