Difference between write and writelines in Python
-
Use the
write()
andwritelines()
Methods to Write Specified Text to a File in Python -
Use the
write()
andwritelines()
Methods to Write Iterables to a File in Python
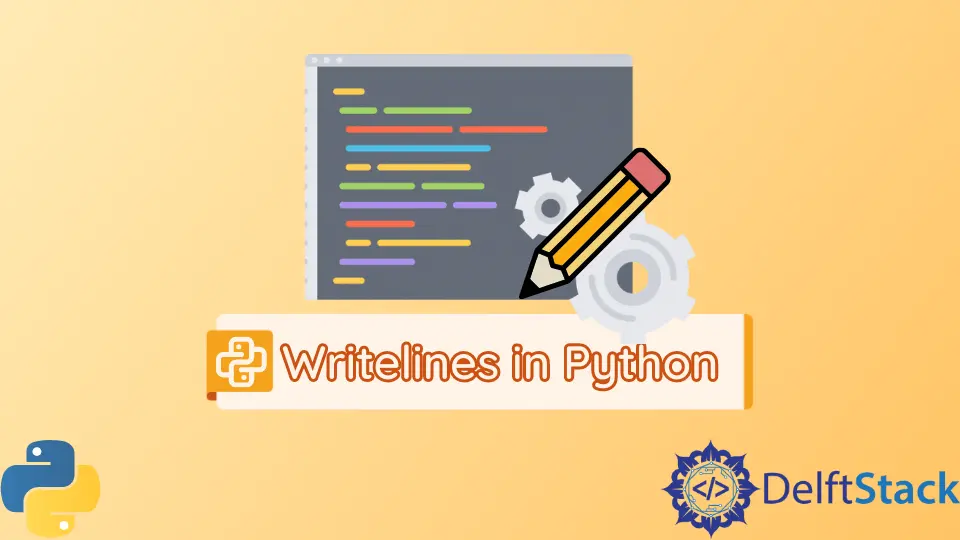
This tutorial will introduce and explain the difference between the write()
and writelines()
methods in Python.
Use the write()
and writelines()
Methods to Write Specified Text to a File in Python
The write()
method expects a string as an argument and writes it to the file. If we provide a list of strings, it will raise an exception. The writelines()
method expects an iterable argument. Also, the write()
method displays the output but does not provide a new line character, whereas the writelines()
method displays the output and provides a new line character at the end of the string. It is important to note that writelines()
do not automatically insert a new line after each item in the iterable. We have to provide a new line by ourselves. If we have many lines to write to a file, writelines()
could be a better option. It performs well because it doesn’t create a temporary concatenated string, just iterating over the lines.
In the program below, we opened the file samplefile.txt
in the write mode. The lines
variable is a tuple, i.e., an immutable collection of strings. When we try to execute the program, it raised an exception-type error. The arguments we provided to the write()
method is a tuple that caused the error. Here, we used the with
method for file handling. This method provides better syntax and exception handling. So, it is a good practice to use the with
method where applicable. The method is helpful because any files opened will be closed after one is done.
Example Code:
# python 3.x
nl = "\n"
line1 = "Good"
line2 = "Morning"
line3 = "Sunshine"
lines = line1, nl, line2, nl, line3, nl
with open("samplefile.txt", "w") as f:
f.write(lines)
Output:
TypeError: write() argument must be str, not tuple
In a similar context, when we use writelines()
, the program is executed successfully, and a file named samplefile.txt
is created where lines
is passed as an argument to the writelines()
method.
Example Code:
# python 3.x
nl = "\n"
line1 = "Good"
line2 = "Morning"
line3 = "Sunshine"
lines = line1, nl, line2, nl, line3, nl
with open("samplefile.txt", "w") as f:
f.writelines(lines)
Output:
Good
Morning
Sunshine
For the same program, the multiple lines are concatenated to a single string variable called lines_joined
, which is easily accepted as an argument for the write()
method.
Example Code:
# python 3.x
nl = "\n"
line1 = "Good"
line2 = "Morning"
line3 = "Sunshine"
lines_joined = line1 + nl + line2 + nl + line3 + nl
with open("samplefile.txt", "w") as f:
f.write(lines_joined)
Output:
Good
Morning
Sunshine
Use the write()
and writelines()
Methods to Write Iterables to a File in Python
The join()
method takes all items in an iterable and joins them into one string. In the program below, we used the join()
method with '\n'
as a glue to concatenate the string in lines
. It is a better approach for concatenation instead of using the +
operator.
Example Code:
# python 3.x
lines = ["Hello", "World"]
with open("hello.txt", "w") as f:
f.write("\n".join(lines))
Output:
Hello
World
It is less efficient to use the join()
method for an extremely long list of strings. In such a case, an entirely new and very long string is created in memory before writing it. So, in the program below, we used a generator expression to write the strings in the newline. Here, the data is written piece-wise.
Example Code:
# python 3.x
lines = ["Hello", "World"]
with open("hello.txt", "w") as f:
for l in lines:
f.write("%s\n" % l)
Output:
Hello
World
The same program as above is implemented through the writelines()
method. It makes the use of a generator expression and dynamically creates newline-terminated strings. The writelines()
method iterates over this sequence of strings and writes every item.
Example Code:
# python 3.x
lines = ["Hello", "World"]
with open("hello.txt", "w") as file:
file.writelines("%s\n" % t for t in texts)
Output:
Hello
World