Reverter uma string em Python
- Reverter uma string em Python usando o método de fatiar
- Reverter uma string em Python usando o loop
-
Reverter uma string em Python usando a função
reversed()
- Reverter uma string em Python usando o método recursivo
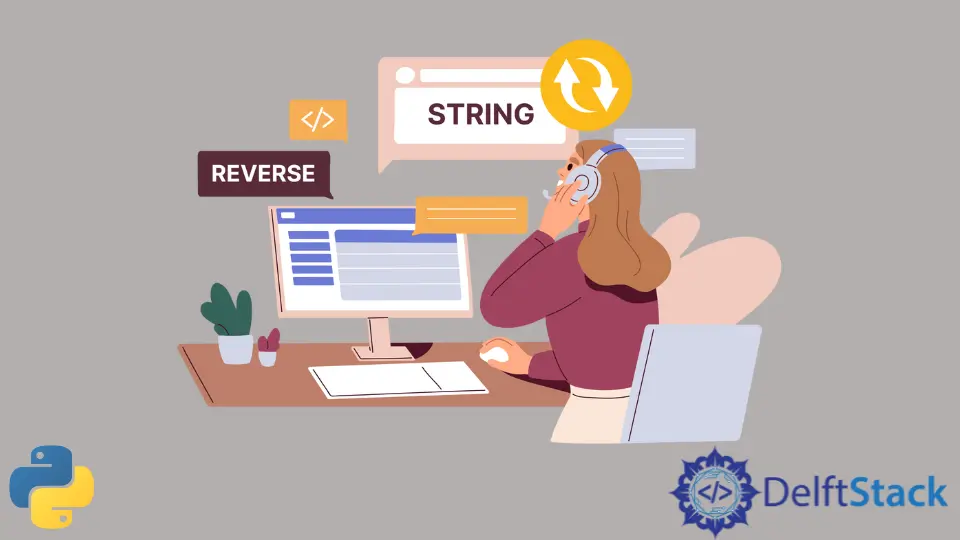
Este artigo apresentará alguns métodos para reverter uma string em Python.
Reverter uma string em Python usando o método de fatiar
Este método usa o operador de fatiamento :
para inverter a string. O índice inicial do fatiamento é o índice do último elemento e o índice final é o índice do primeiro elemento (também pode ser ignorado neste caso). O passo é -1
, indicando que o corte retrocede no passo de 1.
O exemplo de código completo é fornecido abaixo:
str = "LearnPython"
length_str = len(str)
sliced_str = str[length_str::-1]
print("The sliced string is:", sliced_str)
Resultado:
The sliced string is: nohtyPnraeL
Reverter uma string em Python usando o loop
Neste método, primeiro, criamos uma string vazia para armazenar a string invertida. O comprimento da string fornecida é salvo na variável i
como um índice. O loop while
continua até que o índice seja maior que 0.
O código de exemplo completo é fornecido abaixo.
str = "LearnPython"
string_reversed = []
i = len(str)
while i > 0:
string_reversed += str[i - 1]
i = i - 1 # decrement index
print("The Reversed String is", string_reversed)
A instrução string_reversed += str[i-1]
salva o valor de str[i-1]
na string invertida. i=i-1
diminui o índice até atingir o início da string.
Resultado:
The Reversed String is ['n', 'o', 'h', 't', 'y', 'P', 'n', 'r', 'a', 'e', 'L']
Reverter uma string em Python usando a função reversed()
A função reversed()
fornece o iterador reverso da string dada. Seus elementos são unidos por uma string vazia usando o método join()
.
O código de exemplo completo é fornecido abaixo.
str = "LearnPython"
reversed_string = "".join(reversed(str))
print("The Reversed String is", reversed_string)
O método join()
mescla todos os caracteres resultantes da iteração reversa em uma nova string.
Resultado:
The Reversed String is nohtyPnraeL
Reverter uma string em Python usando o método recursivo
Podemos usar uma função recursiva para reverter a string. A condição básica é que a string seja retornada se o comprimento da string for igual a 0. Se não for igual a 0, a função reversa é chamada recursivamente, exceto para o primeiro caractere, para cortar a seção da string e concatenar o primeiro caractere ao final da corda fatiada.
O código de exemplo completo é fornecido abaixo.
def reverse_string(string):
if len(string) == 0:
return string
else:
return reverse_string(string[1:]) + string[0]
string = "LearnPython"
print("The original string is : ", end="")
print(string)
print("The reversed string is : ", end="")
print(reverse_string(string))
Resultado:
The original string is : LearnPython
The reversed string(using loops) is : nohtyPnraeL