How to Check Spelling in Python
-
Spell Checker With the
autocorrect
Library in Python -
Spell Checker With the
pyspellchecker
Library in Python -
Spell Checker With the
textblob
Library in Python
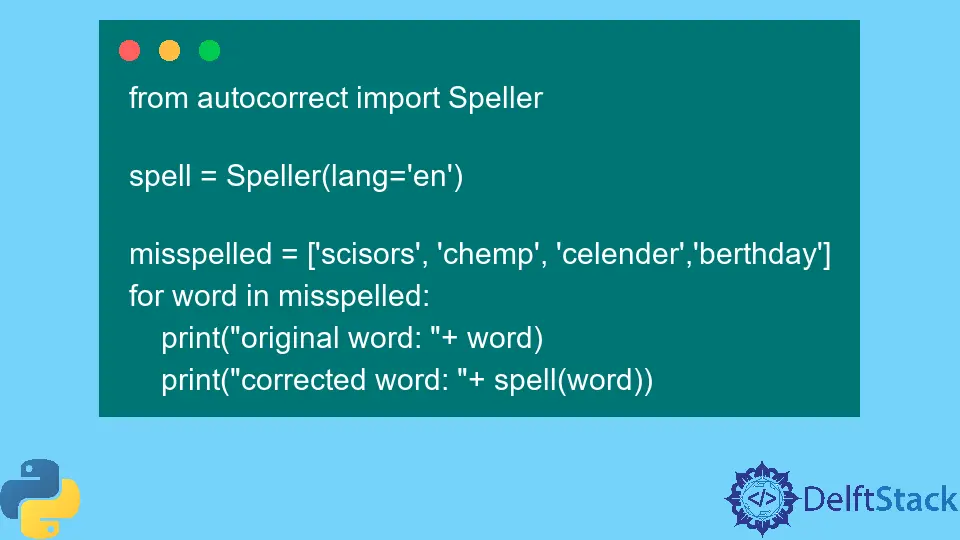
This tutorial will discuss the methods you can use to create a spell checker in Python.
Spell Checker With the autocorrect
Library in Python
The autocorrect
is an external library that can be used to develop a spell checker in Python. Since it is an external library, we have to download and install it before using it in our code. The command to install autocorrect
module is given below.
pip install autocorrect
We can use the Speller
class inside the autocorrect
library and specify the language in the constructor. The following example code shows us how we can create a spell checker with the autocorrect
module.
from autocorrect import Speller
spell = Speller(lang="en")
misspelled = ["scisors", "chemp", "celender", "berthday"]
for word in misspelled:
print("original word: " + word)
print("corrected word: " + spell(word))
Output:
original word: scisors
corrected word: scissors
original word: chemp
corrected word: champ
original word: celender
corrected word: calendar
original word: berthday
corrected word: birthday
In the code above, we developed a spell checker with the Speller
class inside the autocorrect
library. We created an instance spell
of the Speller
class and specified the English language inside the constructor. We passed the misspelled word inside the object just like we do with a normal function, and it returned the corrected word.
Spell Checker With the pyspellchecker
Library in Python
The pyspellchecker
is another external library that can be used in place of the autocorrect
library to develop a spell checker in Python.
Since it is also an external library, we have to download and install it as well to use it in our code. The command to install the pyspellchecker
library is given below.
pip install pyspellchecker
We can use the SpellChecker
class inside the pyspellchecker
library to predict the correct word. The correction()
function inside the SpellChecker
class takes the misspelled word as an input argument and returns the corrected word as a string.
The following program shows us how we can create a spell checker with the pyspellchecker
library.
from spellchecker import SpellChecker
spell = SpellChecker()
misspelled = ["scisors", "chemp", "celender", "berthday"]
for word in misspelled:
print("original word: " + word)
print("corrected word: " + spell.correction(word))
Output:
original word: scisors
corrected word: scissors
original word: chemp
corrected word: cheap
original word: celender
corrected word: calender
original word: berthday
corrected word: birthday
We developed a spell checker with the SpellChecker
class inside the spellchecker
module in the code above. We created an instance spell
of the SpellChecker
class, and the default language is English. We passed the misspelled word inside the correction()
function of the spell
object, returning the corrected word.
Spell Checker With the textblob
Library in Python
To develop a Python spell checker, we can also use the textblob
library. The textblob
is used for processing textual data. It is an external library, and we need to install it with the following command.
pip install textblob
The correct()
function inside the textblob
library return the correction for an incorrect word. The following example program shows us how to create a spell checker program using Python’s textblob
library.
from textblob import TextBlob
misspelled = ["scisors", "chemp", "celender", "berthday"]
for word in misspelled:
print("original word: " + word)
spell = TextBlob(word)
print("corrected word: " + str(spell.correct()))
Output:
original word: scisors
corrected word: scissors
original word: chemp
corrected word: cheap
original word: celender
corrected word: slender
original word: berthday
corrected word: birthday
In the code above, we developed a spell checker with the TextBlob
class inside the textblob
library. We created an instance spell
of the TextBlob
class and passed the word inside the constructor; the default language is English. We then used the correct()
function to display the suitable spelling for that particular word.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn