How to Repeat Code N Times in Python
-
How to Repeat
N
Times in Python Using afor
Loop With therange()
Function -
How to Repeat
N
Times in Python Using awhile
Loop -
How to Repeat
N
Times in Python Using theitertools.repeat()
Function -
How to Repeat
N
Times in Python Using List Comprehension -
How to Repeat
N
Times in Python Using Recursion -
How to Repeat
N
Times in Python Conclusion
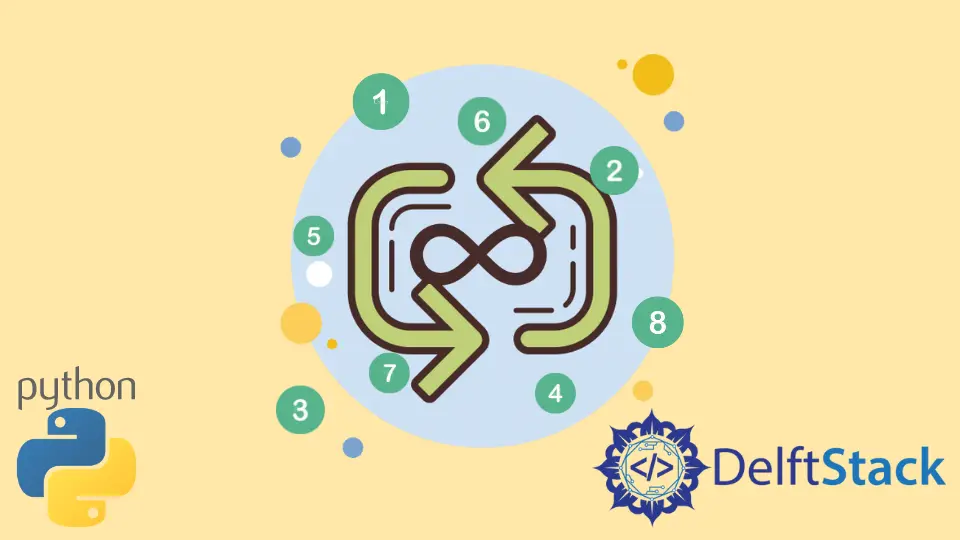
In Python programming, mastering the art of repetition is crucial for optimizing code efficiency and automating tasks. Understanding how to repeat a specific operation N times allows you to streamline workflows and enhance productivity.
This guide explores various methods, from classic for
loops with the range()
function to dynamic while
loops, list comprehensions, itertools
functions, and recursive approaches.
Whether iterating over a sequence, executing a block of code, or creating repetitive data structures, this article equips you with the knowledge to choose the most suitable technique for your needs.
How to Repeat N
Times in Python Using a for
Loop With the range()
Function
The most common method to repeat a specific task or operation N
times is using the for
loop. We can iterate the code lines N
times using the for
loop with the range()
function in Python.
Syntax of the range()
Function
range(start, stop, step)
The range()
function takes three parameters:
start
(optional): The starting value of the sequence. If not specified, the default is0
.stop
: The end value of the sequence. The generated sequence will not include this value.step
(optional): The step or increment between each number in the sequence. If not specified, the default is1
.
Python Repeat N
Times Using for
Loop With range()
Example
# Number of times to repeat the code
N = 5
# Code block to repeat a string n times
for _ in range(N):
# Your code here
print("Code block executed")
In the above code example, we first define the value of N
, which represents the number of times you want to repeat the code. You can change this value to any positive integer.
Then, we create a for
loop that iterates over a range of numbers from 0
to N-1
.
In Python, range(N)
generates numbers from 0
to N-1
. _
is used as a throwaway variable in the loop.
You can place the code block inside the loop that you want to repeat N
times.
In this example, we’ve used a simple print()
statement for demonstration purposes. Replace it with your actual code.
Code Output:
This output is generated by the print("Code block executed")
statement inside the for
loop, which is repeated 5 times due to the specified value of N
(which is 5
in this case).
How to Repeat N
Times in Python Using a while
Loop
Another way to repeat code N
times is using a while
loop.
A while
loop is a control flow statement that repeatedly executes a block of code as long as a specified condition remains true. The loop continues to execute as long as the condition evaluates to True
.
If at any point the condition becomes False
, the loop is exited, and the program continues with the next statement after the loop. This type of loop is suitable for situations where the specific number of iterations is not known beforehand or where the loop should continue until a certain condition is met.
To repeat a block of code N
times in Python using a while
loop, you can set up a condition based on the variable N
and execute the code block until the condition is met.
Python Repeat N
Times Using while
Loop Example
# Number of times to repeat the code
N = 5
count = 0
while count < N:
# Your code here
print("Code block executed")
count += 1
In the above code example, we set the value of N
to the desired number of repetitions. Then, we initialize a count
variable to keep track of the number of iterations.
We then use a while
loop with the condition count < N
to repeatedly execute the code block if the count is less than N
.
Inside the loop, we place the code block we want to repeat. Next, we increment the count
variable by 1
in each iteration to keep track of the number of repetitions.
Code Output:
This output is the result of a while
loop that iterates as long as the count
variable is less than N
(which is 5
).
Inside the loop, the message Code block executed
is printed to the console, and the count
variable is incremented by 1
in each iteration.
The loop continues until count
reaches the value of N
.
How to Repeat N
Times in Python Using the itertools.repeat()
Function
Python’s itertools
module provides another convenient function called repeat()
to create an iterator that repeats elements from an iterable indefinitely.
Syntax of the itertools.repeat()
Function
itertools.repeat(element, times)
This function takes two parameters:
element
: The value that will be repeated in the iterator.times
(optional): The number of times theelement
will be repeated. If not specified, the iterator will continue indefinitely.
As we want to repeat the iteration N
times, we will pass the value of N
to the times
argument and the None
value to the element
argument since we do not need to print anything.
The repeat()
function is more efficient than the range()
function, but the itertools
module needs to be imported to use this method.
Python Repeat N
Times Using itertools.repeat()
Example
from itertools import repeat
# Number of times the string appears
N = 5
# Your code here, enclosed in the repeat() function
for _ in repeat(None, N):
print("Code block executed")
First, we import the repeat()
function from the itertools
module and set the value of N
to indicate how many times we want to repeat the code.
We then use a for
loop with repeat(None, N)
to execute the code block N
times. The None
argument in repeat()
is a placeholder, as the function is primarily designed for repeating elements.
Inside the loop, we place the code block we want to repeat. We’ve used a print()
statement in this example.
Code Output:
This output is a result of the print("Code block executed")
statement inside the loop, which is repeated five times due to the use of the repeat()
function in the for
loop header.
Use Advanced Techniques
Beyond repeat()
, itertools
offers various functions like cycle()
, chain()
, and accumulate()
, each catering to specific iteration needs.
For instance, cycle()
endlessly repeats elements from an iterable, while chain()
seamlessly combines multiple iterables into a single stream. accumulate()
is particularly useful for obtaining cumulative results.
from itertools import cycle, chain, accumulate
print("Example of using cycle:")
for i, element in enumerate(cycle([1, 2, 3])):
if i >= 10: # break after 10 iterations
break
print(f"Iteration {i + 1}: Your task with repeated element {element}")
print("\nExample of using chain:")
for i, combined_element in enumerate(chain([1, 2, 3], ["a", "b", "c"])):
if i >= 6: # break after 6 iterations
break
print(f"Iteration {i + 1}: Your task with combined element {combined_element}")
print("\nExample of using accumulate:")
input_list = [1, 2, 3, 4]
result = list(accumulate(input_list))
print(f"Original List: {input_list}")
print(f"Accumulated Result: {result}")
Code Output:
This code demonstrates the use of cycle()
to repeat elements from [1, 2, 3]
, chain()
to combine elements from [1, 2, 3]
and ['a', 'b', 'c']
, and accumulate()
to calculate the cumulative sum of elements in [1, 2, 3, 4]
.
The enumerate()
function is used to limit the number of iterations for demonstration purposes. Adjust the loop conditions accordingly for your specific use case.
How to Repeat N
Times in Python Using List Comprehension
You can also use list comprehension if you want to repeat the code to create a list of results.
To repeat a specific operation N
times in Python using list comprehension, you can create lists by applying the same expression or operation for each element in the range from 0
to N-1
.
This concise approach allows you to generate a list of results based on a repeated pattern, providing a compact and readable way to express repetitive actions. List comprehension is a powerful and Pythonic way to achieve repetition while maintaining code clarity and brevity.
Python Repeat N
Times Using List Comprehension Example
# Number of times to repeat the code
N = 5
# Your code here, enclosed in a list comprehension
results = ["Code block executed" for _ in range(N)]
print(results)
In the code above, we first define the value of N
to specify how often we want to repeat the code.
The list comprehension ["Code block executed" for _ in range(N)]
creates a list containing the string value Code block executed
repeated N
times. The loop variable _
is used when you don’t need to use its value inside the loop.
The range(N)
generates a sequence of numbers from 0
to N-1
, and for each of these values, the string value in the specified expression (Code block executed
) is appended to the list.
The resulting list, results
, contains the repeated string, and when printed, it displays the repetition as indicated.
Code Output:
The output of the provided code is a list containing the string value Code block executed
repeated 5 times.
This output reflects the result of the list comprehension, which repeats the specified string N
times (in this case, N
is equal to 5
) and collects these repetitions into a list.
How to Repeat N
Times in Python Using Recursion
You can also achieve code repetition using recursion. To repeat a process N
times in Python using recursion, you create a function that calls itself, decrementing N
with each recursive call until reaching the base case.
This approach enables repetitive execution through a series of function calls, making it a flexible and dynamic way to handle iterations.
Recursion in Python allows for concise code while providing a clear structure for repeating actions.
Python Repeat N
Times Using Recursion Example
# Number of times to repeat the code
N = 5
def repeat_code(count):
if count < N:
# Your code here
print("Code block executed")
repeat_code(count + 1)
# Start the repetition
repeat_code(0)
First, we define the value of N
to specify the desired number of repetitions.
We then create a function called repeat_code
that takes a count
argument as input string. This function will be responsible for executing the code block.
Inside the repeat_code
function, we use a base case to stop the recursion when the count
reaches N
. In each recursive call, we execute the code block and increment the count
by 1
.
To start the repetition, we call the repeat_code(0)
function with an initial count of 0
.
Code Output:
This output is the result of the recursive function repeat_code
, which prints Code block executed
and calls itself until the count reaches the specified value of N
(which is 5
).
Each recursive call prints a repeat string of the message, leading to a total of 5 repetitions.
How to Repeat N
Times in Python Conclusion
In this article, we’ve explored five methods to repeat a string of code N
times in Python: using for
loops, while
loops, the itertools.repeat()
function, list comprehension, and recursion. Each method has its use cases and advantages, so choose the one that best fits your requirements and coding style.
Whether you’re iterating through sequences or simulating scenarios, these methods allow you to repeat code efficiently in your Python programs.
To further improve your Python skills, consider exploring Python generators for efficient iteration as your next learning step. Dive into topics like generator expressions and the yield
keyword to enhance your skills in handling large datasets and building scalable, resource-friendly Python programs.