How to Return Multiple Values From a Function in Python
- Use a Tuple to Return Multiple Values From a Function in Python
- Use a List to Return Multiple Values From a Function in Python
- Use a Dictionary to Return Multiple Values From a Function in Python
- Use a Class to Return Multiple Values From a Function in Python
-
Use a
dataclass
to Return Multiple Values From a Function in Python
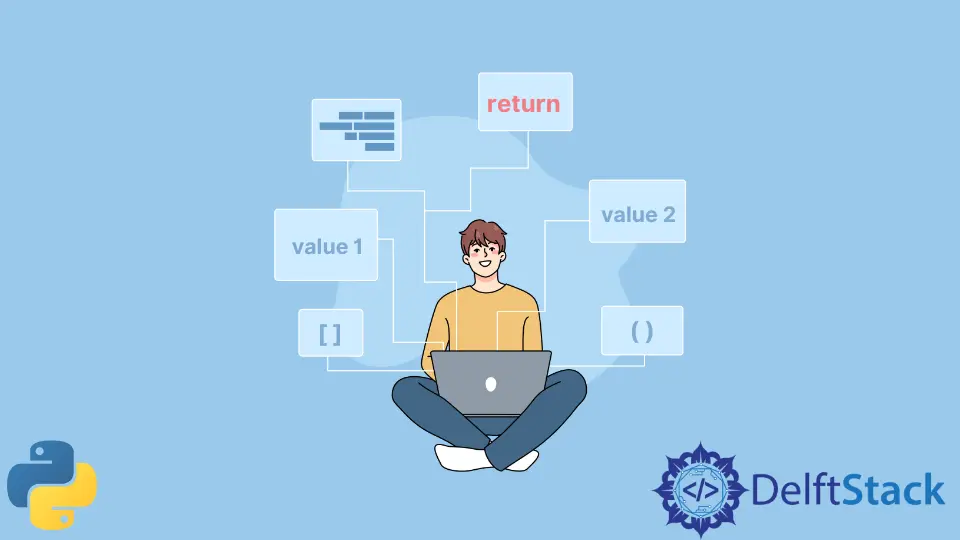
Functions are an essential part of any programming language. A function is a block of code that can be called to perform a specific operation in programming. Usually, a function is used to return a value. This value can be a number, a string, or any other datatype.
In this tutorial, we will discuss different methods to create a function that returns multiple values. We will return different data objects like a list, a dictionary, and other objects from a user-defined function to achieve this.
Use a Tuple to Return Multiple Values From a Function in Python
If we return values from a function separated by a comma, they are considered a tuple. Tuples are usually enclosed in parenthesis. In the code below, we will return a tuple from a Python function.
def return_multi(a):
b = a + 1
c = a + 2
return b, c
x = return_multi(5)
print(x, type(x))
Output:
(6, 7) <class 'tuple'>
Use a List to Return Multiple Values From a Function in Python
Python lists are used to store different items under a common name and at specific positions. Functions can also return multiple values in a list, as shown below.
def return_multi(a):
b = a + 1
c = a + 2
return [b, c]
x = return_multi(5)
print(x, type(x))
Output:
[6, 7] <class 'list'>
Use a Dictionary to Return Multiple Values From a Function in Python
Dictionaries are used to store key-value pairs in Python. We can have the final output in a much more organized format by returning a dictionary from a function with keys assigned to different values. See the following example.
def return_multi(a):
b = a + 1
c = a + 2
return {"b": b, "c": c}
x = return_multi(5)
print(x, type(x))
Output:
{'b': 6, 'c': 7} <class 'dict'>
Use a Class to Return Multiple Values From a Function in Python
Classes contain different data members and functions and allow us to create objects to access these members. We can return an object of such user-defined classes based on the class structure and its data members. For example:
class return_values:
def __init__(self, a, b):
self.a = a
self.b = b
def return_multi(a):
b = a + 1
c = a + 2
t = return_values(b, c)
return t
x = return_multi(5)
print(x.a, x.b, type(x))
Output:
6 7 <class '__main__.return_values'>
Use a dataclass
to Return Multiple Values From a Function in Python
A dataclass
is a new interesting feature added in Python v3.7 and up. They are similar to traditional classes but mainly used to store data and all their basic functionalities are already implemented. The @dataclass
decorator and the dataclass
module are used to create such objects. In the code below, we return a dataclass
from a function.
from dataclasses import dataclass
@dataclass
class return_values:
a: int
b: int
def return_multi(a):
b = a + 1
c = a + 2
t = return_values(b, c)
return t
x = return_multi(5)
print(x.a, x.b, type(x))
Output:
6 7 <class '__main__.return_values'>
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn