How to Write Float Values to a File in Python
-
Method 1: Using the Built-in
open()
Function -
Method 2: Using the
csv
Module - Method 3: Using NumPy for Array-like Structures
- Method 4: Writing Float Values with Formatting
- Conclusion
- FAQ
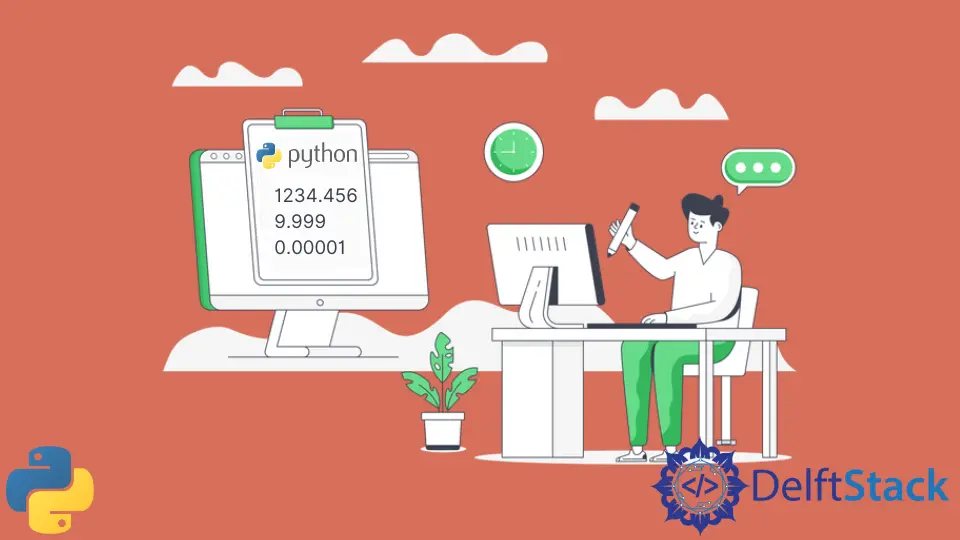
Writing float values to a file in Python may seem daunting at first, but it’s a straightforward process once you understand the basics. Whether you’re logging sensor data, saving scientific calculations, or exporting financial records, knowing how to handle float values efficiently can save you time and effort.
In this article, we’ll explore various methods for writing float values to a file in Python, complete with examples and detailed explanations. By the end, you’ll have a solid grasp of how to implement these techniques in your own projects. Let’s dive in!
Method 1: Using the Built-in open()
Function
One of the simplest ways to write float values to a file in Python is by using the built-in open()
function. This function allows you to create a file object that you can write to. Here’s how you can do it:
float_values = [1.23, 4.56, 7.89]
with open('float_values.txt', 'w') as file:
for value in float_values:
file.write(f"{value}\n")
Output:
1.23
4.56
7.89
In this example, we first create a list of float values. We then open a file named float_values.txt
in write mode ('w'
). The with
statement ensures that the file is properly closed after we’re done writing to it. Inside the loop, each float value is formatted as a string and written to the file, followed by a newline character. This method is efficient for writing multiple float values in a clean format.
Method 2: Using the csv
Module
If you need to write float values in a structured format, using the csv
module is an excellent choice. This module is designed for handling CSV (Comma-Separated Values) files, which are commonly used for data storage and exchange. Here’s how to write float values using this method:
import csv
float_values = [1.23, 4.56, 7.89]
with open('float_values.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(float_values)
Output:
1.23,4.56,7.89
In this code, we import the csv
module and create a list of float values. The open()
function is used again, but this time we specify the newline=''
parameter to avoid extra blank lines in the output file. We then create a CSV writer object and use the writerow()
method to write our float values as a single row in the CSV file. This method is particularly useful when dealing with tabular data, as it allows for easy reading and manipulation later.
Method 3: Using NumPy for Array-like Structures
For those working with numerical data, the NumPy library provides powerful tools for handling arrays and matrices. Writing float values to a file using NumPy is not only efficient but also supports various formats. Here’s how you can do it:
import numpy as np
float_values = np.array([1.23, 4.56, 7.89])
np.savetxt('float_values.txt', float_values)
Output:
1.23
4.56
7.89
In this example, we first import the NumPy library and create a NumPy array containing our float values. The savetxt()
function is then used to write the array to a text file. By default, this function writes the values in a simple format, one per line. NumPy’s savetxt()
function is particularly advantageous when working with large datasets, as it offers options to specify delimiters and formats.
Method 4: Writing Float Values with Formatting
Sometimes, you might want to control the formatting of your float values when writing them to a file. Python allows you to format numbers in various ways using string formatting techniques. Here’s an example:
float_values = [1.234567, 4.567890, 7.890123]
with open('formatted_float_values.txt', 'w') as file:
for value in float_values:
file.write(f"{value:.2f}\n")
Output:
1.23
4.57
7.89
In this code snippet, we format each float value to two decimal places using the format specifier :.2f
. This ensures that the output is cleaner and more readable, especially when dealing with financial or scientific data. The f-string
formatting provides a convenient way to control how many decimal places you want to display, allowing for greater flexibility in your output.
Conclusion
Writing float values to a file in Python is a manageable task with multiple approaches available. Whether you prefer the simplicity of the built-in open()
function, the structure of the csv
module, the efficiency of NumPy, or the precision of formatted strings, Python has you covered. Each method serves its purpose depending on your specific needs, so feel free to choose the one that best fits your project. With these techniques in your toolkit, you’ll be well-equipped to handle float values in a variety of scenarios.
FAQ
-
How do I write float values to a binary file in Python?
You can use thestruct
module to pack float values into binary format before writing them to a file. -
Can I read float values back from a file in Python?
Yes, you can use theopen()
function in read mode and convert the strings back to float usingfloat()
. -
Is it possible to append float values to an existing file?
Yes, you can open a file in append mode ('a'
) and write additional float values without overwriting existing content. -
What is the difference between
write()
andwritelines()
methods?
Thewrite()
method writes a single string to a file, whilewritelines()
can write a list of strings. -
Can I specify the precision of float values when writing to a file?
Yes, you can use formatted strings to control the number of decimal places when writing float values.