How to Run Unit Tests in Python
- What is Unit Testing
- Create and Define a Test Case in Python
- Run a Single Test Using Python
- Run Multiple Tests in Python
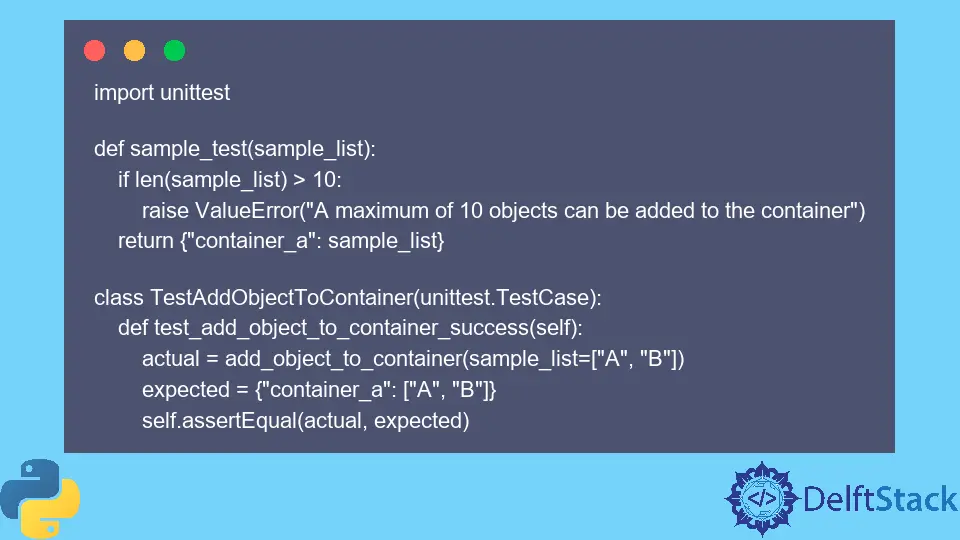
In the software development process known as unit testing, the smaller, independently testable components of an application, known as units, are examined for functionality. The Python unittest
module allows us to run unit tests.
This article will discuss unit testing and how to do it in Python.
What is Unit Testing
Plan, cases and scripting, and the actual unit test are the three stages of a unit test.
The first stage involves writing and reviewing the unit test. The next stage is the production of test cases and scripts.
Create and Define a Test Case in Python
Before starting, keep in mind that we are using Python version 2.7 and up in this article. However, we recommend using the latest Python 3.x version.
A test case is a series of operations on a system to see if it complies with software requirements and operates appropriately. A test case’s objective is to ascertain whether various design features work as anticipated and to ensure the system complies with all applicable standards, recommendations, and client needs.
The act of creating a test case can also aid in identifying flaws or mistakes in the system. In Python, we can use the sub-function TestCase
to create a test case that we will test later on.
But, first, import the unittest
module to make the module available to our code.
Example Code:
import unittest
def sample_test(sample_list):
if len(sample_list) > 10:
raise ValueError("A maximum of 10 objects can be added to the container")
return {"container_a": sample_list}
class TestAddObjectToContainer(unittest.TestCase):
def test_add_object_to_container_success(self):
actual = add_object_to_container(sample_list=["A", "B"])
expected = {"container_a": ["A", "B"]}
self.assertEqual(actual, expected)
Run a Single Test Using Python
For instance, to test our developed test case, we can run the command below once the necessary setup has been made.
Example Code:
python -m unittest main.py
When we execute this command, we get the following output.
Or something like this, if there is an error on our test.
Run Multiple Tests in Python
For example, if we have created multiple Python files per test and placed them under one directory, we can run them together with the discover
method. To auto-detect the test files, we need to name them starting with the keyword test
like our previously created Python file main.py
.
python -m unittest discover
The command above will detect all the files whose names start with test
and execute them.
Marion specializes in anything Microsoft-related and always tries to work and apply code in an IT infrastructure.
LinkedIn