Python-Threadpool
- Thread-Pool-Definition
-
Unterschiede zwischen
multiprocessing.pool.ThreadPool
undmultiprocessing.Pool
-
multiprocessing.Pool
in Python -
multiprocessing.pool.Threadpool
in Python
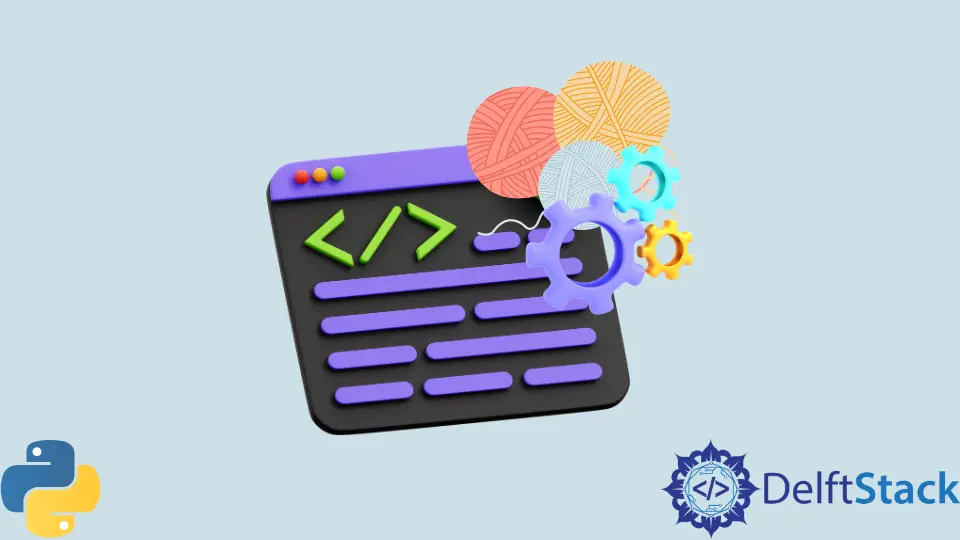
Dieses Tutorial zeigt den Unterschied zwischen Pool
von multiprocessing
und ThreadPool
von multiprocessing.pool
Thread-Pool-Definition
Ein Thread-Pool ist eine Gruppe von vorinstanziierten, inaktiven Threads, die bereit sind, ihnen Arbeit zu geben. Das Erstellen eines neuen Thread-Objekts für jede asynchron auszuführende Aufgabe ist teuer. Bei einem Thread-Pool würden Sie die Aufgabe zu einer Aufgabenwarteschlange hinzufügen, und der Thread-Pool weist der Aufgabe einen verfügbaren Thread zu. Der Thread-Pool hilft zu vermeiden, mehr Threads als nötig zu erstellen oder zu zerstören.
Unterschiede zwischen multiprocessing.pool.ThreadPool
und multiprocessing.Pool
multiprocessing.pool.ThreadPool
verhält sich genauso wie multiprocessing.Pool
. Der Unterschied besteht darin, dass multiprocessing.pool.Threadpool
Threads verwendet, um die Logik des Workers auszuführen, während multiprocessing.Pool
Worker-Prozesse verwendet.
multiprocessing.Pool
in Python
Der folgende Code erzeugt 4 verschiedene Prozesse, die jeweils die Funktion sleepy()
ausführen.
# python 3.x
from multiprocessing import Pool
import os
import time
def sleepy(x):
print("Process Id", os.getpid())
time.sleep(x)
return x
if __name__ == "__main__":
p = Pool(5)
pool_output = p.map(sleepy, range(4))
print(pool_output)
Ausgabe:
Process Id 239
Process Id 240
Process Id 241
Process Id 242
[0, 1, 2, 3]
multiprocessing.pool.Threadpool
in Python
ThreadPool
generiert 4 Threads, die die Funktion sleepy()
anstelle von Worker-Prozessen ausführen.
# python 3.x
from multiprocessing.pool import ThreadPool
import os
import time
def sleepy(x):
print("Process Id", os.getpid())
time.sleep(x)
return x
if __name__ == "__main__":
p = ThreadPool(5)
pool_output = p.map(sleepy, range(4))
print(pool_output)
Ausgabe:
Process Id 217
Process Id 217
Process Id 217
Process Id 217
[0, 1, 2, 3]