How to Replace Multiple Characters in a String in Python
-
Use
str.replace()
to Replace Multiple Characters in Python -
Use
re.sub()
orre.subn()
to Replace Multiple Characters in Python -
Use
translate()
andmaketrans()
to Replace Multiple Characters in Python - Conclusion
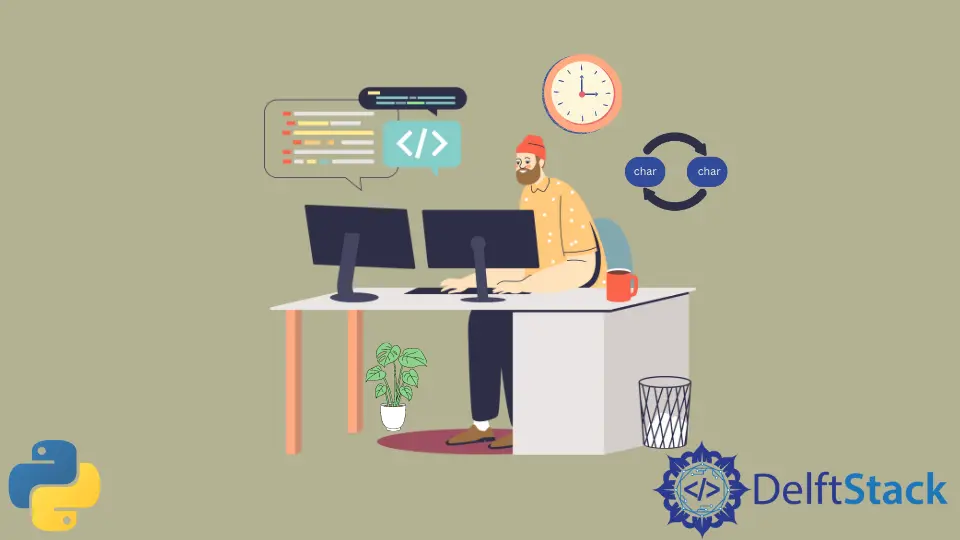
Text manipulation is a common task in programming, and replacing multiple characters within a string is a frequent requirement. In Python, there are various techniques to achieve this goal, each with its own strengths and use cases.
This article shows you how to replace multiple characters in a string in Python. Let’s dive into each approach and understand how they work to provide a versatile set of tools for string manipulation in Python.
Use str.replace()
to Replace Multiple Characters in Python
We can use the replace()
method of the str
data type to replace substrings with a different output.
replace()
accepts two parameters: the first parameter is the regex pattern you want to match strings with, and the second parameter is the replacement string for the matched strings.
Let’s consider a scenario where we want to replace specific characters in a string with predefined replacements. Here’s a concise Python script using the str.replace
method:
def replace_multiple_chars(input_string, replace_dict):
for old_char, new_char in replace_dict.items():
input_string = input_string.replace(old_char, new_char)
return input_string
# Example usage:
original_string = "Hello, World!"
replacement_dict = {"H": "h", "o": "0", "l": "1", "d": "!"}
result = replace_multiple_chars(original_string, replacement_dict)
print(result)
We start by defining a function replace_multiple_chars
that takes an input string and a dictionary (replace_dict
) containing the characters to be replaced as keys and their corresponding replacements as values.
The function then iterates through the key-value pairs in the replace_dict
using a for
loop.
For each pair, the str.replace
method is called on the input_string
. This method searches for occurrences of the old_char
in the string and replaces them with the new_char
.
The modified string is assigned back to input_string
within the loop, allowing multiple replacements to be performed sequentially.
Finally, the modified string is returned from the function.
We demonstrate the function by defining an original_string
and a replacement_dict
. In this case, we want to replace H
with h
, o
with 0
, l
with 1
, and d
with !
.
The result of the function call is printed, showing the string after the specified replacements.
Output:
he110, W0r1!
Use re.sub()
or re.subn()
to Replace Multiple Characters in Python
In Python, you can import the re
module, which has an amount of expression matching operations for regex for you to utilize.
Two of such functions within re
are sub()
and subn()
.
re.sub()
to Replace Multiple Characters in Python
The re.sub
method is a part of Python’s regular expression module (re
). This method provides a robust way to replace multiple characters in a string based on patterns defined by regular expressions.
Consider a scenario where we want to replace specific characters in a string based on a predefined mapping. Below is a Python script utilizing the re.sub
method:
import re
def replace_multiple_chars_regex(input_string, replace_dict):
def replace(match):
return replace_dict[match.group(0)]
pattern = re.compile("|".join(map(re.escape, replace_dict.keys())))
return pattern.sub(replace, input_string)
# Example usage:
original_string = "Hello, World!"
replacement_dict = {"H": "h", "o": "0", "l": "1", "d": "!"}
result = replace_multiple_chars_regex(original_string, replacement_dict)
print(result)
Begin by importing the re
module, which provides support for regular expressions in Python.
Define a function replace_multiple_chars_regex
that takes an input string and a dictionary (replace_dict
) specifying the characters to be replaced and their corresponding replacements.
Inside the function, define a nested function called replace
. This function takes a match object (generated by re.sub
) and returns the replacement for the matched pattern.
Use re.compile
to create a regular expression pattern. The pattern is constructed by joining the keys of replace_dict
using the |
(pipe) character, which acts as a logical OR
in regular expressions.
Apply the re.sub
method to the input string (input_string
). This method searches for occurrences of the pattern in the string and replaces them with the result of the replace
function.
The modified string is returned from the function.
Demonstrate the function by defining an original_string
and a replacement_dict
. In this example, we want to replace H
with h
, o
with 0
, l
with 1
, and d
with !
.
Print the result of the function call, showing the string after the specified replacements.
Output:
he110, W0r1!
re.subn()
to Replace Multiple Characters in Python
Unlike re.sub
, re.subn
not only replaces matched patterns but also returns the count of substitutions made. In this section, we’ll explore the application of re.subn
to efficiently replace multiple characters in a string, providing both the modified string and the count of substitutions.
Let’s consider a scenario where we want to replace specific characters in a string based on a predefined mapping. Below is a Python script utilizing the re.subn
method:
import re
def replace_multiple_chars_subn(input_string, replace_dict):
def replace(match):
return replace_dict[match.group(0)]
pattern = re.compile("|".join(map(re.escape, replace_dict.keys())))
result, count = pattern.subn(replace, input_string)
return result, count
# Example usage:
original_string = "Hello, World!"
replacement_dict = {"H": "h", "o": "0", "l": "1", "d": "!"}
result, count = replace_multiple_chars_subn(original_string, replacement_dict)
print(result)
print(f"Number of substitutions: {count}")
Start by importing the re
module, which provides support for regular expressions in Python.
Define a function replace_multiple_chars_subn
that takes an input string and a dictionary (replace_dict
) specifying the characters to be replaced and their corresponding replacements.
Inside the function, define a nested function called replace
. This function takes a match object (generated by re.subn
) and returns the replacement for the matched pattern.
Use re.compile
to create a regular expression pattern. The pattern is constructed by joining the keys of replace_dict
using the |
(pipe) character, which acts as a logical OR
in regular expressions.
Apply the re.subn
method to the input string (input_string
). This method searches for occurrences of the pattern in the string and replaces them with the result of the replace
function.
It returns a tuple containing the modified string and the count of substitutions.
The modified string and the count of substitutions are returned from the function.
Demonstrate the function by defining an original_string
and a replacement_dict
. In this example, we want to replace H
with h
, o
with 0
, l
with 1
, and d
with !
.
Print the modified string and the count of substitutions. This provides insight into how many replacements were made.
Output:
he110, W0r1!
Number of substitutions: 4
Use translate()
and maketrans()
to Replace Multiple Characters in Python
Python provides a powerful duo for this task: str.maketrans
and str.translate
. These methods work together to create a translation table, simplifying the process of replacing characters in a string.
In this section, we will explore the usage of str.maketrans
and str.translate
and understand how they can be harnessed to streamline multiple character replacements.
Consider a scenario where we want to replace specific characters in a string with predefined mappings. Below is a Python script utilizing str.maketrans
and str.translate
:
def replace_multiple_chars(input_string, replace_dict):
translation_table = str.maketrans(replace_dict)
result = input_string.translate(translation_table)
return result
# Example usage:
original_string = "Hello, World!"
replacement_dict = {"H": "h", "o": "0", "l": "1", "d": "!"}
result = replace_multiple_chars(original_string, replacement_dict)
print(result)
Begin by defining a function named replace_multiple_chars
that takes an input string and a dictionary (replace_dict
), specifying the characters to be replaced and their corresponding replacements.
Use str.maketrans
to create a translation table based on the provided replace_dict
. This method generates a mapping of Unicode ordinals for each character to be replaced and their corresponding replacements.
The modified string is stored in the variable result
and returned from the function.
Use the translate
method on the input string, passing the translation table as an argument. This method replaces characters in the string based on the translation table.
Demonstrate the function by defining an original_string
and a replacement_dict
. In this example, we want to replace H
with h
, o
with 0
, l
with 1
, and d
with !
.
Print the result of the function call, showing the string after the specified replacements.
Output:
he110, W0r1!
Conclusion
In this article, we explored different methods to replace multiple characters in a string in Python. We began by leveraging the str.replace()
method, a simple and effective way to replace substrings within a string.
Next, we delved into the power of regular expressions using the re.sub()
and re.subn()
methods. These methods provide a more sophisticated approach, enabling us to replace characters based on regex patterns. re.sub()
replaces all occurrences, while re.subn()
not only replaces but also provides the count of substitutions made.
Finally, we introduced the combination of str.maketrans
and str.translate
as a powerful duo for multiple character replacements. The str.maketrans
method efficiently generates a translation table, and str.translate
applies this table to replace characters in the string.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn