Python 线程模块中的 Timer 类
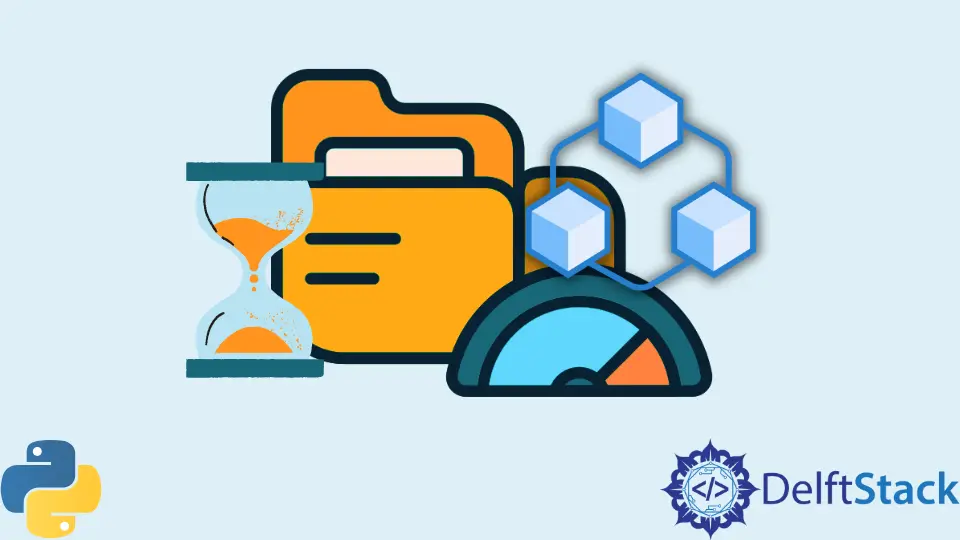
线程是并发运行多个线程以实现并行性的技术。在 Python 中,我们可以使用 threading
模块来实现线程化。现在,threading
模块有一个 Timer
类,可用于在 x
时间后执行某些操作或运行某些代码。在本文中,我们将通过示例了解如何使用该类并更好地理解它。我们将构建一个无限计时器。
Python 中的 threading.Timer
类
Timer
类是 Thread
类的子类,可用于在一些时间单位后执行代码。它接受两个参数,即 interval
和 function
。interval
是指代码应该执行的秒数,而 function
是在所需时间过去后应该调用的回调函数。这个类有两个重要的函数,即 start()
和 cancel()
。start()
方法用于启动计时器,cancel()
方法用于取消它。
计时器对象默认不启动。我们必须调用 start()
方法来启动它们。要停止正在运行的计时器,我们可以使用 cancel()
方法。
现在我们已经完成了理论,让我们了解如何实际使用这个类来创建一个无限计时器。相同的参考下面的代码。
from time import sleep
from threading import Timer
from datetime import datetime
class MyInfiniteTimer:
"""
A Thread that executes infinitely
"""
def __init__(self, t, hFunction):
self.t = t
self.hFunction = hFunction
self.thread = Timer(self.t, self.handle_function)
def handle_function(self):
self.hFunction()
self.thread = Timer(self.t, self.handle_function)
self.thread.start()
def start(self):
self.thread = Timer(self.t, self.handle_function)
self.thread.start()
def cancel(self):
self.thread.cancel()
def print_current_datetime():
print(datetime.today())
t = MyInfiniteTimer(1, print_current_datetime)
t.start()
sleep(5)
t.cancel()
sleep(5)
t.start()
sleep(5)
t.cancel()
输出:
2021-10-31 05:51:20.754663
2021-10-31 05:51:21.755083
2021-10-31 05:51:22.755459
2021-10-31 05:51:23.755838
2021-10-31 05:51:24.756172
2021-10-31 05:51:30.764942
2021-10-31 05:51:31.765281
2021-10-31 05:51:32.765605
2021-10-31 05:51:33.766017
2021-10-31 05:51:34.766357
正如我们所见,MyInfiniteTimer
类使用了 Timer
类。它接受两个参数:t
和 hFunction
,它们指的是秒数和 Timer
对象的回调函数。创建 MyInfiniteTimer
类对象时,该类的构造函数会创建一个新的计时器对象,但不会启动它。可以使用 MyInfiniteTimer
类的 start()
方法启动计时器。并使用 stop()
方法,可以停止计时器。当前计时器结束后,处理程序或 handle_function()
会创建一个与前一个计时器具有相同配置的新计时器并启动它。
为了展示 MyInfiniteTimer
类的工作,我们首先在 29
行创建了一个新的 MyInfiniteTimer
类对象,然后调用了 start()
方法。接下来,我们等待 5
秒或让计时器运行 5
秒。然后我们停止计时器并再次等待 5
秒。最后,我们重复最后两个过程,然后程序终止。