How to Sort List of Objects in Python
-
Use the
list.sort()
Method to Sort the List of Objects in Python -
Use the
sorted()
Function to Sort the List of Objects in Python
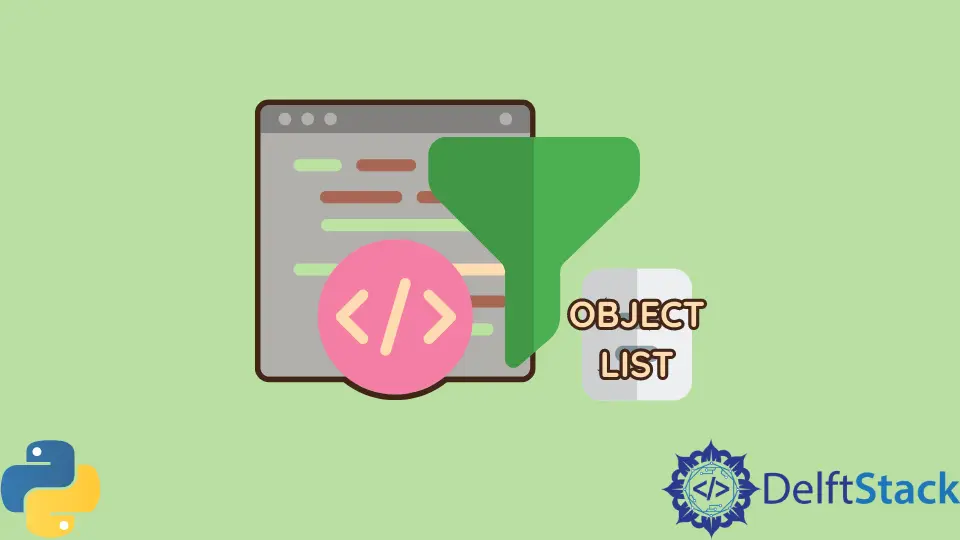
Python Lists are not homogeneous, which makes them different from the other data types provided in Python.
The indexing of a List starts with 0 as the first index and is done sequentially. All the elements in the list have their definite place because of which duplication is allowed in lists.
This tutorial will introduce different methods to sort the list of objects in Python.
Use the list.sort()
Method to Sort the List of Objects in Python
The list.sort()
method sorts all the list elements in ascending or descending order.
It contains two optional parameters, key
and reverse
. The key
parameter, just like its name suggests, serves as a key for the sorting process. The reverse
parameter is used to determine the sorting order. If the value of reverse
is True
, then the sorting occurs in reverse/descending order.
However, the function works just fine without the use of the aforementioned optional parameters.
The following code uses the list.sort()
method to sort the list of objects in Python.
class BankEMP:
def __init__(self, name, dept, age):
self.name = name
self.dept = dept
self.age = age
def __repr__(self):
return "{" + self.name + ", " + self.dept + ", " + str(self.age) + "}"
if __name__ == "__main__":
emps = [
BankEMP("Elijah", "IT", 20),
BankEMP("Nik", "Banking", 21),
BankEMP("Lucien", "Finance", 19),
]
# sort list by `name` in the natural order
emps.sort(key=lambda x: x.name)
print(emps)
# sort list by `name` in reverse order
emps.sort(key=lambda x: x.name, reverse=True)
print(emps)
Output:
[{Elijah, IT, 20}, {Lucien, Finance, 19}, {Nik, Banking, 21}]
[{Nik, Banking, 21}, {Lucien, Finance, 19}, {Elijah, IT, 20}]
To produce the value for the key
parameter, we can use operator.attrgetter()
.
attrgetter()
is imported from the operator
module and is utilized to return a callable object that fetches the attribute from its operand.
The following code uses the list.sort()
method and operator.attrgetter()
to sort the list of objects in Python.
from operator import attrgetter
class BankEMP:
def __init__(self, name, dept, age):
self.name = name
self.dept = dept
self.age = age
def __repr__(self):
return "{" + self.name + ", " + self.dept + ", " + str(self.age) + "}"
if __name__ == "__main__":
emps = [
BankEMP("Elijah", "IT", 20),
BankEMP("Nik", "Banking", 21),
BankEMP("Lucien", "Finance", 19),
]
# sort list by name in the natural order using 'attrgetter()'
emps.sort(key=attrgetter("name"))
print(emps)
Output:
[{Elijah, IT, 20}, {Lucien, Finance, 19}, {Nik, Banking, 21}]
Use the sorted()
Function to Sort the List of Objects in Python
Python provides a built-in sorted()
function that can serve as an alternative to the list.sort()
function.
While list.sort()
does not return any specific value and makes changes to the original list, the sorted()
function returns the sorted list rather than making any modifications to the original list.
The sorted()
function only contains reverse
as an optional parameter.
The following code uses the sorted()
function to sort the list of objects in Python.
class BankEMP:
def __init__(self, name, dept, age):
self.name = name
self.dept = dept
self.age = age
def __repr__(self):
return "{" + self.name + ", " + self.dept + ", " + str(self.age) + "}"
if __name__ == "__main__":
emps = [
BankEMP("Elijah", "IT", 20),
BankEMP("Nik", "Banking", 21),
BankEMP("Lucien", "Finance", 19),
]
# sort list in natural order of name using sorted() function
sort1 = sorted(emps, key=lambda x: x.name)
print(sort1)
Output:
[{Elijah, IT, 20}, {Lucien, Finance, 19}, {Nik, Banking, 21}]
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python