How to Accept Timeout in Python Socket
- Socket Accept, Reject, and Timeout
- Socket Methods and Their Uses in Python
- Example of Socket Accept Timeout in Python
- Set Default Timeout For Each Socket in Python
- Conclusion
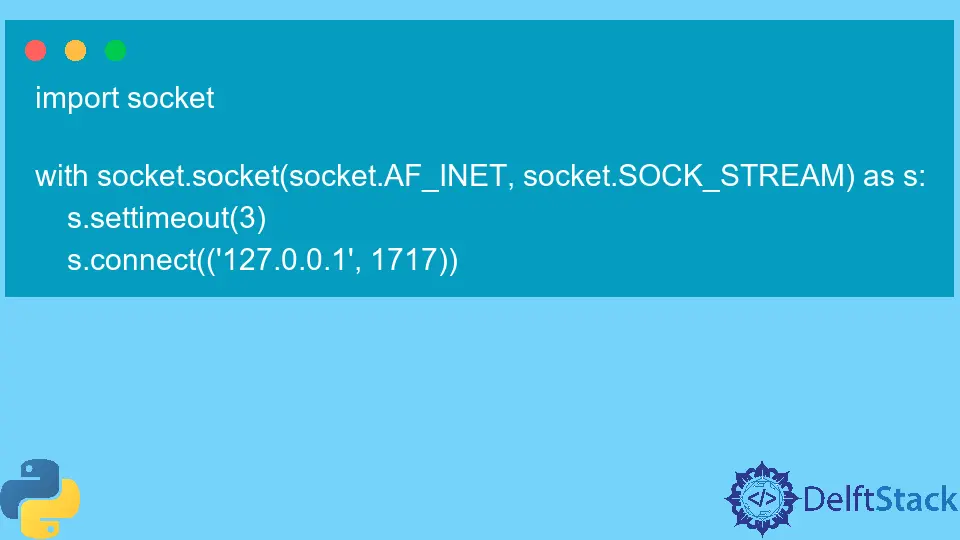
The socket is the basic building block for network communication. A socket is opened whenever two network entities need to transfer the data.
These sockets stay connected during the session. But sometimes, while working with sockets in Python, you may be left waiting for long periods while the other end still accepts the socket connection.
This article discusses the timeout
feature of sockets in Python that is necessary to mitigate the issue of waiting for socket accept
for an infinite time.
Socket Accept, Reject, and Timeout
Whenever you try to establish a connection to a server using Python script, you are required to open a socket connection. This socket connection acts as a tunnel for transferring the data between the client and the server.
In the process of opening the socket, there are three scenarios.
-
Socket Accept
: When the socket is opened successfully, and the server and client are now connected to send and receive data, we term itsocket accept
. This scenario is the final goal of opening a socket. -
Socket reject
: Let us say you opened a socket but passed some different parameters, forgot to pass parameters or the process of opening a socket was not followed correctly; you would get a reject. It means the connection to send and receive data could not be established. -
Socket Accept timeout
: This is an important yet overlooked scenario. Sometimes when you try to open a socket, you may not get any response from the other end.Due to this, you are left waiting forever. This is an unwanted situation as you would like to close the current request and try another request rather than wait forever.
The
timeout
option allows you to set the maximum waiting time to get your request accepted by the other party. After the time limit exceeds, an error is raised.
Socket Methods and Their Uses in Python
Python has an inbuilt library with the name socket
that you need to import to work with socket connections. Let us see some of the important methods of the socket
library.
-
accept()
: As the name suggests, theaccept()
method accepts an incoming socket request from another party. This method returns a socket connection that you can use to transfer the data between the connected entities. -
bind()
: This method binds or attaches a socket connection to an address. This method is a must to be called method if you want to work with sockets.The
bind()
method accepts a tuple of an IP address and a port to which it binds the socket. -
listen()
: This is a server-side method that enables the server to accept a socket to transfer data between the connections. -
connect()
: This method accepts an address as an argument. It then connects the remote socket at the address. -
settimeout()
: This method accepts a non-zero number as the number of seconds to wait before it raises aTimeoutError
. This method is important to mitigate the problem of infinite wait times. -
setdefaulttimeout()
: If you are using multiple socket connections and would like to set the same timeout limit for each connection, then this is the go-to method for your purpose. It accepts the number of seconds as an argument and applies it to all the new socket connections as the wait timeout limit.
Example of Socket Accept Timeout in Python
Let us look at an example of socket accept timeout with the help of code in Python. For this example, we would need to write two Python scripts, one for the server and the other for the client.
We would see three different scenarios, as discussed below.
-
The first scenario is when there is no timeout limit set. In this case, the client would keep on waiting.
Note that, depending on your operating system, the request may be automatically turned down after some time.
-
The second scenario is where we set the timeout limit using the
settimeout()
method. In this case, you will get theTimeoutError
after the set limit. -
In the third case, we will use the
setdefaulttimeout()
method to set the timeout limit.
Let us see the codes of the three cases above one by one.
Socket Accept Timeout in Python When No Timeout Limit Is Set
Server-side script:
import socket
with socket.socket(socket.AF_INET, socket.SOCK_STREAM) as s:
s.bind(("", 1717))
Client-side script:
import socket
with socket.socket(socket.AF_INET, socket.SOCK_STREAM) as s:
s.connect(("127.0.0.1", 1717))
You can see no listen()
method on the server side. Therefore, the server is not responding, and the client is waiting.
Socket Accept Timeout in Python When Timeout Is Set
In this case, you will see that the client aborts the operation and raises a TimeoutError
after the timeout time is passed.
Server-side:
import socket
with socket.socket(socket.AF_INET, socket.SOCK_STREAM) as s:
s.bind(("", 1717))
Client-side:
import socket
with socket.socket(socket.AF_INET, socket.SOCK_STREAM) as s:
s.settimeout(3)
s.connect(("127.0.0.1", 1717))
In this case, a Python socket TimeoutError
is raised. Please note that sockets can behave differently depending on the platform.
On the Linux machine, the socket throws the ConnectionRefusedError: [Errno 111] Connection refused
error until you do not accept the socket on the server side.
Set Default Timeout For Each Socket in Python
This method would set the same default timeout for all your new sockets. In this way, you can save time and hassle by setting the timeout for each socket separately.
Server-side:
import socket
with socket.socket(socket.AF_INET, socket.SOCK_STREAM) as s:
s.bind(("", 1717))
Client-side:
import socket
with socket.socket(socket.AF_INET, socket.SOCK_STREAM) as s:
socket.setdefaulttimeout(3)
s.connect(("127.0.0.1", 1717))
Note that for setdefaulttimeout()
, you must use the socket class directly instead of the object because it sets the timeout for all threads.
Conclusion
The socket timeout is an important aspect of socket programming in Python. If you do not handle the timeout, you may leave your client waiting for the socket forever.
Or in the other case, depending on your environment implementation, it may throw an error.