Python 中的拼写检查器
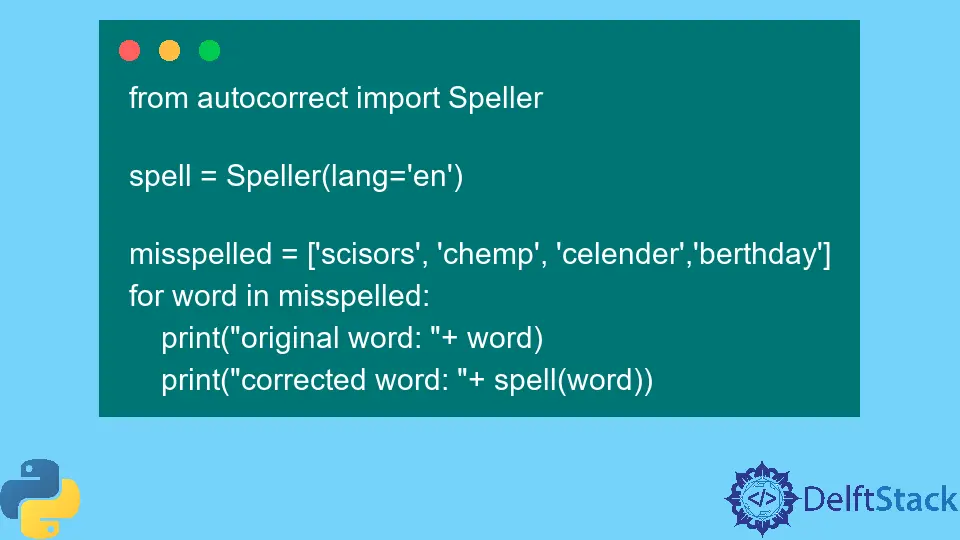
本教程将讨论可用于在 Python 中创建拼写检查器的方法。
带有 autocorrect
库的 Python 拼写检查器
autocorrect
是一个外部库,可用于在 Python 中开发拼写检查器。由于它是一个外部库,我们必须先下载并安装它,然后才能在我们的代码中使用它。下面给出了安装 autocorrect
模块的命令。
pip install autocorrect
我们可以使用 autocorrect
库中的 Speller
类并在构造函数中指定语言。以下示例代码向我们展示了如何使用 autocorrect
模块创建拼写检查器。
from autocorrect import Speller
spell = Speller(lang="en")
misspelled = ["scisors", "chemp", "celender", "berthday"]
for word in misspelled:
print("original word: " + word)
print("corrected word: " + spell(word))
输出:
original word: scisors
corrected word: scissors
original word: chemp
corrected word: champ
original word: celender
corrected word: calendar
original word: berthday
corrected word: birthday
在上面的代码中,我们在 autocorrect
库中开发了一个带有 Speller
类的拼写检查器。我们创建了 Speller
类的实例 spell
,并在构造函数中指定了英语。我们在对象中传递拼写错误的单词,就像我们在普通函数中所做的那样,它返回更正的单词。
使用 Python 中的 pyspellchecker
库的拼写检查器
pyspellchecker
是另一个外部库,可用于代替 autocorrect
库以在 Python 中开发拼写检查器。
由于它也是一个外部库,我们必须下载并安装它才能在我们的代码中使用它。下面给出了安装 pyspellchecker
库的命令。
pip install pyspellchecker
我们可以使用 pyspellchecker
库中的 SpellChecker
类来预测正确的单词。SpellChecker
类中的 correction()
函数将拼写错误的单词作为输入参数,并将正确的单词作为字符串返回。
以下程序向我们展示了如何使用 pyspellchecker
库创建拼写检查器。
from spellchecker import SpellChecker
spell = SpellChecker()
misspelled = ["scisors", "chemp", "celender", "berthday"]
for word in misspelled:
print("original word: " + word)
print("corrected word: " + spell.correction(word))
输出:
original word: scisors
corrected word: scissors
original word: chemp
corrected word: cheap
original word: celender
corrected word: calender
original word: berthday
corrected word: birthday
我们在上面的代码中的 spellchecker
模块中开发了一个带有 SpellChecker
类的拼写检查器。我们创建了一个 SpellChecker
类的实例 spell
,默认语言是英语。我们在 spell
对象的 correction()
函数中传递拼写错误的单词,返回更正后的单词。
Python 中带有 textblob
库的拼写检查器
要开发 Python 拼写检查器,我们还可以使用 textblob
库。textblob
用于处理文本数据。它是一个外部库,我们需要使用以下命令安装它。
pip install textblob
textblob
库中的 correct()
函数返回对错误单词的更正。以下示例程序向我们展示了如何使用 Python 的 textblob
库创建拼写检查程序。
from textblob import TextBlob
misspelled = ["scisors", "chemp", "celender", "berthday"]
for word in misspelled:
print("original word: " + word)
spell = TextBlob(word)
print("corrected word: " + str(spell.correct()))
输出:
original word: scisors
corrected word: scissors
original word: chemp
corrected word: cheap
original word: celender
corrected word: slender
original word: berthday
corrected word: birthday
在上面的代码中,我们使用 textblob
库中的 TextBlob
类开发了一个拼写检查器。我们创建了一个 TextBlob
类的实例 spell
并在构造函数中传递了单词;默认语言是英语。然后我们使用 correct()
函数来显示该特定单词的合适拼写。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn