How to Sort String Alphabetically in Python
-
Alphabetically Sort String With the
sorted()
Function in Python -
Alphabetically Sort String With the
str.join()
Function in Python
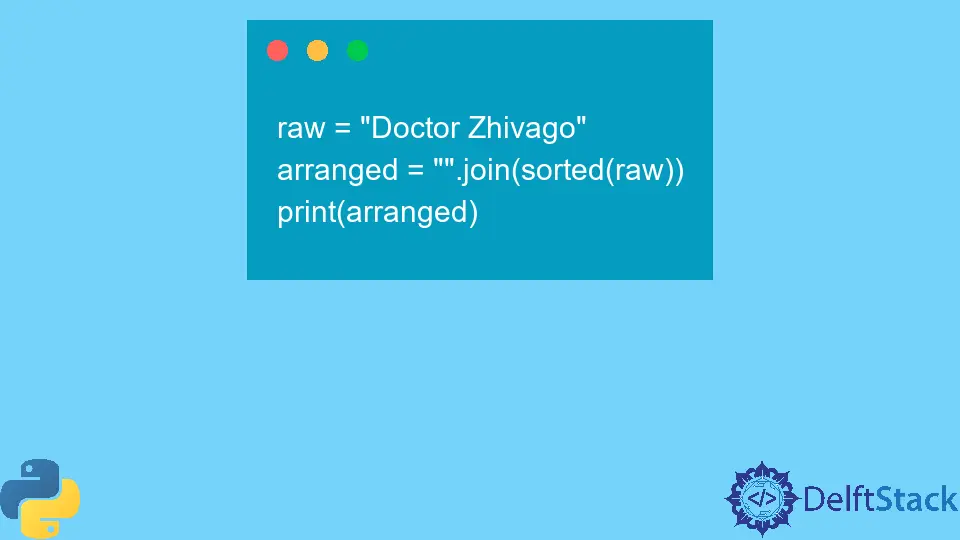
This tutorial will discuss the methods to alphabetically sort a string in Python.
Alphabetically Sort String With the sorted()
Function in Python
The sorted()
function in Python is used to sort iterable objects by the values of their elements. As we already know, the python string is an iterable object. Hence, we can use the sorted()
function to alphabetically sort a string. The sample code below shows us how to alphabetically sort a string in Python.
raw = "Doctor Zhivago"
print(sorted(raw))
Output:
[' ', 'D', 'Z', 'a', 'c', 'g', 'h', 'i', 'o', 'o', 'o', 'r', 't', 'v']
We sorted the raw
string and displayed the output on the console. This approach sorts on the basis of ASCII values of the elements or characters. The only problem with this is approach is that the sorted()
function only returns a list of sorted characters.
Alphabetically Sort String With the str.join()
Function in Python
The previous method works fine, but the only problem with that approach is that the sorted()
function only returns a list of sorted characters. This issue can be resolved with the str.join()
function. The str.join()
function takes an iterable and appends each element at the end of the calling string. The sample code below shows us how to alphabetically sort a string with the str.join()
function in Python.
raw = "Doctor Zhivago"
arranged = "".join(sorted(raw))
print(arranged)
Output:
DZacghiooortv
We sorted the raw
string, stored the results inside the arranged
string, and displayed the arranged
string to the user. We used an empty string with the join()
function to append the sorted characters at the end of an empty string.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn