How to Get Union of Lists in Python
- Get Union of Two lists with repetition of common elements in Python
- Get Union of two List in sorted order in Python
- Get Union of Two Lists without repetition of common elements in Python
- Get Union of More than Two Lists in Python
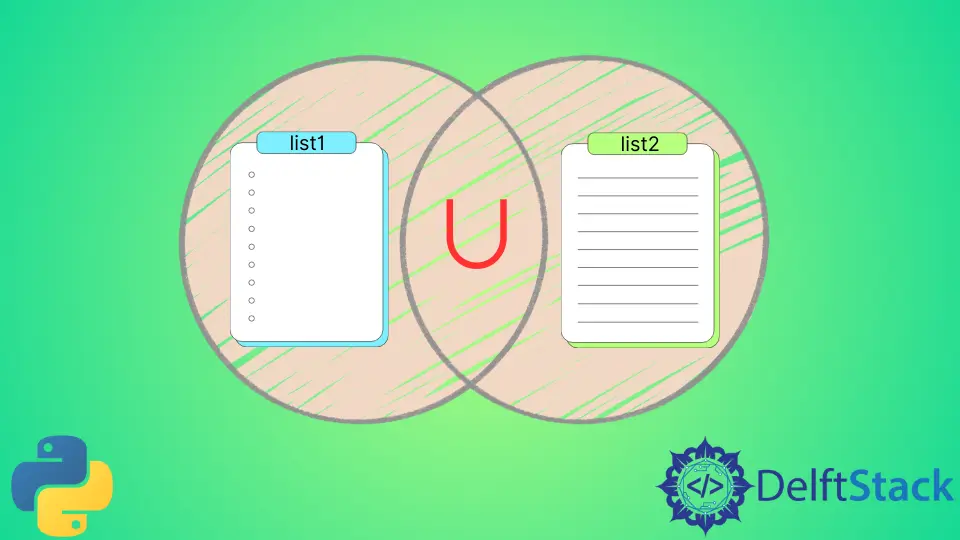
Union of lists means that all the elements from the different lists will be put up inside a single list. We can find a union of two or more than two lists. There are various methods to achieve a Union of Lists. All the methods are described below with sufficient code examples.
Get Union of Two lists with repetition of common elements in Python
We can add two lists using the +
operator to get the union of the two lists.
Example code:
l1 = [1, 2, 3, 4, 5]
l2 = [2, 4, 6.8, 10]
l3 = l1 + l2
print("l1: ", l1)
print("l2: ", l2)
print("Union of l1 and l2 with element repetition: ", l3)
Output:
l1: [1, 2, 3, 4, 5]
l2: [2, 4, 6.8, 10]
Union of l1 and l2 with element repetition: [1, 2, 3, 4, 5, 2, 4, 6.8, 10]
It finds the union of the lists l1
and l2
and stores the result in l3
. From the output, it is clear that we have repeated elements while finding union if we have the same element repeated in any of the list operands.
Get Union of two List in sorted order in Python
If we wish to find the union of Lists in a sorted manner, we use the sorted()
method to sort the list obtained after union operation.
Code :
l1 = [11, 20, 1, 2, 3, 4, 5]
l2 = [2, 4, 6, 8, 10]
union_l1_l2 = l1 + l2
l3 = sorted(union_l1_l2)
print("l1: ", l1)
print("l2: ", l2)
print("Sorted union of two l1 and l2 : ", l3)
Output:
l1: [11, 20, 1, 2, 3, 4, 5]
l2: [2, 4, 6, 8, 10]
Sorted union of two l1 and l2 : [1, 2, 2, 3, 4, 4, 5, 6, 8, 10, 11, 20]
It computes the union of lists l1
and l2
using the +
operator and stores the union in union_l1_l2
. We use the sorted()
method to sort the elements of the list union_l1_l2
to get the union of l1
and l2
in a sorted fashion.
Get Union of Two Lists without repetition of common elements in Python
In Python, sets are the data types that do not allow duplication of elements. So, we can use set()
to get the union of two lists without repetition of common elements.
Code :
def union_without_repetition(list1, list2):
result = list(set(list1 + list2))
return result
l1 = [1, 2, 3, 4, 5]
l2 = [2, 4, 6, 8, 10]
l3 = union_without_repetition(l1, l2)
print("l1: ", l1)
print("l2: ", l2)
print("Union of two l1 and l2 without repetition : ", l3)
Output:
l1: [1, 2, 3, 4, 5]
l2: [2, 4, 6, 8, 10]
Union of two l1 and l2 without repetition : [1, 2, 3, 4, 5, 6, 8, 10]
Here, we find the union of l1
and l2
using the +
operator and select only unique elements using the set()
function and then finally convert the set into a list using the list()
function.
Get Union of More than Two Lists in Python
We have already computed the union of the two lists. But, what to do in the case of finding a union of more than two lists. It is very simple. We can use both the set()
and union()
inbuilt python function to find the union of more than two lists.
Code :
def union(lst1, lst2, lst3):
final_list = list(set().union(lst1, lst2, lst3))
return final_list
l1 = [1, 2, 3, 4, 5]
l2 = [2, 4, 6, 8, 10]
l3 = [5, 6, 7, 8, 11, 15, 18]
print("l1: ", l1)
print("l2: ", l2)
print("l3 : ", l3)
print("Union of more than l1 l2 and l3: ", union(l1, l2, l3))
Output:
l1: [1, 2, 3, 4, 5]
l2: [2, 4, 6, 8, 10]
l3 : [5, 6, 7, 8, 11, 15, 18]
Union of more than l1 l2 and l3: [1, 2, 3, 4, 5, 6, 7, 8, 10, 11, 15, 18]
Here, we create a set
object using set()
constructor and then call union()
method for the object. The union()
method can take any number of list
objects and return their union.
In this case, as we are using the union()
method of the set
class, we don’t get repeated elements.
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python