The sprintf() Function in Python
-
Understanding
sprintf()
in Other Languages - String Formatting in Python
-
String Formatting Using the
%
Operator in Python -
String Formatting Using the
format()
Function in Python -
Formatted String Literal
f-string
in Python -
How to Use
sprintf
in Python: Customsprintf
Function - Conclusion
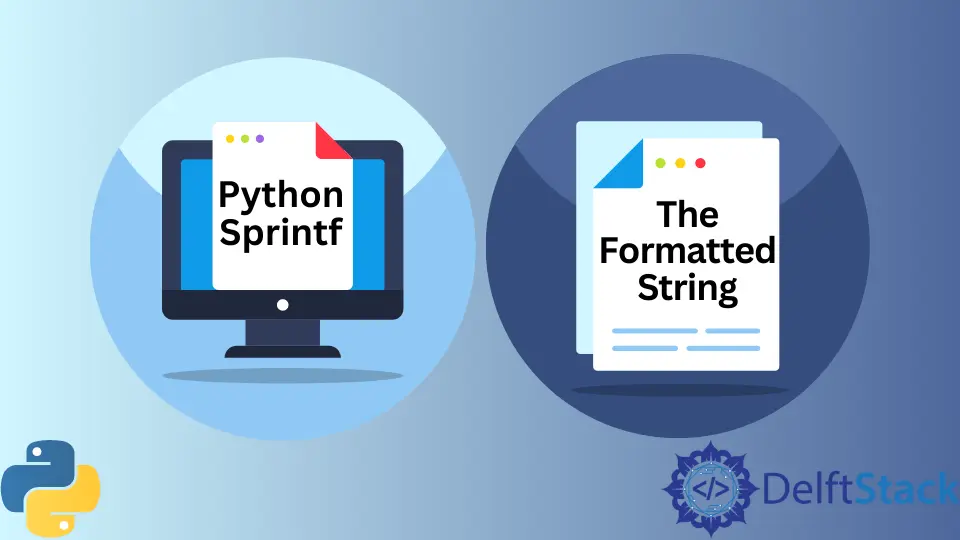
In Python, the sprintf()
function, unlike other languages like C or C++, doesn’t have a direct counterpart in the standard library. Instead, Python offers various formatting mechanisms, including the format()
method and f-strings
, which provide similar functionality to sprintf()
in other languages.
There isn’t the exact sprintf
built-in function to print formatted strings, but thanks to Python, numerous methods exist to print formatted strings. In this article, we will cover four sprintf
like functionality in Python, including the %
operator, format()
, f-string
, and a user-defined sprintf
function, so let’s dive deep into it.
Understanding sprintf()
in Other Languages
sprintf()
is a function present in many programming languages, primarily used for string formatting. It allows developers to create formatted strings by inserting values into a predefined string template, similar to how placeholders work in Python’s format()
or f-string
mechanisms.
String Formatting in Python
String formatting is also addressed as string interpolation, where we can insert a custom value, string, or variable in the predefined text. It is one of the preferred and readable methods for printing strings, including some values or results, which gives us the sprintf
like functionality in Python.
We can also use mathematical expressions in the formatted strings. For example, let us say we have two values and want to add them to print a final result.
x = 3
y = 4
print(f"The sum of {x} and {y} = {x+y}") # formatted string
print("The sum of", x, "and", y, "=", (x + y)) # normal string
Output:
The sum of 3 and 4 = 7
The sum of 3 and 4 = 7
As we can see, the result of the above is the same, but the normal string is very junky; however, the formatted string is more readable, and it is easy for developers to write code using the formatted string.
Python is a general-purpose programming language with a diverse open-source community and continuous support that offers solutions and functionalities to developers to achieve any task in numerous ways, depending upon the nature of the job and the particular scenario.
Similarly to using formatted strings, we have different methods that we can use to print formatting strings which we will discuss in this article one by one.
String Formatting Using the %
Operator in Python
In Python, the %
operator is used for string formatting. It allows you to create formatted strings by inserting values into a string template using placeholders.
The %
operator is used with a string on the left side containing placeholders and a tuple or dictionary of values on the right side. The placeholders in the string are represented by %
followed by a character that specifies the type of value to be inserted.
Basic Syntax
For Positional Formatting:
formatted_string = "String with placeholders: %s %d %f" % (
string_value,
int_value,
float_value,
)
%s
,%d
,%f
: These are placeholders in the string template. They indicate the type of value that will replace them (string, integer, floating-point number).(string_value, int_value, float_value)
: These values are passed in a tuple in the order that corresponds to the placeholders in the string template.
For Named Formatting (Using Dictionary):
formatted_string = (
"String with named placeholders: %(string)s %(integer)d %(float)f"
% {"string": string_value, "integer": int_value, "float": float_value}
)
%()
syntax: In named formatting, placeholders are enclosed within%()
with a dictionary passed on the right side.%(
and)s
,%(
and)d
: These are named placeholders in the string template. They specify the type of value to replace them and use keys from the dictionary.{'string': string_value, 'integer': int_value}
: This dictionary contains key-value pairs where keys correspond to the named placeholders in the string template.
It is one of the old techniques for formatted strings in Python and other programming languages. In Python, strings have a built-in operation accessible using the %
operator, allowing us to do string formatting easily, similar to sprintf
in C and C++.
Code Example:
name = "Zeeshan Afridi"
msg = "Hey! developers, it's %s." % name
print(msg)
Output:
Hey! developers, it's Zeeshan Afridi.
In the above code, %s
alarms the compiler that we are expecting a string at the end, and as demonstrated, we have specified the string with the %
operator as %name
.
There are different format specifiers for different data types in string formatting:
Format Specifier | Data Type | Output |
---|---|---|
%c |
char | It shows a Unicode character |
%d |
integer | Integer |
%f |
Floating point | Decimal number |
%o |
integer (octal) | Octal number |
%s |
String | It gives a string |
%t |
Date and Time | Data and time |
Note:
- The
%
operator for string formatting is older and less recommended compared to newer methods likestr.format()
orf-strings
due to limitations in flexibility and readability. - This method has been mostly replaced by the more modern and versatile formatting options available in Python, such as
format()
andf-strings
.
String Formatting Using the format()
Function in Python
The format()
method in Python is used for string formatting. It allows you to create formatted strings by replacing placeholders within a string template with specified values.
The format()
method is a new method for formatting strings in Python, and it was introduced in Python 3. It is a new style for formatting strings where we don’t need to remember the format specifiers and their sequence in the string; instead, we can use the format()
at the end of the string and provide the arguments at the specified positions.
Basic Syntax
The format()
method can be applied to a string and uses curly braces {}
as placeholders where values will be inserted.
Using Indexed Placeholders:
formatted_string = "String with placeholders: {} {} {}".format(value1, value2, value3)
Using Indexed Placeholders with Positions:
formatted_string = "String with placeholders: {0} {1} {2}".format(
value1, value2, value3
)
Using Named Placeholders:
formatted_string = "String with named placeholders: {name} {age}".format(
name="Alice", age=30
)
Parameters in the Syntax:
- Placeholders: Enclosed within curly braces
{}
or{index}
, whereindex
refers to the index or name of the value to be inserted. - Values: These are the values to be inserted into the placeholders. They can be provided in order for indexed placeholders or as keyword arguments for named placeholders.
Placeholder Types:
{}
: Indexed placeholders. Values are inserted in the order they appear.{index}
: Indexed placeholders with specified positions for values.{name}
: Named placeholders where values are specified using keyword arguments.
Code Example:
program = "BSSE"
cgpa = 3.2
student = "Hi! I am a student of {}, and my CGPA is {}".format(program, cgpa)
print(student)
Output:
Hi! I am a student of BSSE, and my CGPA is 3.2
In the above program, we have specified the positions where we wanted to insert a value with curly brackets {}
, and at the end of the string student
, we have used the .format()
function and given it two arguments accordingly.
Note:
- The
format()
method is more versatile and recommended compared to the older%
operator for string formatting. - It provides greater flexibility in formatting and is more readable, supporting both positional and named placeholders along with various formatting options.
Formatted String Literal f-string
in Python
Formatted string literal is also known as f-string
, and it is included in Python 3.6 as a new string formatting method whose syntax is more readable and straightforward. f-string
has an excellent and unique feature that the other methods were missing out, and we can define the expressions and values inside the string.
Basic Syntax
Using f-strings
:
variable = "value"
formatted_string = f"This is an {variable} formatted using f-string."
Embedding Expressions:
name = "Alice"
age = 30
formatted_string = f"Name: {name}, Age: {age}"
Parameters in the Syntax:
- Prefix
f
: Thef
prefix before the string literal indicates that it’s anf-string
, allowing the string to contain expressions enclosed in curly braces{}
. - Expressions within Curly Braces: Enclosed expressions within curly braces
{}
are evaluated, and their values are inserted into the string.
There isn’t any need to use .format()
at the end of the string. Let’s see an example of f-String
in Python below.
my_fav_lang = "Python"
experience = 2
zeeshan = f"I love to code in {my_fav_lang}, and I have {experience} years experience in Python programming."
print(zeeshan)
Output:
I love to code in Python, and I have 2 years experience in Python programming.
The f-string
is super easy and readable. Unlike the other two methods, we don’t need to use any other method or commas but only use f
as a prefix for the string, and we are good to go.
How to Use sprintf
in Python: Custom sprintf
Function
sprintf
stands for string print; basically, it is a built-in function in C and C++ programming language to print out a formatted string, and it stores the output on a char
buffer specified in sprintf()
. And there are different but similar ways in Python.
Comparing With sprintf()
The sprintf()
function in languages like C allows for placeholder-based formatting where placeholders like %s
, %d
, and %f
are used to insert string, integer, and floating-point values, respectively, into a string template. The equivalent functionalities in Python can be achieved using the format()
method or f-strings
.
The sprintf
isn’t a user-defined function in Python, but we can use the StringIO
instance as a buffer. And unlike the sprintf
, we must pass a string to the buf.write
and the formatting method. The %
is used for string formatting in this case.
Code Example:
import io
from io import StringIO
def sprintf(buf, fmt, *args):
buf.write(fmt % args)
age = 24
fav_color = "Blue"
buf = io.StringIO()
sprintf(buf, "I'm %d years old, and my favourite colour is %s!", age, fav_color)
print(buf.getvalue())
This code defines a custom sprintf
function that mimics the behavior of the sprintf
function in C. It takes a format string, fills in placeholders with provided values, and writes the formatted string to an in-memory buffer.
The formatted string is then retrieved from the buffer and printed. In this specific example, it combines the age
and fav_color
variables into a formatted string and outputs it as a sentence.
Output:
I'm 24 years old, and my favourite colour is Blue!
If you get any ImportError
for this program, install the required dependencies from your command line interface (CLI
).
Conclusion
In Python, the absence of a direct sprintf()
function as seen in C or C++ is compensated by versatile string formatting methods:
%
Operator: Allows placeholder-based formatting, akin tosprintf()
in C, using%
followed by format specifiers to insert values into a string template.format()
Method: Offers a more flexible approach, utilizing{}
as placeholders to insert values in order or as named arguments.f-strings
(f"..."
): Introduced in Python 3.6, it provides a concise way to embed expressions and variables directly within string literals for dynamic formatting.- Custom
sprintf
Function: Achievable in Python by utilizing tools likeStringIO
as a buffer to replicatesprintf()
behavior, combining a format string with provided arguments.
These diverse formatting mechanisms offer readability, flexibility, and ease of use, compensating for the absence of a direct sprintf()
function in Python.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn