How to Remove Substring From String in Python
-
Use
str.replace()
Method to Replace Substring From String in Python 3.x -
Use
string.replace()
Method to Replace Substring From String in Python 2.x -
Use
str.removesuffix()
to Remove Suffix From String
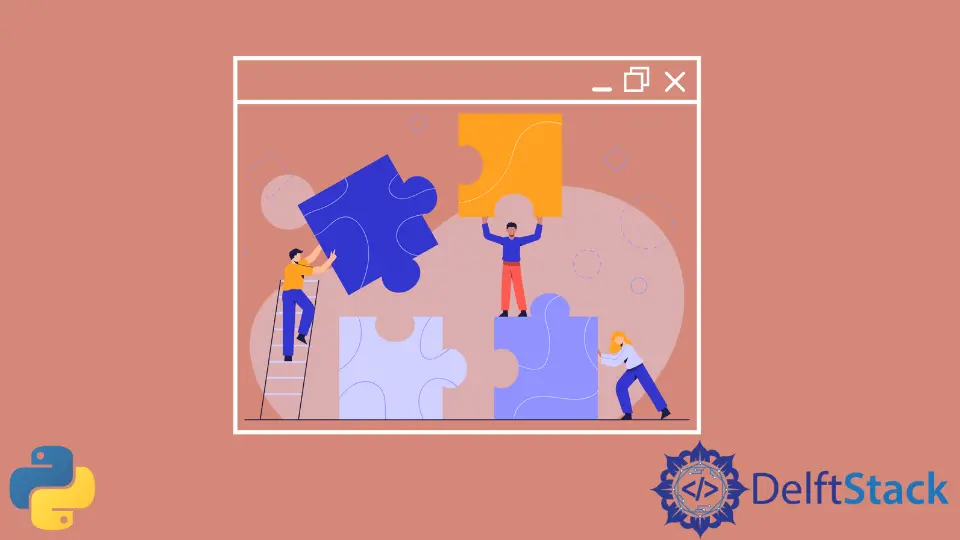
This tutorial describes how to remove a substring from a string in Python. It will tell us that strings can not be just removed but just replaced. The tutorial also lists some example codes to clarify the concepts as the method has changed from previous Python versions.
Use str.replace()
Method to Replace Substring From String in Python 3.x
There are many built-in methods available for strings. Actually, strings are immutable in Python. You can usestr.replace()
method to create a new string. str.replace(oldvalue, newvalue, count)
returns a copy of string whose oldvalue
is replaced with newvalue
. count
tells how many occurrences the replacement will be performed.
list_str = {"Abc.ex", "Bcd.ex", "cde.ex", "def.jpg", "efg.jpg"}
new_set = {x.replace(".ex", "").replace(".jpg", "") for x in list_str}
print(new_set)
Output:
{'Bcd', 'Abc', 'cde', 'def', 'efg'}
Use string.replace()
Method to Replace Substring From String in Python 2.x
If you are using Python 2.x, you can use string.replace()
method to replace a substring. This method takes old value
, new value
, and count
as its parameters. new value
is required to replace the old value
and count
is a number specifying how many occurrences of the old value you want to replace. Default is all occurrences.
An example code for this method is given below:
text = "Hello World!"
x = text.replace("l", "k", 1)
print(x)
Output:
Heklo World!
Use str.removesuffix()
to Remove Suffix From String
If you are using Python 3.9, you could remove suffix using str.removesuffix('suffix')
.
If the string ends with a suffix string and the suffix is non-empty, return the string with suffix removed. Otherwise, the original string will be returned.
The basis example for str.removesuffix()
is given below:
text = "Quickly"
print(text.removesuffix("ly"))
print(text.removesuffix("World"))
Output:
Quick
Quickly
Syed Moiz is an experienced and versatile technical content creator. He is a computer scientist by profession. Having a sound grip on technical areas of programming languages, he is actively contributing to solving programming problems and training fledglings.
LinkedIn