使用 Python 套接字发送字符串
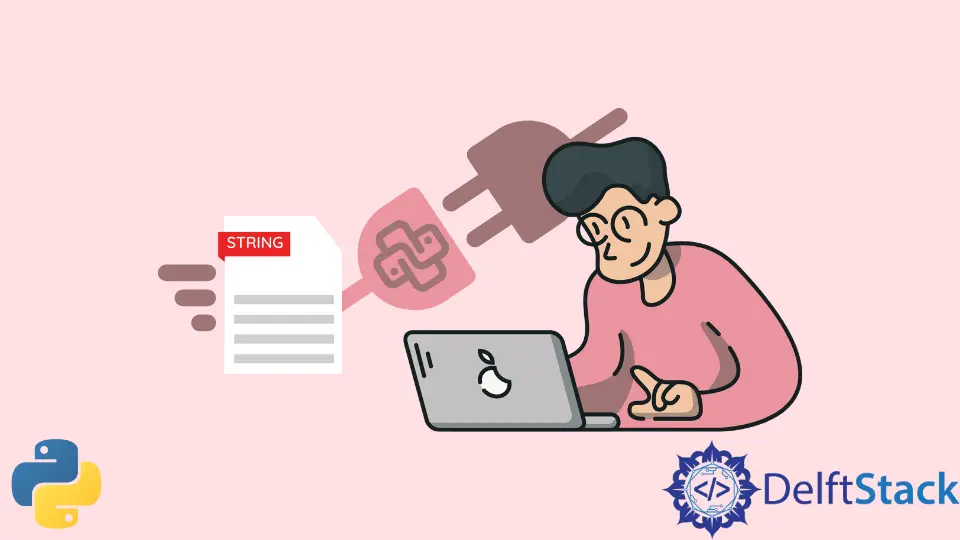
在本文中,我们将介绍套接字、套接字中的内置方法、服务器和客户端,以及如何在 Python 中实现与套接字的成功通信。套接字在服务器端和客户端之间起着桥梁的作用,用于接收和发送数据。
在 Python 代码中,我们将创建套接字,在套接字的帮助下,我们将传递字符串并在客户端和服务器之间建立连接。
Python 中的套接字
套接字定义为为接收和发送数据而构建的端点。单个网络中有两个套接字;套接字中存在 IP 地址和端口的组合。
单个设备有 n 个基于端口号的套接字。不同类型的端口可用于不同的协议。
以下是一些标准端口号及其协议。
协议 | 描述 |
---|---|
HTTP |
它的端口号是 80,它的 Python 库是 httplib 、urllib 和 xmlrpclib 。HTTP 端口用于网页。 |
FTP |
它的端口号是 20,它的 Python 库是 ftplib 和 urllib 。此端口用于文件传输。 |
NNTP |
它的端口号是 119,它的 Python 库是 nntplib 。此端口用于未发送的消息。 |
SMTP |
它的端口号是 25,它的 Python 库是 smtplib 。此端口用于发送电子邮件。 |
TELNET |
它的端口号是 23,它的 Python 库是 telnetlib 。此端口用于命令行。 |
Pop3 |
它的端口号是 110,它的 Python 库是 poplib 。此端口用于获取电子邮件。 |
Gopher |
它的端口号是 70,它的 Python 库是 gopherlib 。此端口用于文件传输。 |
在 Python 中实现套接字的内置方法
我们必须导入 Socket
模块或框架以在 Python 中实现套接字。所有这些模块都包含内置方法,这些方法有助于创建套接字并有助于相互关联。
以下是一些强大的套接字内置方法:
Socket.socket()
方法创建套接字。双方都需要它,例如客户端和服务器端。Socket.accept()
用于接受连接。Socket.accept()
方法返回一对值,例如 (conn, address)。Socket.bind()
用于绑定指定为参数的地址。Socket.close()
表示套接字已关闭。Socket.connect()
用于连接作为参数指定的地址。Socket.listen()
用于侦听服务器或客户端中的命令。
Socket 编程中的客户端和服务器
下面,你将了解套接字编程中客户端和服务器的含义。
客户
从服务器接收信息或数据的计算机或软件称为客户端。客户端向服务器请求服务; Web 浏览器是客户端的最佳示例。
服务器
服务器是用于管理网络资源的程序、计算机或设备。服务器可以是同一设备、本地计算机或远程计算机。
在 Python Socket 中使用客户端-服务器通信发送字符串
我们将创建两个文件用于从 Python 文件中的套接字进行通信——一个用于服务器端,另一个用于客户端。并且将有两个用于建立连接的程序。
Python Socket 中的服务器端
我们将首先创建服务器端代码。我们将使用内置方法来创建服务器端代码。
服务器端的代码如下。
import socket
s = socket.socket(
socket.AF_INET, socket.SOCK_STREAM
) # Socket will create with TCP and IP protocols
# This method will bind the sockets with server and port no
s.bind(("localhost", 9999))
s.listen(1) # Will allow a maximum of one connection to the socket
c, addr = s.accept() # will wait for the client to accept the connection
print("CONNECTION FROM:", str(addr)) # Will display the address of the client
c.send(
b"HELLO, Are you enjoying programming?/Great! Keep going"
) # Will send message to the client after encoding
msg = "Take Care.............."
c.send(msg.encode())
c.close() # Will disconnect from the server
输出:
This connection is from: ('127.0.0.1', 50802)
Python Socket 中的客户端
客户端将消息发送到服务器,服务器将响应该消息。客户端还将在代码中使用内置方法。
在客户端,我们将首先创建一个套接字。然后我们将连接主机的 IP 地址和端口号。
代码:
import socket
s = socket.socket(
socket.AF_INET, socket.SOCK_STREAM
) # Socket will create with TCP and, IP protocols
s.connect(("localhost", 9999)) # Will connect with the server
# Will receive the reply message string from the server at 1024 B
msg = s.recv(1024)
while msg:
print("Received:" + msg.decode())
msg = s.recv(1024) # Will run as long as the message string is empty
s.close() # Will disconnect the client
输出:
The message is Revived: HELLO, Are you enjoying programming? Great! Keep going
The message is Revived: Take Care..............
当给定的代码运行时,将在服务器和客户端之间建立连接。连接后,客户端屏幕将显示一条消息。
消息将是:
HELLO, Are you enjoying programming? Great! Keep going
另一条消息将是:
Take Care..............
此消息将显示在客户端,因为它是由服务器端发送的。这样,代码将运行并给出通过 Python 中的套接字发送字符串的输出。
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn