The while True Statement in Python
- Understanding the While True Statement
- Implementing While True for Continuous User Input
- Using While True in Event-Driven Programming
- Best Practices for Using While True
- Conclusion
- FAQ
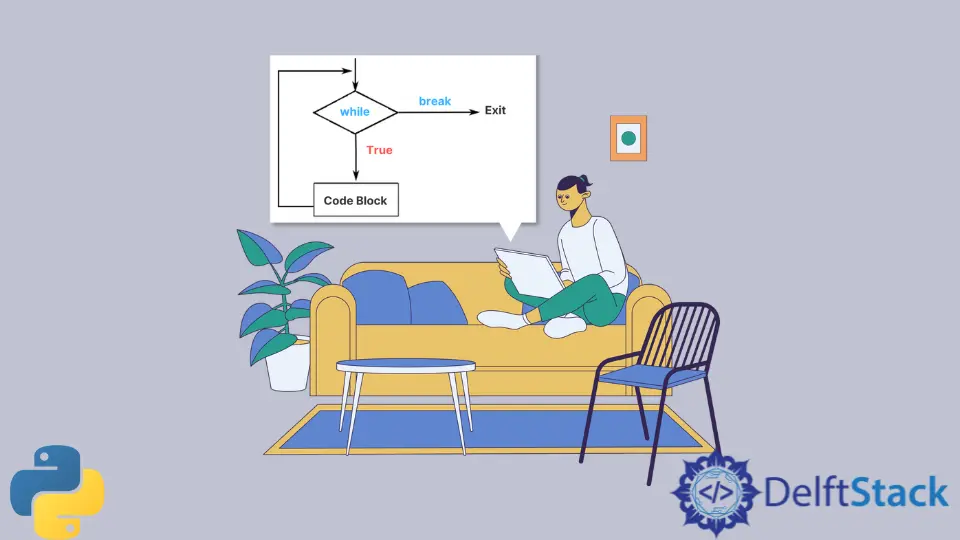
Infinite loops can be a powerful tool in programming, especially in Python. One of the most common ways to create an infinite loop is by using the while True
statement. This simple yet effective construct allows you to execute a block of code repeatedly until a specific condition is met, making it ideal for scenarios where you want continuous execution. Whether you’re building a server, a game, or any application that requires ongoing processes, understanding how to implement while True
can enhance your coding skills.
In this article, we will dive into the mechanics of the while True
statement, explore practical examples, and discuss best practices to avoid common pitfalls.
Understanding the While True Statement
At its core, the while True
statement creates an infinite loop. The loop will continue executing until it is explicitly broken out of, either by a break statement or an external condition. This makes it particularly useful for scenarios where you need to continuously check for certain conditions, such as user input or system status.
Here’s a simple example to illustrate how while True
functions:
while True:
user_input = input("Type 'exit' to quit: ")
if user_input.lower() == 'exit':
break
In this snippet, the program will keep asking the user for input indefinitely until they type ’exit’. The break
statement is what allows us to exit the loop gracefully.
Output:
Type 'exit' to quit: hello
Type 'exit' to quit: exit
This loop showcases how while True
can be controlled using conditions. It’s straightforward yet powerful, allowing for flexibility in your code.
Implementing While True for Continuous User Input
One of the most practical uses of the while True
statement is to continuously gather user input. This can be particularly useful in applications that require ongoing interaction, such as chatbots or command-line tools. By using while True
, you ensure that your program remains responsive and can handle multiple inputs without closing.
Here’s how you can implement this:
while True:
command = input("Enter a command (type 'quit' to exit): ")
if command.lower() == 'quit':
print("Exiting the program.")
break
else:
print(f"You entered: {command}")
In this example, the program prompts the user to enter a command repeatedly. If the user types ‘quit’, the program will exit; otherwise, it will display the entered command. This loop keeps the program active and responsive, allowing for a seamless user experience.
Output:
Enter a command (type 'quit' to exit): hello
You entered: hello
Enter a command (type 'quit' to exit): quit
Exiting the program.
The flexibility of while True
allows you to create interactive applications that can handle user input effectively. Just be cautious about ensuring there’s a clear exit condition to avoid unintentional infinite loops.
Using While True in Event-Driven Programming
Another area where while True
shines is in event-driven programming. In scenarios like game development or GUI applications, you often need to keep the application running to listen for events, such as user actions or system changes. The while True
statement can help manage this continuous listening process.
Here’s a basic example of how you might structure such a program:
while True:
event = wait_for_event() # Hypothetical function to wait for an event
if event == 'quit':
print("Shutting down the application.")
break
handle_event(event) # Hypothetical function to handle the event
In this code, the program continuously waits for events. If the event is ‘quit’, it exits the loop; otherwise, it processes the event. This pattern is common in applications that require constant monitoring of user interactions or system states.
Output:
Waiting for event...
Event received: click
Handling event: click
Waiting for event...
Event received: quit
Shutting down the application.
Using while True
in this context allows your application to remain active and responsive to user interactions, making it essential for real-time applications.
Best Practices for Using While True
While while True
is a powerful tool, it’s essential to use it wisely to avoid common pitfalls, such as unintentional infinite loops that can crash your program or consume excessive resources. Here are some best practices to keep in mind:
- Always Include an Exit Condition: Ensure that there is a clear and reachable condition to break out of the loop. Without this, your program may run indefinitely.
- Consider Resource Usage: Infinite loops can consume CPU resources if not managed properly. Implement sleep intervals or checks to prevent high resource usage.
- Use Exception Handling: Incorporate error handling to manage unexpected input or conditions that might cause the loop to fail.
- Test Thoroughly: Before deploying your application, test the loop under various conditions to ensure it behaves as expected.
By adhering to these practices, you can harness the power of while True
while minimizing the risks associated with infinite loops.
Conclusion
The while True
statement is a fundamental construct in Python that enables the creation of infinite loops. Whether you’re gathering user input, managing events, or building interactive applications, mastering this statement can significantly enhance your programming capabilities. Remember to implement exit conditions and follow best practices to ensure your loops function correctly and efficiently. With a solid understanding of while True
, you’ll be well-equipped to tackle a variety of programming challenges.
FAQ
-
What is the purpose of the while True statement in Python?
The while True statement creates an infinite loop that continues executing until explicitly broken out of. -
How can I exit a while True loop?
You can exit a while True loop using the break statement or by raising an exception. -
Are there performance concerns with using while True?
Yes, if not managed properly, while True loops can consume excessive CPU resources. It’s important to include exit conditions and manage resource usage. -
Can I use while True in event-driven programming?
Absolutely! While True is often used in event-driven programming to continuously listen for and respond to events. -
What are some common mistakes to avoid with while True?
Common mistakes include forgetting to include an exit condition, leading to unintentional infinite loops, and not considering resource consumption.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn