How to Repeat String N Times in Python
-
Repeat String N Times With the
*
Operator in Python - Repeat String to a Length With a User-Defined Function in Python
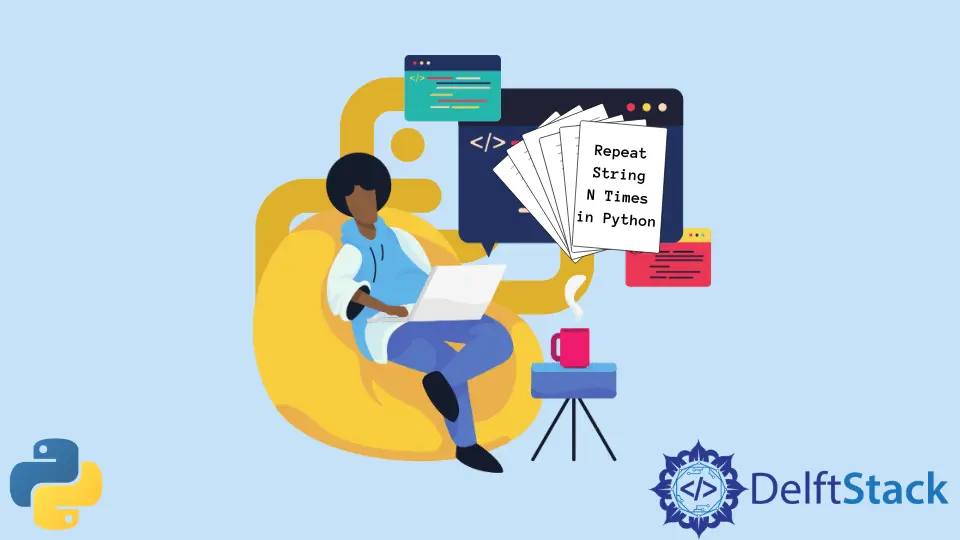
In this tutorial, we will learn the methods to repeat a string n times in Python.
Repeat String N Times With the *
Operator in Python
In python, it is very straightforward to repeat a string as many times as we want. We have to use the *
operator and specify the number of times we want to repeat the whole string. The code example below shows how to use the *
operator to repeat a string n times.
text = "txt"
repeated = text * 4
print(repeated)
Output:
txttxttxttxt
In the code above, we created a string variable text
, repeated it 4
times, and stored the repeated string inside the new string variable repeated
. In the end, we displayed the value of the repeated
variable to the user.
This method is convenient when we want to repeat the entire string n
times, as shown in the output txttxttxttxt
. But if we’re going to repeat a string to a certain length, we have to write our implementation. For example, if the specified length was 10
characters, the above string would look like txttxttxtt
.
Repeat String to a Length With a User-Defined Function in Python
The previous method fails if we want to repeat a string but also stay inside a character limit. In python, there is no built-in method for it, so we have to implement our own logic inside a function. The code example below shows how to repeat a string to a certain length with a user-defined function.
def repeat(string_to_repeat, length):
multiple = int(length / len(string_to_repeat) + 1)
repeated_string = string_to_repeat * multiple
return repeated_string[:length]
r = repeat("txt", 10)
print(r)
Output:
txttxttxtt
This time, we have repeated the string txt
to length 10
. We wrote the repeat()
function that takes the original string string_to_repeat
and the length of the repeated string length
as input parameters. We then initialized the multiple
integer variable that determines how many times the original string needs to be repeated to fit the length limit. This can be determined by dividing the length
parameter by the actual length of the string_to_repeat
parameter. We added 1
to compensate for the lost values after the decimal point. We then stored a repeating string inside the repeated_string
variable by multiplying string_to_repeat
with the multiple
variable. In the end, we returned the values inside the repeated_string
from 0
to the length
index.
We used the repeat()
function to repeat the string txt
to the length 10
and displayed the output. The output shows txttxttxtt
, which is what we discussed in the previous section.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn