How to Read gzip File in Python
-
the
gzip
Module in Python - Importance of Compressing a File
-
Compress a File With
gzip
in Python -
Read From a
gzip
File in Python -
Decompress
gzip
File in Python
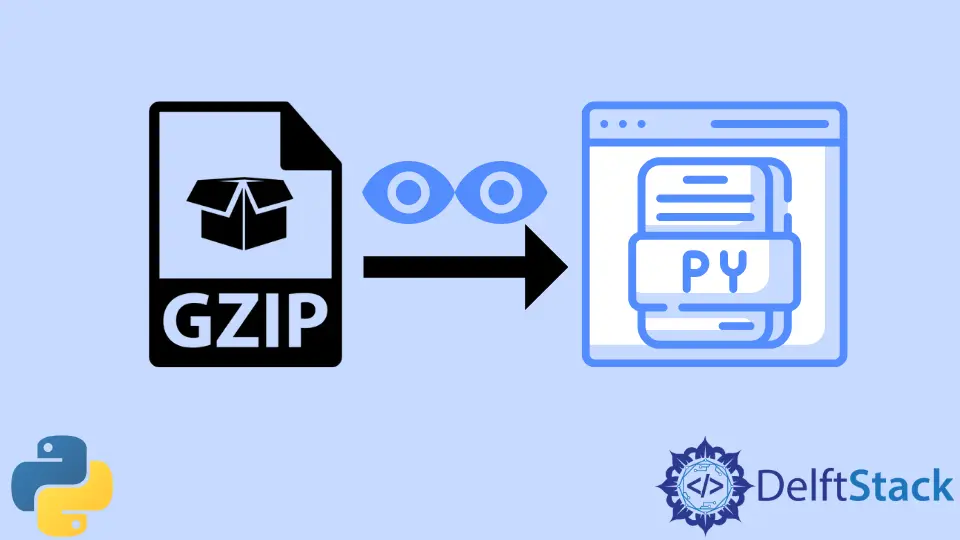
This tutorial discusses the importance of compressing a file and illustrates how to compress and decompress a file using Python’s gzip
module.
the gzip
Module in Python
This module provides a straightforward interface for compressing and decompressing files like the GNU programmers gzip
and gunzip
. The gzip
module offers the GzipFile
class as well as the open()
, compress()
, and decompress()
convenience methods.
The gzip
module reads and writes files in the gzip
format, compressing or decompressing the contents to make them seem like conventional file objects.
The’ gzip’ is one of the most widely used file compression/decompression formats. Text files can significantly save storage space by using gzip
compression. When working with large data files, it is common practice to gzip
or compress large text files to reduce file size.
Unzipping a compressed gzip
file and editing a much larger unzipped file line by line is fundamental to working with compressed files. However, that cannot possibly be the most excellent option.
Importance of Compressing a File
Reduced storage hardware, data transfer times, and communication bandwidth are just a few benefits of compression. In addition, this might result in significant financial savings.
Compressed files need much less storage space than uncompressed ones, which significantly reduces the cost of storage. Additionally, a compressed file transfers more quickly while utilizing less network capacity.
This can save expenditures while boosting productivity. Data compression’s main drawback is that it uses more computing power to compress the necessary data.
As a result, compression providers place a high value on maximizing speed and resource efficiency to lessen the effect of intensive compression activities. As a result, costs can be reduced while productivity rises.
Compress a File With gzip
in Python
In the below example opening a text file in wb
mode means that the file is open to writing in a binary mode. So here, the file test.txt
is changed into test.txt.gz
.
Example Code:
import gzip
f_in = open("test.txt", "rb")
f_out = gzip.open("test.txt.gz", "wb")
f_out.writelines(f_in)
f_out.close()
f_in.close()
print("The file is compressed.")
Output:
The file is compressed.
Data Addition While Using the gzip
Module
In the code below, it is shown that we can add any data we want to add in the existing file test.txt.gz
, but we can only add data in binary mode, so we have used b
at the start of the text, and a message is displayed successfully added
.
Example Code:
import gzip
data = b"Information included in this file."
with gzip.open("test.txt.gz", "wb") as f:
f.write(data)
print("successfully added")
Output:
successfully added
Read From a gzip
File in Python
We can read the file in the following code as we have added text in binary format. Then, it displays that text in the output as shown. The binary file is opened for reading in rb
mode, so we have used rb
for the opening file.
Example Code:
import gzip
with gzip.open("test.txt.gz", "rb") as f:
for line in f:
print(line)
Output:
b'Information included in this file.'
Decompress gzip
File in Python
In the following example, we’ll open the unzipped file first, then the zipped file, and then use shutil
to copy the objects from the unzipped file to the zipped file.
Example Code:
import gzip
import shutil
with gzip.open("test.txt.gz", "rb") as f_in:
with open("test.txt", "wb") as f_out:
shutil.copyfileobj(f_in, f_out)
print("The file is Decompressed.")
Output:
The file is Decompressed.
Here are a few essential things that you must remember. First, to create a gzip
file, we have to convert a text file into a gz
file; basically, you must compress the file.
You can write the file in binary mode only wb
, read the file in binary mode rb
, and decompress the compressed file.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn