How to Convert Sentence to Title Case in Python
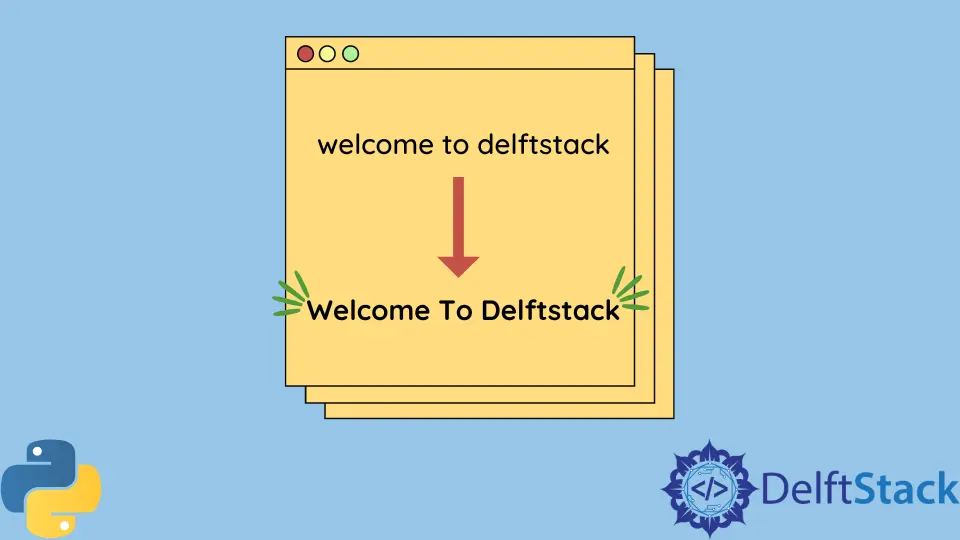
Title Case is a sentence style where the first letter of every major word is written in a capital letter, while for the minor words(such as articles or prepositions), the first letter is written in small. For Example: I Am a Programmer from France
is written in the title case.
To convert any sentence to a title case in Python, we can either use the title()
function with the string or use the titlecase()
function available with the titlecase
module.
Title Case Using the title()
Function in Python
We can call the title()
function with any string to convert it into a title case. This is a built-in method available with strings.
Example Code:
sentence = "i am a programmer from france and i am fond of python"
print("Sentence before:", sentence)
sentence = sentence.title()
print("Sentence now:", sentence)
Output:
Sentence before: i am a programmer from france and i am fond of python
Sentence now: I Am A Programmer From France And I Am Fond Of Python
Title Case Using the titlecase
Module in Python
Another way to convert a sentence into a title case is to import the titlecase
module and pass a string to its function titlecase()
. Note: This module should be installed first to use it.
Example Code:
from titlecase import titlecase
sentence = "i am a programmer from france and i am fond of python"
print("Sentence before:", sentence)
sentence = titlecase(sentence)
print("Sentence now:", sentence)
Output:
Sentence before: i am a programmer from france and i am fond of python
Sentence now: I Am A Programmer From France And I Am Fond Of Python
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn