Python Static Method
- What is a Static Method?
- When to Use Static Methods
- Benefits of Using Static Methods
- Common Misconceptions About Static Methods
- Conclusion
- FAQ
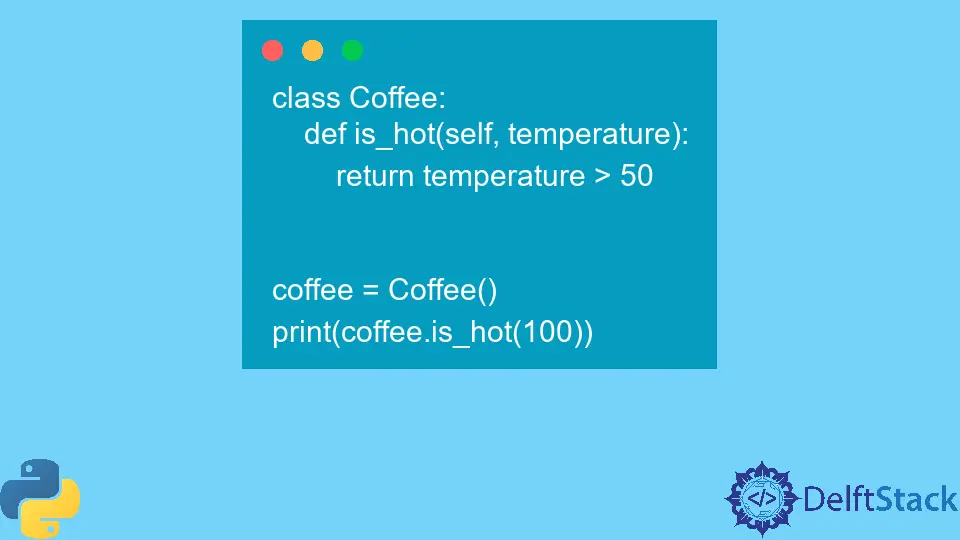
Static methods in Python are a powerful feature that allows you to define methods that belong to a class rather than an instance of the class. This means you can call these methods without needing to create an object of the class. If you’re looking to streamline your code and enhance its organization, understanding how to create and utilize static methods is essential.
In this article, we’ll explore the concept of static methods in Python, how to implement them, and when to use them effectively. By the end, you’ll have a solid grasp of this important aspect of Python programming, helping you write cleaner and more efficient code.
What is a Static Method?
Static methods are defined using the @staticmethod
decorator in Python. Unlike instance methods, static methods do not require a reference to the instance or the class. This makes them particularly useful for utility functions that perform a task in isolation from the class’s state. By using static methods, you can encapsulate functionality that logically belongs to the class but does not need to access or modify any instance-specific data.
Here’s how to define a static method:
class MathOperations:
@staticmethod
def add(x, y):
return x + y
result = MathOperations.add(5, 10)
Output:
15
In this example, we define a class called MathOperations
with a static method add
. This method takes two parameters, x
and y
, and returns their sum. Notice that we call MathOperations.add(5, 10)
without creating an instance of the class. This illustrates the core benefit of static methods: they can be called directly on the class itself.
When to Use Static Methods
Choosing when to use static methods can significantly improve the clarity and maintainability of your code. Static methods are ideal in scenarios where you need a utility function that does not need access to instance or class-level data. For example, if you have a method that validates input or formats data, a static method is a perfect fit.
Consider the following example:
class StringUtilities:
@staticmethod
def is_palindrome(s):
return s == s[::-1]
result = StringUtilities.is_palindrome("racecar")
Output:
True
In this code, we define a static method is_palindrome
within the StringUtilities
class. This method checks if the provided string is a palindrome. Since it does not rely on any instance variables, it makes sense to implement it as a static method. By using static methods in this way, you can create a library of utility functions that are easy to access and maintain.
Benefits of Using Static Methods
Static methods come with several advantages that can enhance your programming practices. First, they promote code reusability. Since static methods can be called on the class itself, you can use them across different parts of your application without needing to instantiate the class. This reduces memory overhead and increases efficiency.
Moreover, static methods contribute to better organization of code. By grouping related utility functions within a class, you create a logical structure that makes the codebase easier to navigate. This is particularly beneficial in larger projects where maintaining clarity is crucial.
Here’s a practical example of a static method that converts temperatures:
class TemperatureConverter:
@staticmethod
def celsius_to_fahrenheit(celsius):
return (celsius * 9/5) + 32
result = TemperatureConverter.celsius_to_fahrenheit(25)
Output:
77.0
In this case, the celsius_to_fahrenheit
method converts a temperature from Celsius to Fahrenheit. It’s a self-contained function that does not depend on any instance data. By encapsulating this functionality within a class, you maintain a clean and organized code structure.
Common Misconceptions About Static Methods
One common misconception about static methods is that they are the same as class methods. While both are associated with a class rather than an instance, class methods can access class-level data and modify it. In contrast, static methods cannot access or modify class or instance data. Understanding this distinction is vital for effective use of both method types.
Another misconception is that static methods are less flexible or powerful than instance methods. While it’s true that they lack access to instance-specific data, this limitation is also their strength. Static methods enforce a clear separation of concerns, allowing you to create functions that are straightforward and predictable.
To illustrate this further, consider a class method example:
class Counter:
count = 0
@classmethod
def increment(cls):
cls.count += 1
Counter.increment()
Output:
1
In this example, increment
is a class method that modifies the class variable count
. This is something a static method could not do, highlighting the unique roles each method type plays in Python.
Conclusion
In summary, static methods in Python are a valuable tool for creating utility functions that belong to a class but do not require access to instance or class data. They enhance code organization, promote reusability, and clarify the intention behind your methods. By understanding when and how to use static methods, you can write cleaner and more efficient code. Whether you’re a beginner or an experienced developer, mastering static methods will undoubtedly improve your Python programming skills.
FAQ
-
What is a static method in Python?
A static method is a method that belongs to a class rather than an instance and does not require access to instance or class-specific data. -
How do you define a static method in Python?
You define a static method using the@staticmethod
decorator above the method definition within a class. -
When should I use static methods?
Use static methods for utility functions that do not need to access or modify class or instance data. -
Can static methods access instance variables?
No, static methods cannot access instance variables or class variables. -
What is the difference between static methods and class methods?
Static methods do not have access to class or instance data, while class methods can access and modify class-level data using thecls
parameter.