How to Send String Using Python Socket
- Sockets in Python
- Built-in Methods to Achieve Sockets in Python
- Client and Server in Socket Programming
- Send String Using Client-Server Communication in Python Socket
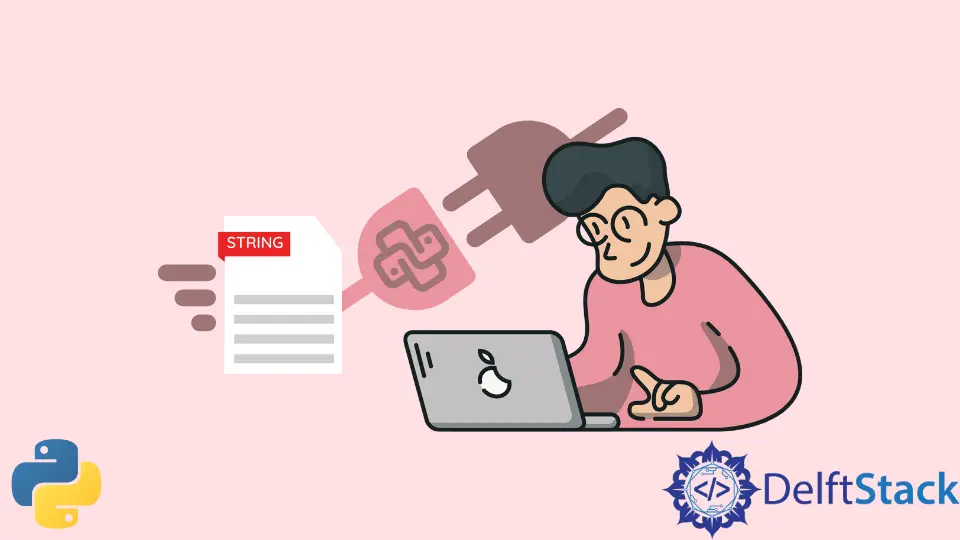
In this article, we will cover the sockets, built-in methods in sockets, the server and client, and how to achieve successful communication with sockets in Python. Sockets play the role of bridge between the server and client sides for receiving and sending data.
In the Python code, we will create sockets, and with the help of sockets, we will pass strings and make the connections between client and server.
Sockets in Python
Sockets define as the endpoints which are built for receiving and sending data. There are two sockets in a single network; the combination of an IP address and a port is present in sockets.
A single device has n number of sockets based on the port numbers. Different types of ports are available for different protocols.
There are following some standard port numbers and their protocols.
Protocol | Description |
---|---|
HTTP |
Its port number is 80, and its Python libraries are httplib , urllib , and xmlrpclib . The HTTP port is used for web pages. |
FTP |
Its port number is 20, and its Python libraries are ftplib and urllib . This port is used for file transfer. |
NNTP |
Its port number is 119, and its Python library is nntplib . This port is used for unsent news. |
SMTP |
Its port number is 25, and its Python library is smtplib . This port is used for sending emails. |
TELNET |
Its port number is 23, and its Python library is telnetlib . This port is used for command lines. |
Pop3 |
Its port number is 110, and its Python library is poplib . This port is used for fetching email. |
Gopher |
Its port number is 70, and its Python library is gopherlib . This port is used for document transfer. |
Built-in Methods to Achieve Sockets in Python
We must import the Socket
module or framework to achieve sockets in Python. All these modules consist of built-in methods, which help in creating sockets and also help to associate with each other.
The following are some robust built-in methods for sockets:
- The
Socket.socket()
method creates sockets. It is required on both sides, like the client and server sides. Socket.accept()
is used to accept the connections. TheSocket.accept()
method returns a pair of values like (conn, address).Socket.bind()
is used to bind addresses specified as a parameter.Socket.close()
represent that socket is closed.Socket.connect()
is used to connect the address specified as a parameter.Socket.listen()
is used to listen to commands in the server or client.
Client and Server in Socket Programming
Below, you will learn what client and server mean in socket programming.
Client
The computer or software that receives information or data from the server is called a client. The client requests services from the server; a web browser is the best example of a client.
Server
A server is a program, computer, or device used to manage network resources. A server may be the same device, local computer, or remote.
Send String Using Client-Server Communication in Python Socket
We will create two files for communication from sockets in Python files - one for the server-side and the other for the client-side. And there will be two programs for connection building.
Server-side in Python Socket
We will create the server-side code first. We will use built-in methods to create the server-side code.
The code for the server-side is the following.
import socket
s = socket.socket(
socket.AF_INET, socket.SOCK_STREAM
) # Socket will create with TCP and IP protocols
# This method will bind the sockets with server and port no
s.bind(("localhost", 9999))
s.listen(1) # Will allow a maximum of one connection to the socket
c, addr = s.accept() # will wait for the client to accept the connection
print("CONNECTION FROM:", str(addr)) # Will display the address of the client
c.send(
b"HELLO, Are you enjoying programming?/Great! Keep going"
) # Will send message to the client after encoding
msg = "Take Care.............."
c.send(msg.encode())
c.close() # Will disconnect from the server
Output:
This connection is from: ('127.0.0.1', 50802)
Client-side in Python Socket
The client will send the message to the server, and the server will respond to that message. The client-side will also use built-in methods in the code.
On the client side, we will create a socket first. Then we will connect the IP address and port number of the host.
Code:
import socket
s = socket.socket(
socket.AF_INET, socket.SOCK_STREAM
) # Socket will create with TCP and, IP protocols
s.connect(("localhost", 9999)) # Will connect with the server
# Will receive the reply message string from the server at 1024 B
msg = s.recv(1024)
while msg:
print("Received:" + msg.decode())
msg = s.recv(1024) # Will run as long as the message string is empty
s.close() # Will disconnect the client
Output:
The message is Revived: HELLO, Are you enjoying programming? Great! Keep going
The message is Revived: Take Care..............
When the given code runs, a connection will be built between the server and the client. And after the connection, the client screen will show a message.
The message will be:
HELLO, Are you enjoying programming? Great! Keep going
And the other message will be:
Take Care..............
This message will be shown on the client-side because it was sent by the server-side. This way, the code will run and give an output of sending strings via sockets in Python.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn