How to Read Binary File in Python
-
Read a Binary File With
open()
Function in Python -
Read a Binary File With
pathlib.Path
in Python -
Read a Binary File With
numpy.fromfile()
Function in Python
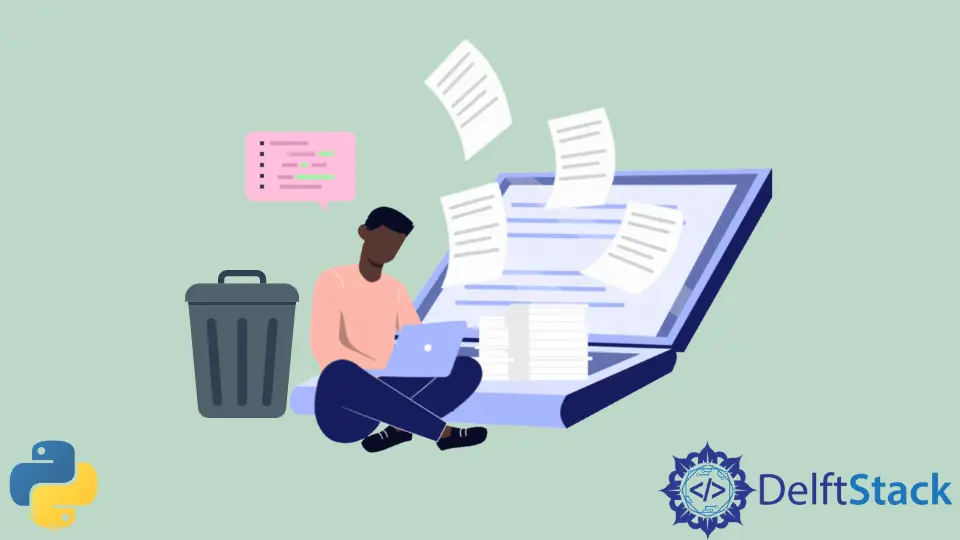
The program or the internal processor interprets a binary file. It contains the bytes as the content. When we read a binary file, an object of type bytes
is returned.
Read a Binary File With open()
Function in Python
In Python, we have the open()
function used to create a file object by passing its path to the function and opening a file in a specific mode, read mode by default. When we open binary files, we have to specify the b
parameter when opening such files in reading, writing, or appending mode. In this tutorial, we will deal with the binary reading mode - rb
.
In the code below, we will read a binary file and print a character from the file:
with open("sample.bin", "rb") as f:
data = f.read()
print(data[2])
Output:
83
If we print individual characters, then we can view the integers.
Python has a package called struct
, which has many methods and can be used to handle binary data stored in files, databases, and other resources.
The struct.unpack()
is used to read packed data in a specified format layout. Such layout, which is used while packing and unpacking data, is specified using format characters. These format characters, along with their size, are shown below:
Note that the struct.unpack()
function always returns a tuple.
import struct
with open("sample.bin", "rb") as f:
data = f.read()
unpack_result = struct.unpack("hhl", data[0:8])
print(unpack_result)
Output:
(1280, 27731, 7037801)
Here, hhl
indicates short, short, and long int as the data format layout, as we can see in the output. That is why the buffer for unpacking is only 8 bytes since the format layout’s size is 8(2+2+4).
Read a Binary File With pathlib.Path
in Python
We can also use the read_bytes()
method from the Path
class in the pathlib
library to read a file in bytes mode and then interpret data using the struct.unpack()
function as shown earlier:
from pathlib import Path
import struct
data = Path("sample.bin").read_bytes()
multiple = struct.unpack("ii", data[:8])
print(multiple)
Output:
(1817380096, 7037801)
Read a Binary File With numpy.fromfile()
Function in Python
Another interesting approach is provided in the NumPy
module. Using the fromfile()
function in this module, we can read binary data from files after specifying the format data using the dtype()
function. This is considered to be a speedy method. The following code shows how to implement this:
import numpy as np
with open("sample.bin") as f:
rectype = np.dtype(np.int32)
bdata = np.fromfile(f, dtype=rectype)
print(bdata)
Output:
[1817380096 7037801]
Here we specify the format type as integer-32 bit and extract the data using the fromfile()
function.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn