How to Remove Non-Alphanumeric Characters From Python String
-
Use the
isalnum()
Method to Remove All Non-Alphanumeric Characters in Python String -
Use the
filter()
Function to Remove All Non-Alphanumeric Characters in Python String -
Use Regular Expressions (
re
Module) to Remove All Non-Alphanumeric Characters in Python String - Use ASCII Values to Remove All Non-Alphanumeric Characters in Python String
- Conclusion
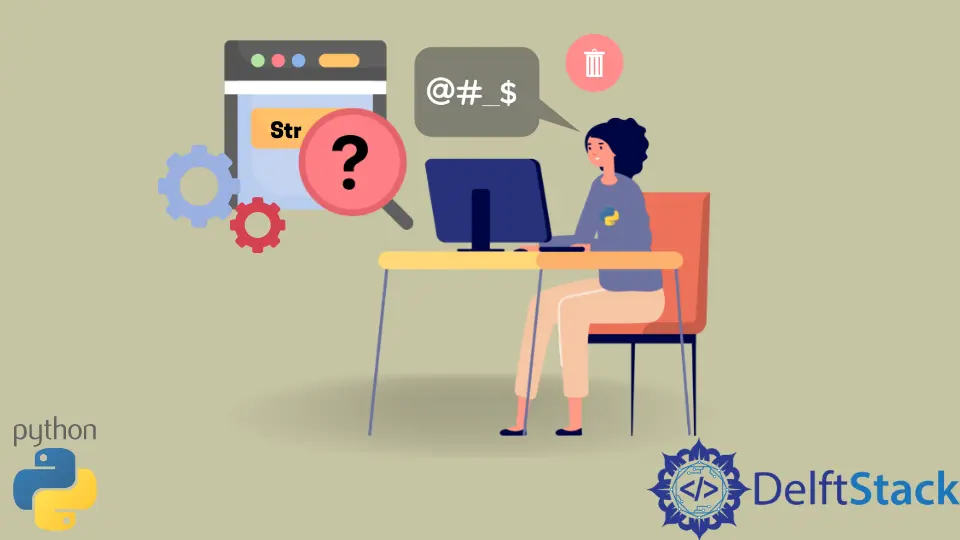
In many text processing tasks, it’s common to need a cleaned version of a string, with only alphanumeric characters remaining. Python offers several methods to accomplish this task efficiently.
Alphanumeric characters contain the blend of the 26 characters of the letter set and the numbers 0 to 9. Non-alphanumeric characters include characters that are not letters or digits, like +
and @
.
In this tutorial, we will discuss how to remove all non-alphanumeric characters from a string in Python.
Use the isalnum()
Method to Remove All Non-Alphanumeric Characters in Python String
Python’s isalnum()
method checks if all characters in a given string are alphanumeric (letters and numbers) and returns True
if they are. By using list comprehension and join()
, we can efficiently remove non-alphanumeric characters.
This method is particularly useful when you need to filter out non-alphanumeric characters from a string. It provides a quick and efficient way to clean and validate input data, making it a valuable tool in various applications, such as data preprocessing and form validation.
Syntax:
str.isalnum()
str
: The string to be checked for alphanumeric characters.
Return Value:
True
: If all characters in the string are alphanumeric.False
: If the string contains any non-alphanumeric characters.
We can use the isalnum()
method to check whether a given character or string is alphanumeric or not. We can compare each character individually from a string, and if it is alphanumeric, then we combine it using the join()
function.
For example:
string_value = "alphanumeric@123__"
s = "".join(ch for ch in string_value if ch.isalnum())
print(s)
This code takes a string (string_value
) that contains a mix of alphanumeric and non-alphanumeric characters. It then iterates through each character, checks if it’s alphanumeric, and if so, adds it to the s
variable.
Finally, it prints the cleaned string s
.
Output:
alphanumeric123
Use the filter()
Function to Remove All Non-Alphanumeric Characters in Python String
The filter()
function is a built-in Python function that enables precise filtering of elements from an iterable (such as a list or string) based on a given condition.
It takes two arguments: a function and an iterable. The function is applied to each element in the iterable, and only elements for which the function returns True
are retained.
Syntax:
filter(function, iterable)
function
: A function that tests each element of an iterable.iterable
: A sequence or collection of elements.
Return Value:
- A filter object that contains the elements for which the function returns
True
.
For this example, the string is our object, and we will use the isalnum()
function, which checks whether a given string contains alphanumeric characters or not by checking each character. The join()
function combines all the characters to return a string.
For example:
string_value = "alphanumeric@123__"
s = "".join(filter(str.isalnum, string_value))
print(s)
This code takes a string (string_value
) that contains a mix of alphanumeric and non-alphanumeric characters. It then applies a filtering operation using filter()
along with the str.isalnum
function, which checks for alphanumeric characters.
The result is a string s
that contains only the alphanumeric characters from the original string. Finally, it prints the cleaned string s
.
Output:
alphanumeric123
Use Regular Expressions (re
Module) to Remove All Non-Alphanumeric Characters in Python String
A regular expression is an exceptional grouping of characters that helps you match different strings or sets of strings, utilizing a specific syntax in a pattern. To use regular expressions, we import the re
module.
We can use the sub()
function from this module to replace all the string that matches a non-alphanumeric character with an empty character.
The re.sub()
function in Python is used to perform regular expression-based substitution in a string. It has the following syntax:
re.sub(pattern, repl, string, count=0, flags=0)
Here’s what each parameter represents:
pattern
: This is the regular expression pattern to be matched in the input string.repl
: This is the replacement string that will replace the occurrences of the pattern.string
: This is the input string on which the operation will be performed.count
(optional): This parameter specifies the maximum number of substitutions to be made. By default, all occurrences are replaced.flags
(optional): This parameter allows you to provide additional flags to control the behavior of the regular expression. Flags can be used to modify how the pattern is interpreted.
To remove non-alphanumeric characters from a string using regular expressions, we’ll construct a pattern that matches everything except letters (both uppercase and lowercase) and digits.
For example:
import re
string_value = "alphanumeric@123__"
s = re.sub(r"[^a-zA-Z0-9]", "", string_value)
print(s)
In this example, the input string contains a mix of alphanumeric and non-alphanumeric characters. After applying the regular expression pattern r"[^a-zA-Z0-9]"
, which matches non-alphanumeric characters, and replacing them with an empty string ""
, the code prints the modified string.
The output demonstrates that the non-alphanumeric characters have been successfully removed, leaving only the alphanumeric characters in the result.
Output:
alphanumeric123
Alternatively, we can also use the following pattern, r"[\W_]+"
.
import re
string_value = "alphanumeric@123__"
s = re.sub(r"[\W_]+", "", string_value)
print(s)
This code provides an alternative approach using a slightly different regular expression pattern to achieve the same result.
Output:
alphanumeric123
Using Regular Expression Compilation
This is similar to the above method but with the regular expression pattern precompiled for efficiency in case of repeated use.
Example Code:
import re
non_alphanumeric_pattern = re.compile(r'[^a-zA-Z0-9]')
def remove_non_alphanumeric_compiled(input_string):
return non_alphanumeric_pattern.sub('', input_string)
s = remove_non_alphanumeric_compiled("alphanumeric@123__")
print(s)
Explanation:
First, import re
imports the regular expressions module.
Then, the re.compile(r'[^a-zA-Z0-9]')
compiles the regular expression pattern, creating a pattern object. This step is performed once, and the object can be reused for multiple substitutions.
The non_alphanumeric_pattern.sub('', input_string)
uses the sub()
method of the precompiled pattern to perform the substitution. Next, return
is used to return the modified string.
The s = remove_non_alphanumeric_compiled("alphanumeric@123__")
line applies the function to the input string "alphanumeric@123__"
. Lastly, print(s)
prints the modified string.
Output:
alphanumeric123
Use ASCII Values to Remove All Non-Alphanumeric Characters in Python String
American Standard Code for Information Interchange or ASCII is a character encoding standard that assigns unique numerical values to letters, digits, and symbols. Each character is represented by a unique integer value, making it possible to perform operations based on these values.
In Python, you can retrieve the ASCII value of a character using the ord()
function and convert an ASCII value back to its corresponding character using the chr()
function.
The concept behind using ASCII values to filter out non-alphanumeric characters involves iterating through each character in a string, checking its ASCII value, and retaining only those within specific ranges corresponding to letters (both uppercase and lowercase) and digits.
Example Code:
def remove_non_alphanumeric_ascii(input_string):
return ''.join(char for char in input_string if 65 <= ord(char) <= 90 or 97 <= ord(char) <= 122 or 48 <= ord(char) <= 57)
cleaned_string = remove_non_alphanumeric_ascii("alphanumeric@123__")
print(cleaned_string)
Explanation:
First, the def remove_non_alphanumeric_ascii(input_string)
line defines a function remove_non_alphanumeric_ascii
that takes a string (input_string
) as input.
The return
function will return a modified string after removing non-alphanumeric characters. Next, the for char in input_string
initiates a loop that iterates through each character in the input string.
Then, the if 65 <= ord(char) <= 90 or 97 <= ord(char) <= 122 or 48 <= ord(char) <= 57
condition checks if the ASCII value of the character falls within specific ranges. The 65 <= ord(char) <= 90
checks for uppercase letters (A-Z), the 97 <= ord(char) <= 122
checks for lowercase letters (a-z), and the 48 <= ord(char) <= 57
checks for digits (0-9).
The ''.join(...)
joins the characters that meet the conditions into a single string. And, the cleaned_string = remove_non_alphanumeric_ascii("alphanumeric@123__")
line applies the function to the input string "alphanumeric@123__"
.
Lastly, the print(cleaned_string)
prints the modified string.
Output:
alphanumeric123
In this example, the function remove_non_alphanumeric_ascii
filters out non-alphanumeric characters using ASCII values. The conditions within the loop ensure that only letters and digits are retained.
The final result is the cleaned string "alphanumeric123"
.
Conclusion
This tutorial explored multiple methods for removing non-alphanumeric characters from strings in Python. We discussed four methods in detail and had examples to further understand their advantages and disadvantages.
First, we discussed the isalnum()
method that utilizes isalnum()
to efficiently remove non-alphanumeric characters. It is suitable for quick data cleaning and validation tasks.
Next, we got into detail about the filter()
function that employs filter()
in combination with str.isalnum
for elegant character filtering. This is valuable for scenarios like user input validation or data preparation.
Then, we studied the Regular Expressions (re
Module) leverages powerful pattern-based substitutions for versatile text manipulation. It allows for complex pattern matching and replacement.
Lastly, we analyzed the ASCII values method that uses ASCII values to selectively retain alphanumeric characters. It offers fine-grained control over character inclusion.
These methods cater to various needs, from quick data cleaning to complex pattern-based processing. Python provides a rich set of tools for efficient text manipulation, enabling developers to choose the most appropriate approach for their specific tasks.