How to Split List Into Chunks in Python
- Split List in Python to Chunks Using the List Comprehension Method
-
Split List in Python to Chunks Using the
itertools
Method -
Split List in Python to Chunks Using the
lambda
Function -
Split List in Python to Chunks Using the
lambda
&islice
Method -
Split List in Python to Chunks Using the
NumPy
Method - Split List Into Chunks in Python Using a User Defined Function
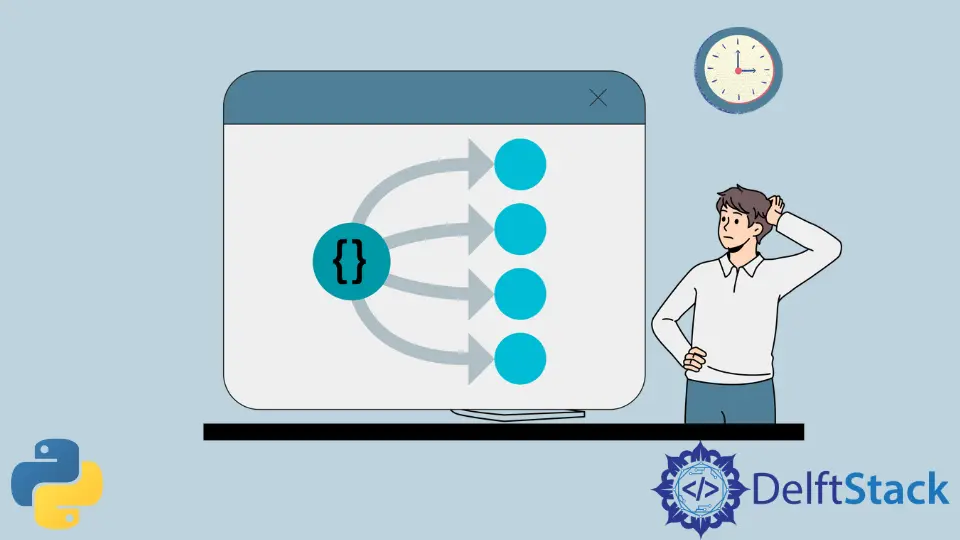
One of the Python data structures that can contain mixed values or elements within it is called lists. This article will introduce various ways to split a list into chunks. You can use any code example that fits your specifications.
Split List in Python to Chunks Using the List Comprehension Method
We can use list comprehension to split a Python list into chunks. It is an efficient way to encapsulate the operations to make the code easier to understand.
The complete example code is given below.
test_list = ["1", "2", "3", "4", "5", "6", "7", "8", "9", "10"]
n = 3
output = [test_list[i : i + n] for i in range(0, len(test_list), n)]
print(output)
Output:
[['1', '2', '3'], ['4', '5', '6'], ['7', '8', '9'], ['10']]
range(0, len(test_list), n)
returns a range of numbers starting from 0 and ending at len(test_list)
with a step of n
. For example, range(0, 10, 3)
will return (0, 3, 6, 9)
.
test_list[i:i + n]
gets the chunk of the list that starts at the index i
and ends exclusively at i + n
. The last chunk of the split list is test_list[9]
, but the calculated indices test_list[9:12]
will not raise an error but be equal to test_list[9]
.
Split List in Python to Chunks Using the itertools
Method
This method provides a generator that must be iterated using a for
loop. A generator is an efficient way of describing an iterator.
from itertools import zip_longest
test_list = ["1", "2", "3", "4", "5", "6", "7", "8", "9", "10"]
def group_elements(n, iterable, padvalue="x"):
return zip_longest(*[iter(iterable)] * n, fillvalue=padvalue)
for output in group_elements(3, test_list):
print(output)
Output:
('1', '2', '3')
('4', '5', '6')
('7', '8', '9')
('10', 'x', 'x')
[iter(iterable)]*n
generates one iterator and iterated n
times in the list. A round-robin of every iterator is then effectively done by izip-longest
; since this is a similar iterator, each such call is advanced, resulting in each such zip-round-robin producing one tuple of n objects.
Split List in Python to Chunks Using the lambda
Function
It is possible to use a basic lambda
function to divide the list into a certain size or smaller chunks. This function works on the original list and N-sized variable, iterate over all the list items and divides it into N-sized chunks.
The complete example code is given below:
test_list = ["1", "2", "3", "4", "5", "6", "7", "8", "9", "10"]
x = 3
def final_list(test_list, x):
return [test_list[i : i + x] for i in range(0, len(test_list), x)]
output = final_list(test_list, x)
print("The Final List is:", output)
Output:
The Final List is: [['1', '2', '3'], ['4', '5', '6'], ['7', '8', '9'], ['10']]
Split List in Python to Chunks Using the lambda
& islice
Method
A lambda
function can be used with the islice
function and produce a generator that iterates over the list. The islice
function creates an iterator that extracts selected items from the iterable. If the start is non-zero, the iterable elements will be skipped before the start is reached. Elements are then returned consecutively unless a step is set higher than one that results in the skipping of items.
The complete example code is given below:
from itertools import islice
test_list = ["1", "2", "3", "4", "5", "6", "7", "8", "9", "10"]
def group_elements(lst, chunk_size):
lst = iter(lst)
return iter(lambda: tuple(islice(lst, chunk_size)), ())
for new_list in group_elements(test_list, 3):
print(new_list)
Output:
('1', '2', '3')
('4', '5', '6')
('7', '8', '9')
('10',)
Split List in Python to Chunks Using the NumPy
Method
The NumPy
library can also be used to divide the list into N-sized chunks. The array_split()
function divides the array into sub-arrays of specific size n
.
The complete example code is given below:
import numpy
n = numpy.arange(11)
final_list = numpy.array_split(n, 4)
print("The Final List is:", final_list)
The arange
function ordered the values according to the given argument and the array_split()
function produces the list/sub-arrays based on the parameter given in it as a parameter.
Output:
The Final List is: [array([0, 1, 2]), array([3, 4, 5]), array([6, 7, 8]), array([ 9, 10])]
Split List Into Chunks in Python Using a User Defined Function
This method will iterate over the list and produces consecutive n-sized chunks where n refers to the number at which a split needs to be implemented. A keyword yield
is used in this function and it allows a function to be halted and restored as the value turns when the execution is suspended. This is the important distinctions from a normal function. A normal function cannot return to where it has left off. The function is called Generator when we use a yield
statement in a function. A generator produces or returns values and can not be named as a simple function, but rather as an iterable, i.e., using a loop.
The complete example code is as follows.
test_list = ["1", "2", "3", "4", "5", "6", "7", "8", "9", "10"]
def split_list(lst, n):
for i in range(0, len(lst), n):
yield lst[i : i + n]
n = 3
output = list(split_list(test_list, n))
print(output)
Output:
[['1', '2', '3'], ['4', '5', '6'], ['7', '8', '9'], ['10']]
Related Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python