Correcteur orthographique en Python
-
Correcteur orthographique avec la bibliothèque
autocorrect
en Python -
Correcteur orthographique avec la bibliothèque
pyspellchecker
en Python -
Correcteur orthographique avec la bibliothèque
textblob
en Python
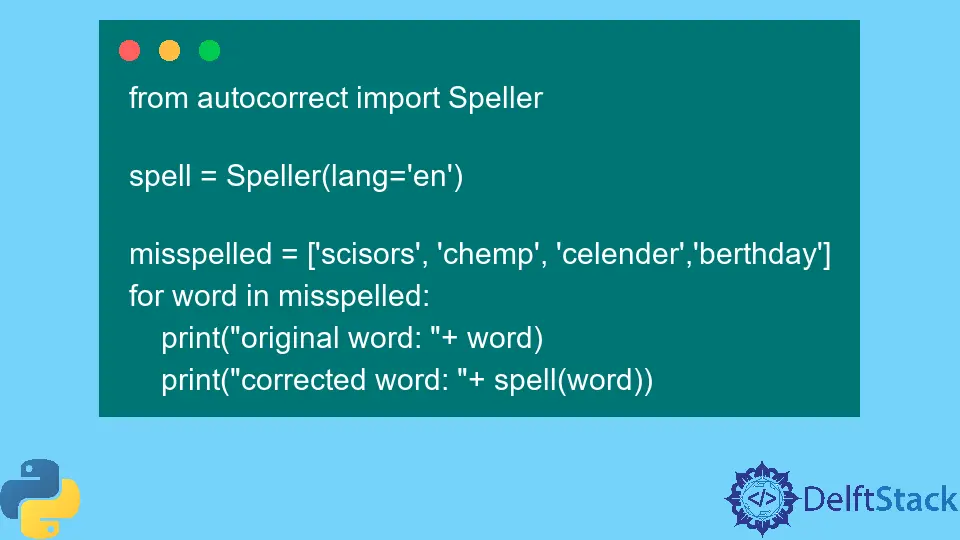
Ce didacticiel abordera les méthodes que vous pouvez utiliser pour créer un correcteur orthographique en Python.
Correcteur orthographique avec la bibliothèque autocorrect
en Python
Le autocorrect
est une bibliothèque externe qui peut être utilisée pour développer un correcteur orthographique en Python. Comme il s’agit d’une bibliothèque externe, nous devons la télécharger et l’installer avant de l’utiliser dans notre code. La commande d’installation du module autocorrect
est donnée ci-dessous.
pip install autocorrect
Nous pouvons utiliser la classe Speller
à l’intérieur de la bibliothèque autocorrect
et spécifier la langue dans le constructeur. L’exemple de code suivant nous montre comment créer un correcteur orthographique avec le module autocorrect
.
from autocorrect import Speller
spell = Speller(lang="en")
misspelled = ["scisors", "chemp", "celender", "berthday"]
for word in misspelled:
print("original word: " + word)
print("corrected word: " + spell(word))
Production:
original word: scisors
corrected word: scissors
original word: chemp
corrected word: champ
original word: celender
corrected word: calendar
original word: berthday
corrected word: birthday
Dans le code ci-dessus, nous avons développé un correcteur orthographique avec la classe Speller
à l’intérieur de la bibliothèque autocorrect
. Nous avons créé une instance spell
de la classe Speller
et spécifié la langue anglaise à l’intérieur du constructeur. Nous avons passé le mot mal orthographié à l’intérieur de l’objet comme nous le faisons avec une fonction normale, et il a renvoyé le mot corrigé.
Correcteur orthographique avec la bibliothèque pyspellchecker
en Python
Le pyspellchecker
est une autre bibliothèque externe qui peut être utilisée à la place de la bibliothèque autocorrect
pour développer un correcteur orthographique en Python.
Comme il s’agit également d’une bibliothèque externe, nous devons également la télécharger et l’installer pour l’utiliser dans notre code. La commande pour installer la bibliothèque pyspellchecker
est donnée ci-dessous.
pip install pyspellchecker
Nous pouvons utiliser la classe SpellChecker
à l’intérieur de la bibliothèque pyspellchecker
pour prédire le mot correct. La fonction correction()
à l’intérieur de la classe SpellChecker
prend le mot mal orthographié comme argument d’entrée et renvoie le mot corrigé sous forme de chaîne.
Le programme suivant nous montre comment créer un correcteur orthographique avec la bibliothèque pyspellchecker
.
from spellchecker import SpellChecker
spell = SpellChecker()
misspelled = ["scisors", "chemp", "celender", "berthday"]
for word in misspelled:
print("original word: " + word)
print("corrected word: " + spell.correction(word))
Production:
original word: scisors
corrected word: scissors
original word: chemp
corrected word: cheap
original word: celender
corrected word: calender
original word: berthday
corrected word: birthday
Nous avons développé un correcteur orthographique avec la classe SpellChecker
à l’intérieur du module spellchecker
dans le code ci-dessus. Nous avons créé une instance spell
de la classe SpellChecker
, et la langue par défaut est l’anglais. Nous avons passé le mot mal orthographié à l’intérieur de la fonction correction()
de l’objet spell
, renvoyant le mot corrigé.
Correcteur orthographique avec la bibliothèque textblob
en Python
Pour développer un correcteur orthographique Python, nous pouvons également utiliser la bibliothèque textblob
. Le textblob
est utilisé pour le traitement de données textuelles. C’est une bibliothèque externe, et nous devons l’installer avec la commande suivante.
pip install textblob
La fonction correct()
à l’intérieur de la bibliothèque textblob
renvoie la correction d’un mot incorrect. L’exemple de programme suivant nous montre comment créer un programme de vérification orthographique à l’aide de la bibliothèque textblob
de Python.
from textblob import TextBlob
misspelled = ["scisors", "chemp", "celender", "berthday"]
for word in misspelled:
print("original word: " + word)
spell = TextBlob(word)
print("corrected word: " + str(spell.correct()))
Production:
original word: scisors
corrected word: scissors
original word: chemp
corrected word: cheap
original word: celender
corrected word: slender
original word: berthday
corrected word: birthday
Dans le code ci-dessus, nous avons développé un correcteur orthographique avec la classe TextBlob
à l’intérieur de la bibliothèque TextBlob
. Nous avons créé une instance spell
de la classe TextBlob
et passé le mot à l’intérieur du constructeur ; la langue par défaut est l’anglais. Nous avons ensuite utilisé la fonction correct()
pour afficher l’orthographe appropriée pour ce mot particulier.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn