Timer Class in the Threading Module in Python
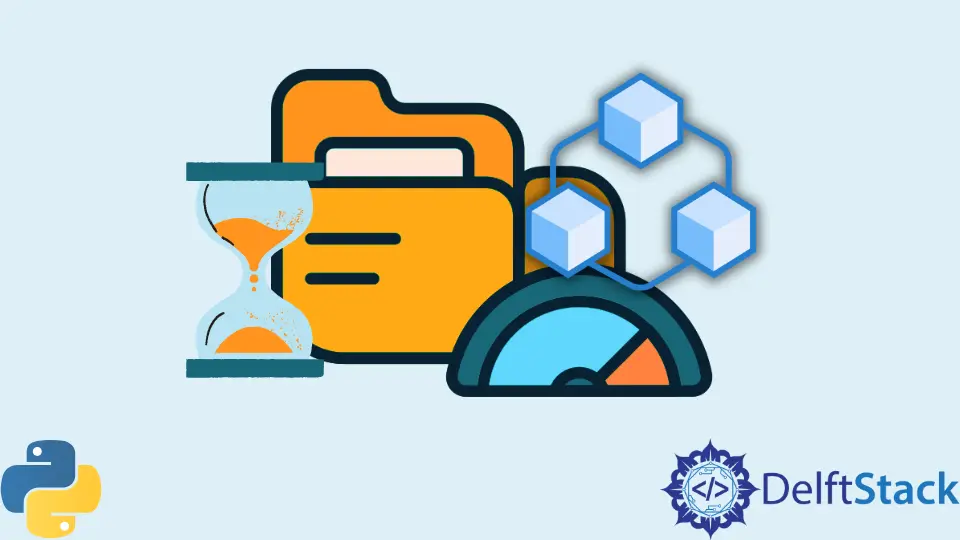
Threading is the technique of running multiple threads concurrently to achieve parallelism. In Python, we can implement threading using the threading
module. Now, the threading
module has a class Timer
, which can be used to perform some action or run some code after x
amount of time. In this article, we will see how we can use this class and understand it better with the help of an example. We will build an infinite timer.
the threading.Timer
Class in Python
The Timer
class is a subclass of the Thread
class and can be used to execute code after some units of time. It accepts two arguments, namely, interval
and function
. interval
refers to the number of seconds after which the code should be executed, and function
is the call back function that should be called when the required time has elapsed. This class has two important functions, namely, start()
and cancel()
. start()
method is used to start the timer and cancel()
method is used to cancel it.
Timer objects don’t start by default. We have to call the start()
method to launch them. And to stop a running timer, we can use the cancel()
method.
Now that we are done with the theory, let us understand how we can practically use this class to create an infinite timer. Refer to the following code for the same.
from time import sleep
from threading import Timer
from datetime import datetime
class MyInfiniteTimer:
"""
A Thread that executes infinitely
"""
def __init__(self, t, hFunction):
self.t = t
self.hFunction = hFunction
self.thread = Timer(self.t, self.handle_function)
def handle_function(self):
self.hFunction()
self.thread = Timer(self.t, self.handle_function)
self.thread.start()
def start(self):
self.thread = Timer(self.t, self.handle_function)
self.thread.start()
def cancel(self):
self.thread.cancel()
def print_current_datetime():
print(datetime.today())
t = MyInfiniteTimer(1, print_current_datetime)
t.start()
sleep(5)
t.cancel()
sleep(5)
t.start()
sleep(5)
t.cancel()
Output:
2021-10-31 05:51:20.754663
2021-10-31 05:51:21.755083
2021-10-31 05:51:22.755459
2021-10-31 05:51:23.755838
2021-10-31 05:51:24.756172
2021-10-31 05:51:30.764942
2021-10-31 05:51:31.765281
2021-10-31 05:51:32.765605
2021-10-31 05:51:33.766017
2021-10-31 05:51:34.766357
As we can see, the MyInfiniteTimer
class uses the Timer
class. It accepts two arguments: t
and hFunction
, which refer to the number of seconds and the call back function for the Timer
object. When a MyInfiniteTimer
class object is created, the class’s constructor creates a new timer object but does not start it. The timer can be launched using the start()
method of the MyInfiniteTimer
class. And using the stop()
method, the timer can be stopped. Once the current timer ends, the handler or the handle_function()
creates a new timer with the same configurations as the previous timer and starts it.
To showcase the working of the MyInfiniteTimer
class, we first created a new MyInfiniteTimer
class object at line 29
and then called the start()
method. Next, we waited for 5
seconds or let the timer run for 5
seconds. Then we stopped the timer and again waited for 5
seconds. Lastly, we repeated the last two processes, and then the program terminated.