How to Remove Multiple Elements From a List in Python
-
Remove Multiple Elements From a List Using
if...else
Control Statements - Deleting Multiple Elements From a List Using List Comprehension Method in Python
- Remove Multiple Elements From a List Using List Slicing
-
Remove Multiple Elements From a List Using
for
Loop
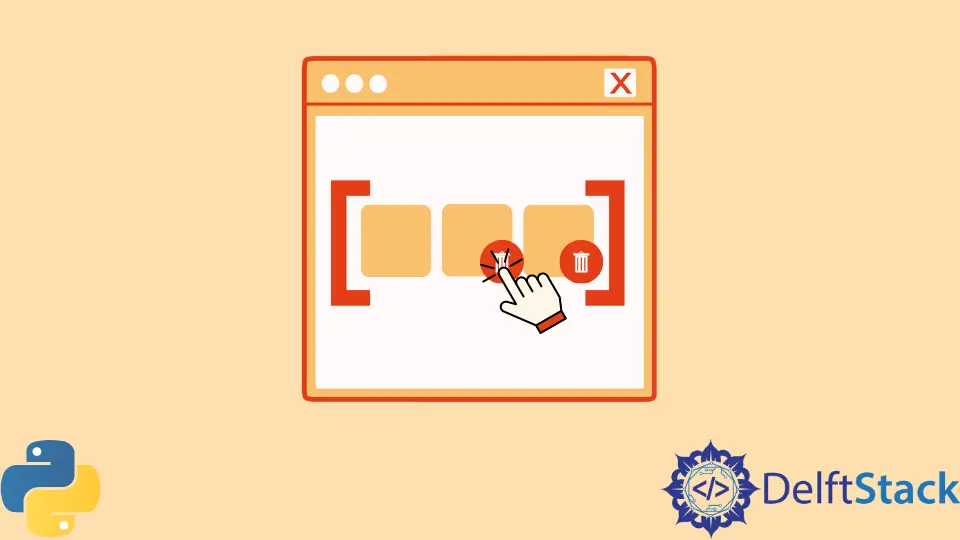
To remove multiple values from a Python list, we can either remove the actual values of the list or the indexes of values to be removed from the list. We can use if...else
control statements, list comprehension, list slicing, and for
loops to remove multiple elements from a list in Python.
Remove Multiple Elements From a List Using if...else
Control Statements
We can use if...else
control statements to remove multiple values from a list in Python if the values satisfy a certain condition. The elements can also be removed if their indexes satisfy a certain condition.
list1 = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 20]
print("Original list : ", list1)
for ele in list1:
if (ele % 2) != 0:
list1.remove(ele)
print("List after deleting all values which are odd : ", list1)
Output:
Original list : [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 20]
List after removing all the odd values : [2, 4, 6, 8, 10, 20]
Here, we have a list of integers and need to remove the elements with the odd value from the list. In this case, we loop through all the list l1
elements and remove an element from the list using the remove()
method if the element has an odd value.
Deleting Multiple Elements From a List Using List Comprehension Method in Python
In Python, list comprehension refers to the process of generating a new list from an existing list. The list comprehension can also be used to remove multiple elements from the list. We can create a new list by removing the values to be removed from the original list.
l1 = [2, 3, 4, 5, 6, 7, 8, 9, 10, 20, 80, 99]
l2 = [x for x in l1 if x % 2 == 0]
print("Original list : ", l1)
print("List after deleting all the odd values present in List : ", l2)
Output:
Original List : [2, 3, 4, 5, 6, 7, 8, 9, 10, 20, 80, 99]
List after deleting all the odd values present in List : [2, 4, 6, 8, 10, 20, 80]
Here, we create list l2
from the list l1
, which only contains all the even values in the l1
. In other words, we can say that we created a new list l2
from the existing list l1
by removing all the odd values in the list l1
.
Remove Multiple Elements From a List Using List Slicing
We can also remove multiple elements from a list using the List slicing method. Here we can put the range of the element from starting to last index of the element that we want to remove from the list in the del
method.
Instead of using a single index in the del
method, we use a range of values from starting to the last index of the elements that we want to remove from the list. It will remove the contiguous elements from the list. We must note that the index of values starts from 0
in Python for lists.
l1 = [2, 3, 4, 5, 6, 7, 8, 9, 10, 20, 80, 99]
print("Original list : ", l1)
del l1[2:5]
print("List after removing values at index 2, 3 and 4 : ", l1)
Output:
Original List : [2, 3, 4, 5, 6, 7, 8, 9, 10, 20, 80, 99]
List after removing values at index 2, 3 and 4 : [1, 2, 6, 7, 8, 9, 10, 20, 80, 99]
It removes the values at the indexes 2
, 3
, and 4
from the list l1
using the del
method.
One important thing to be noted here is the first index 2
is inclusive i.e. element at index 2
in the list 3
is removed while the last index is an exclusive value, meaning element at index 5
in the list 6
is not removed.
Remove Multiple Elements From a List Using for
Loop
We can also use the for
loops to remove multiple elements from a list.
To apply this method, we have to store the indexes of the elements that we want to delete from the list. But randomly removing the element will result in changing the values of the indexes. Our smart strategy will be to delete the element whose position in the list is greater. So the value of the element at other indexes will not change. Now, we will sort the list in descending order to delete the element at a higher index will first. The code for this approach is shown below.
l1 = [2, 3, 4, 5, 6, 7, 8, 9, 10, 20, 80, 99]
print("Original list : ", l1)
indexes_to_be_removed = [0, 2, 5]
for idx in sorted(indexes_to_be_removed, reverse=True):
del l1[idx]
print("List after removing values at index 0, 2 and 5: ", l1)
Output:
Original List : [2, 3, 4, 5, 6, 7, 8, 9, 10, 20, 80, 99]
List after removing values at index 0, 2 and 5: [3, 5, 6, 8, 9, 10, 20, 80, 99]
It removes the values at the indexes 0
, 2
, and 5
from the list l1
using the del
method.
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python