Python Threadpool
- Understanding Python Threadpool
- Multiprocessing vs. multiprocessing.pool
- Using Multiprocessing
- Using multiprocessing.pool
- Conclusion
- FAQ
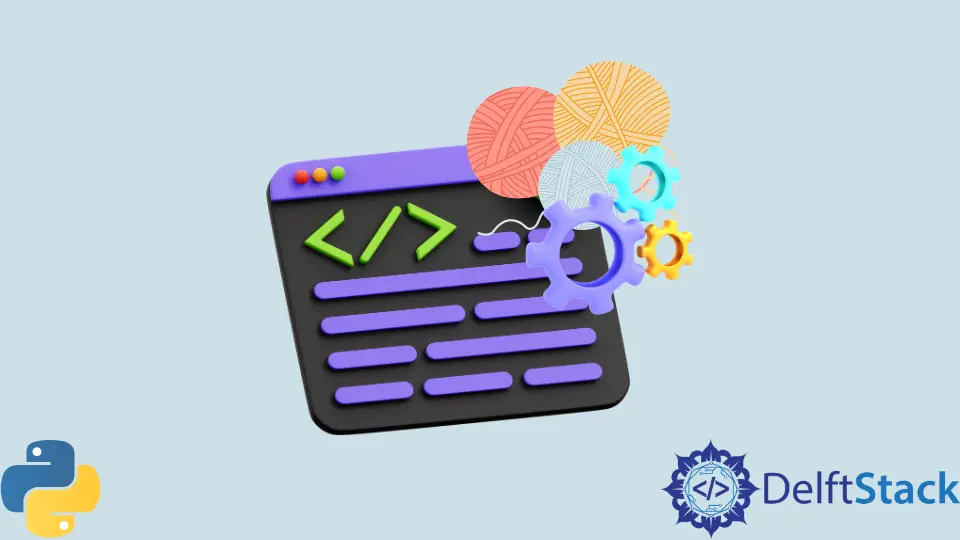
In today’s fast-paced tech landscape, efficiency is key, especially when it comes to executing tasks in parallel. Python offers various methods for achieving concurrency, with threading and multiprocessing being two of the most prominent. Among these, the Python threadpool stands out as a powerful tool for managing multiple threads simultaneously.
This article will delve into the differences between Python’s multiprocessing and the multiprocessing.pool module, shedding light on their unique features, benefits, and ideal use cases. By the end, you’ll have a clearer understanding of when to use each approach, helping you optimize your Python applications for better performance and resource management.
Understanding Python Threadpool
At its core, a threadpool in Python is a collection of threads that can be used to execute tasks concurrently. This allows for efficient management of multiple threads, reducing the overhead associated with creating and destroying threads repeatedly. The threadpool concept is particularly useful when dealing with I/O-bound operations, where tasks spend a significant amount of time waiting for external resources, such as file systems or network responses.
In contrast, the multiprocessing module is designed for CPU-bound tasks, where the goal is to utilize multiple CPU cores to speed up processing. The multiprocessing.pool module extends this functionality by providing a convenient way to manage a pool of worker processes, allowing for easy distribution of tasks across multiple processes.
Multiprocessing vs. multiprocessing.pool
When deciding between using the multiprocessing module and the multiprocessing.pool module, it’s essential to understand the key differences. The multiprocessing module provides a lower-level interface for creating and managing processes, giving you more control over how tasks are executed. However, this increased control comes at the cost of added complexity.
On the other hand, the multiprocessing.pool module abstracts much of the complexity involved in process management. It allows you to create a pool of worker processes and submit tasks for execution with minimal boilerplate code. This makes it an excellent choice for users who want to quickly implement parallel processing without diving deep into the intricacies of process management.
Using Multiprocessing
To illustrate the use of the multiprocessing module, let’s look at a simple example of how to create and manage processes. In this example, we’ll create a function that simulates a CPU-bound task and then spawn multiple processes to execute that function concurrently.
import multiprocessing
import time
def cpu_bound_task(n):
time.sleep(1)
return n * n
if __name__ == "__main__":
with multiprocessing.Pool(processes=4) as pool:
results = pool.map(cpu_bound_task, range(10))
print(results)
Output:
[0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
In this example, we define a function cpu_bound_task
that takes an integer and returns its square after a brief sleep. We then create a pool of four processes using multiprocessing.Pool
. The map
method allows us to apply the cpu_bound_task
function to a range of numbers concurrently. The results are collected in a list and printed out. This method is particularly effective for CPU-bound tasks, as it leverages multiple cores to speed up computation.
Using multiprocessing.pool
Now, let’s explore the multiprocessing.pool module in a bit more detail. The pool module simplifies the process of managing a pool of worker processes. It allows for easy task submission and retrieval of results, making it an ideal choice for many parallel processing scenarios.
from multiprocessing import Pool
import time
def process_task(x):
time.sleep(2)
return x * x
if __name__ == "__main__":
pool = Pool(processes=4)
inputs = list(range(8))
outputs = pool.map(process_task, inputs)
pool.close()
pool.join()
print(outputs)
Output:
[0, 1, 4, 9, 16, 25, 36, 49]
This example uses the Pool
class from the multiprocessing
module to create a pool of four processes. The process_task
function simulates a time-consuming operation by sleeping for two seconds before returning the square of the input. The map
function submits the inputs for processing, and the results are collected in a list. After processing, we close the pool and wait for all worker processes to finish using join
. This approach is particularly beneficial when you have a large amount of data to process, as it efficiently distributes the workload across the available processes.
Conclusion
In conclusion, understanding the differences between Python’s multiprocessing and multiprocessing.pool modules is crucial for optimizing your applications. While the multiprocessing module offers more control over process management, the multiprocessing.pool module provides a simpler interface for handling parallel tasks. By choosing the right approach based on your specific needs—whether CPU-bound or I/O-bound—you can enhance the performance of your Python applications significantly. Embrace the power of Python threadpool and multiprocessing to take your coding skills to the next level.
FAQ
-
What is a threadpool in Python?
A threadpool is a collection of threads that can be used to execute tasks concurrently, improving efficiency and resource management. -
When should I use multiprocessing over multiprocessing.pool?
Use multiprocessing for more control over process management, while multiprocessing.pool is ideal for simpler task distribution and management. -
Are threadpools suitable for CPU-bound tasks?
Threadpools are generally more suited for I/O-bound tasks. For CPU-bound tasks, consider using the multiprocessing module.
-
How can I measure the performance of my multiprocessing code?
You can use the time module to measure the execution time of your functions and compare the performance of different implementations. -
Can I mix threading and multiprocessing in a Python application?
Yes, you can combine threading and multiprocessing in a Python application, but be cautious about potential complications with shared resources and data.