How to Remove Quotes From String in Python
-
Remove Quotes From a String in Python Using the
replace()
Method -
Remove Quotes From a String in Python Using the
strip()
Method -
Remove Quotes From a String in Python Using the
lstrip()
Method -
Remove Quotes From a String in Python Using the
rstrip()
Method -
Remove Quotes From a String in Python Using the
eval()
Method - Remove Quotes From a String in Python Using String Slicing
- Remove Quotes From a String in Python Using Regular Expressions
-
Remove Quotes From a String in Python Using a
for
Loop Withnot in
-
Remove Quotes From a String in Python Using the
join()
Function Withnot in
-
Remove Quotes From a String in Python Using the
startswith()
Function With Slicing -
Remove Quotes From a String in Python Using the
endswith()
Function with Slicing -
Remove Quotes From a String in Python Using the
ast.literal_eval()
Function - Conclusion
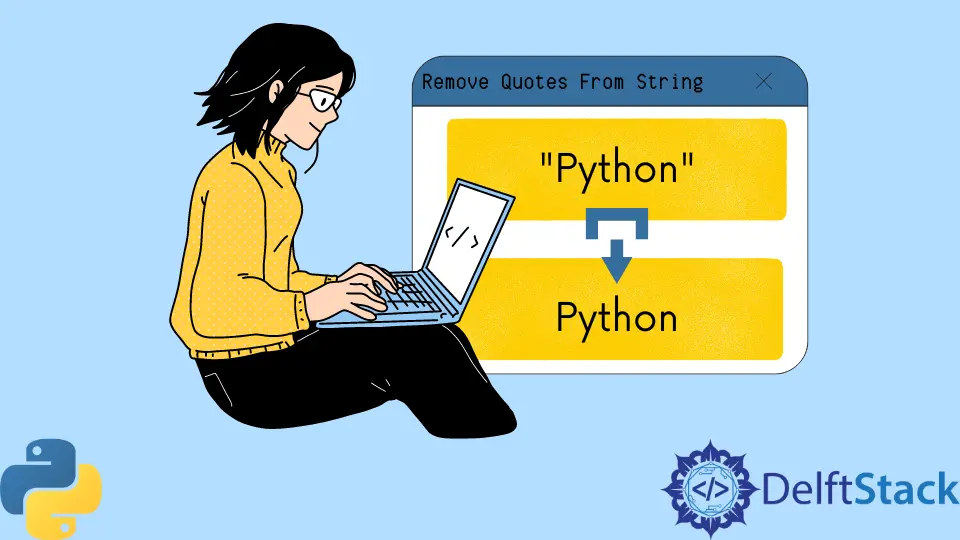
Working with strings in Python often involves dealing with quotes, and there are various scenarios where you may want to remove them. Whether you’re processing user input, manipulating data, or evaluating expressions, the ability to cleanly remove quotes is a valuable skill.
In this article, we will explore different methods to achieve this task.
Remove Quotes From a String in Python Using the replace()
Method
In Python, strings are often enclosed within single (''
) or double (""
) quotes. If you want to remove these quotes from a string, the replace()
method provides a simple and effective solution.
This method allows you to replace occurrences of a specified substring with another substring, making it ideal for eliminating quotes from a string.
The syntax for the replace()
method is as follows:
new_string = original_string.replace(old, new)
Where:
original_string
: The string from which you want to remove quotes.old
: The substring you want to replace (in this case, the quotes).new
: The substring to replace the old substring with (an empty string to remove quotes).
By calling replace()
on a string and specifying the quotes ('"'
) as the old substring and an empty string (""
) as the new substring, all instances of quotes in the original string will be replaced, effectively removing them.
Let’s explore the replace()
method with a practical example:
original_string = '"Python is versatile and powerful."'
no_quotes_string = original_string.replace('"', "")
print("Original string: {}".format(original_string))
print("String without quotes: {}".format(no_quotes_string))
In this example, we start with a string original_string
containing some quotes. The replace()
method is then called on this string, with '"'
as the old substring and ""
(an empty string) as the new substring.
This effectively replaces all instances of quotes in the original string with an empty string.
After the replacement, we print both the original string and the modified string without quotes using formatted strings. The result is displayed, showcasing the string manipulation achieved using the replace()
method.
Output:
Original string: "Python is versatile and powerful."
String without quotes: Python is versatile and powerful.
Remove Quotes From a String in Python Using the strip()
Method
Another approach to removing quotes from a string in Python involves the use of the strip()
method. This method is particularly useful when you want to eliminate quotes from both ends of a string.
It allows you to specify the characters that need to be removed from the beginning and end of the string, making it suitable for handling quotes.
The syntax for the strip()
method is as follows:
new_string = original_string.strip(characters)
Where:
original_string
: The string from which you want to remove quotes.characters
: A string specifying the characters to be removed (in this case, quotes).
By calling strip()
on a string and passing '"'
as the characters to be removed, the method trims the quotes from both ends of the string, leaving the content intact.
Let’s dive into a practical example of using the strip()
method:
original_string = '"Python makes programming enjoyable."'
no_quotes_string = original_string.strip('"')
print("Original string: {}".format(original_string))
print("String without quotes: {}".format(no_quotes_string))
In this example, the strip()
method is applied to the string original_string
, with '"'
specified as the characters to be removed. As a result, any leading and trailing quotes are removed from the original string, and the modified string without quotes is stored in no_quotes_string
.
After the manipulation, we print both the original string and the string without quotes using formatted strings. This showcases the effectiveness of the strip()
method in removing quotes from the specified string.
Output:
Original string: "Python makes programming enjoyable."
String without quotes: Python makes programming enjoyable.
Remove Quotes From a String in Python Using the lstrip()
Method
When you specifically want to remove quotes only from the beginning (left side) of a string, the lstrip()
method comes in handy. This method trims characters from the left side of the string and is particularly useful when you want to eliminate leading quotes.
The syntax for the lstrip()
method is as follows:
new_string = original_string.lstrip(characters)
Where:
original_string
: The string from which you want to remove quotes.characters
: A string specifying the characters to be removed (in this case, quotes).
By calling lstrip()
on a string and passing '"'
as the characters to be removed, the method trims leading quotes from the left side of the string, leaving the rest of the content untouched.
Take a look at an example showing the use of the lstrip()
method to remove quotes:
original_string = '"Pythonistas are passionate about coding.'
no_quotes_string = original_string.lstrip('"')
print("Original string: {}".format(original_string))
print("String without leading quotes: {}".format(no_quotes_string))
In this example, we initialize a string original_string
that starts with a leading quote. The lstrip()
method is then applied to this string, with '"'
specified as the characters to be removed.
As a result, only the leading quotes on the left side are removed, leaving the remaining content intact. This highlights how the lstrip()
method effectively trims quotes from the beginning of the specified string.
Output:
Original string: "Pythonistas are passionate about coding."
String without leading quotes: Pythonistas are passionate about coding.
Remove Quotes From a String in Python Using the rstrip()
Method
In Python, when the objective is to remove quotes exclusively from the end (right side) of a string, the rstrip()
method proves to be a valuable tool. This method trims characters from the right side of the string and is particularly useful when you want to eliminate trailing quotes.
The syntax for the rstrip()
method is as follows:
new_string = original_string.rstrip(characters)
Where:
original_string
: The string from which you want to remove quotes.characters
: A string specifying the characters to be removed (in this case, quotes).
By calling rstrip()
on a string and passing '"'
as the characters to be removed, the method trims trailing quotes from the right side of the string, preserving the content preceding the quotes.
Let’s delve into an example illustrating the use of the rstrip()
method to remove quotes:
original_string = 'Pythonistas are excited about coding."'
no_quotes_string = original_string.rstrip('"')
print("Original string: {}".format(original_string))
print("String without trailing quotes: {}".format(no_quotes_string))
In this example, we start with a string original_string
that ends with trailing quotes. The rstrip()
method is then applied to this string, with '"'
specified as the characters to be removed.
Consequently, only the trailing quotes on the right side are removed, leaving the content before the quotes unaltered. The output emphasizes how the rstrip()
method effectively trims quotes from the end of the specified string.
Output:
Original string: Pythonistas are excited about coding."
String without trailing quotes: Pythonistas are excited about coding.
Remove Quotes From a String in Python Using the eval()
Method
In certain scenarios, you might encounter situations where the content within the quotes is a valid Python expression. In order to handle such cases and remove the quotes while evaluating the expression, the eval()
method can be employed.
This method evaluates the expression passed to it as a string and returns the result.
The syntax for the eval()
method is as follows:
result = eval(expression)
Where:
expression
: The string containing a Python expression to be evaluated.
By calling eval()
on a string containing a Python expression, the method interprets and evaluates the expression, providing the result. In the context of removing quotes, the idea is to pass a string with quotes to eval()
, allowing it to evaluate and return the content without quotes.
Let’s see an example demonstrating the use of the eval()
method to remove quotes:
expression_with_quotes = "'Python Programming'"
result = eval(expression_with_quotes)
print("Original expression: {}".format(expression_with_quotes))
print("Expression without quotes: {}".format(result))
Here, we initialize a string expression_with_quotes
containing a Python expression within single quotes. The eval()
method is then applied to this string, effectively interpreting and evaluating the expression.
As a result, the quotes are removed, and the content within them is returned. After the evaluation, we print both the original expression and the expression without quotes using formatted strings.
Output:
Original expression: 'Python Programming'
Expression without quotes: Python Programming
It’s essential to note that using eval()
should be done cautiously, especially with untrusted input, to prevent security risks.
Remove Quotes From a String in Python Using String Slicing
In Python, an alternative and straightforward approach to removing quotes from a string involves using string slicing. This technique leverages the ability to extract a portion of a string by specifying start and end indices.
By defining the appropriate indices, you can effectively exclude the quotes from the desired substring. The syntax for string slicing is as follows:
new_string = original_string[start:end]
Where:
original_string
: The string from which you want to remove quotes.start
: The index from which the slicing begins.end
: The index up to which the slicing is performed (exclusive).
Let’s now look at an example demonstrating the use of string slicing to remove quotes:
original_string = '"Pythonic Wisdom"'
no_quotes_string = original_string[1:-1]
print("Original string: {}".format(original_string))
print("String without quotes: {}".format(no_quotes_string))
In this example, the string slicing operation is applied to the given string using [1:-1]
as the indices. Here, 1
represents the index of the second character (excluding the first quote), and -1
signifies the index of the second-to-last character (excluding the last quote).
Consequently, the resulting substring excludes both quotes, effectively removing them.
Output:
Original string: "Pythonic Wisdom"
String without quotes: Pythonic Wisdom
Remove Quotes From a String in Python Using Regular Expressions
Regular expressions provide a powerful tool for pattern matching and string manipulation. To remove quotes from a string, we can utilize the re
module along with regular expressions.
This method allows for more flexibility in handling various quote patterns within a string.
The syntax is as follows:
import re
new_string = re.sub(pattern, replacement, original_string)
Where:
pattern
: The regular expression pattern to match.replacement
: The string to replace the matched pattern.original_string
: The string from which quotes need to be removed.
By defining a regular expression pattern that matches quotes and using an empty string as the replacement, we can effectively remove the quotes from the original string.
Let’s look at an example demonstrating the use of regular expressions to remove quotes:
import re
original_string = '"Pythonistas Embrace Challenges"'
no_quotes_string = re.sub(r'["\']', "", original_string)
print("Original string: {}".format(original_string))
print("String without quotes: {}".format(no_quotes_string))
In this example, the re.sub()
method is applied to the original_string
using the regular expression r'["\']'
. This pattern matches both single and double quotes.
The second argument, an empty string ''
, serves as the replacement, effectively removing the matched quotes. This approach allows us to handle various types of quotes within the string.
After the substitution, we print both the original string and the string without quotes using formatted strings.
Output:
Original string: "Pythonistas Embrace Challenges"
String without quotes: Pythonistas Embrace Challenges
Remove Quotes From a String in Python Using a for
Loop With not in
For a more manual approach to removing quotes from a string in Python, we can use a for
loop in combination with the not in
condition. This method provides a clear and straightforward way to handle each character individually.
The basic idea is to iterate through each character in the original string and construct a new string by appending characters that are not quotes. The syntax is as follows:
new_string = ""
for char in original_string:
if char not in ['"', "'"]:
new_string += char
Where:
original_string
: The string from which quotes need to be removed.new_string
: The string being constructed without quotes.
In this loop, we check if each character is not a quote ('"'
or '
) and, if so, append it to the new string. This effectively creates a string without the quotes.
Let’s now look at an example demonstrating the use of a for loop with not in
to remove quotes:
original_string = '"Programming is an Art"'
new_string = ""
for char in original_string:
if char not in ['"', "'"]:
new_string += char
print("Original string: {}".format(original_string))
print("String without quotes: {}".format(new_string))
Here, we initiate a for
loop to iterate through each character in the original_string
. For each character, we check if it is not a quote ('"'
or '
).
If the character is not a quote, it is appended to the new_string
. This process is repeated for every character in the original string, effectively constructing a new string without quotes.
Output:
Original string: "Programming is an Art"
String without quotes: Programming is an Art
While this method may be more manual, it provides a transparent and explicit way to handle each character individually.
Remove Quotes From a String in Python Using the join()
Function With not in
Another concise and efficient way to remove quotes from a string in Python involves using the join()
function in combination with the not in
condition. This approach provides a more Pythonic and streamlined solution by leveraging the capabilities of the join()
function to construct the new string.
The idea is to use a generator expression within the join()
function to iterate through each character in the original string and include only those characters that are not quotes. The syntax is as follows:
new_string = "".join(char for char in original_string if char not in ['"', "'"])
Where:
original_string
: The string from which quotes need to be removed.new_string
: The string being constructed without quotes.
In this single line, the generator expression iterates through each character in original_string
, and only characters that are not quotes ('"'
or '
) are included in the construction of the new string.
Let’s look at an example demonstrating the use of the join()
function with not in
to remove quotes:
original_string = '"Pythonic Code is Readable"'
new_string = "".join(char for char in original_string if char not in ['"', "'"])
print("Original string: {}".format(original_string))
print("String without quotes: {}".format(new_string))
In this example, we use a single line of code to apply the join()
function to the generator expression that iterates through each character in the original_string
.
For each character, the condition if char not in ['"', "'"]
ensures that only characters not being quotes are included in the construction of the new_string
. This concise approach leverages the power of Python’s functional programming constructs.
After applying the method, we print both the original string and the string without quotes.
Output:
Original string: "Pythonic Code is Readable"
String without quotes: Pythonic Code is Readable
Remove Quotes From a String in Python Using the startswith()
Function With Slicing
In Python, the combination of the startswith()
function and string slicing also provides an effective approach to removing quotes from the beginning of a string.
The startswith()
function is used to check if a string starts with a specified prefix. In combination with slicing, we can then remove the quotes from the beginning of the string.
The syntax is as follows:
new_string = (
original_string[len(prefix) :]
if original_string.startswith(prefix)
else original_string
)
Where:
original_string
: The string from which quotes need to be removed.prefix
: The string to check for at the beginning oforiginal_string
.new_string
: The resulting string without quotes.
In this line of code, startswith()
checks if original_string
starts with the specified prefix. If true, slicing is used to create new_string
by excluding the prefix; otherwise, new_string
remains the same as original_string
.
Let’s now look at an example demonstrating the use of the startswith()
function with slicing to remove quotes:
original_string = '"Begin with a Single Step"'
prefix = '"'
new_string = (
original_string[len(prefix) :]
if original_string.startswith(prefix)
else original_string
)
print("Original string: {}".format(original_string))
print("String without starting quotes: {}".format(new_string))
In this example, we use a conditional expression to check if original_string
starts with the specified prefix ("
).
If true, the slicing operation original_string[len(prefix):]
is executed to create new_string
by excluding the prefix. If false, new_string
remains the same as original_string
.
This method efficiently handles the removal of quotes, specifically from the beginning of the string.
Output:
Original string: "Begin with a Single Step"
String without starting quotes: Begin with a Single Step"
This method is particularly useful when dealing with strings where quotes may appear only at the start.
Remove Quotes From a String in Python Using the endswith()
Function with Slicing
In Python, the combination of the endswith()
function and string slicing also offers an effective method to remove quotes from the end of a string.
The endswith()
function is employed to check if a string ends with a specified suffix. When combined with slicing, we can then remove the quotes from the end of the string.
The syntax is as follows:
new_string = (
original_string[: -len(suffix)]
if original_string.endswith(suffix)
else original_string
)
Where:
original_string
: The string from which quotes need to be removed.suffix
: The string to check for at the end oforiginal_string
.new_string
: The resulting string without quotes.
In this line of code, endswith()
checks if original_string
ends with the specified suffix. If true, slicing is used to create new_string
by excluding the suffix; otherwise, new_string
remains the same as original_string
.
Let’s have an example presenting the use of the endswith()
function with slicing to remove quotes:
original_string = 'Silence is golden"'
suffix = '"'
new_string = (
original_string[: -len(suffix)]
if original_string.endswith(suffix)
else original_string
)
print("Original string: {}".format(original_string))
print("String without ending quotes: {}".format(new_string))
Similar to the previous example, we use a conditional expression to check if original_string
ends with the specified suffix ("
).
If true, the slicing operation original_string[:-len(suffix)]
is executed to create new_string
by excluding the suffix. If false, new_string
remains the same as original_string
.
This code efficiently handles the removal of quotes specifically from the end of the string.
Output:
Original string: Silence is golden"
String without ending quotes: Silence is golden
Remove Quotes From a String in Python Using the ast.literal_eval()
Function
The ast
module provides a powerful function called literal_eval()
that can be used to safely evaluate and parse strings containing literal Python expressions. By leveraging this function, we can also effectively remove quotes from a string, considering the string as a valid Python expression.
The ast.literal_eval()
function takes a string as input and safely evaluates it as a Python literal or container display expression. The syntax is as follows:
import ast
result = ast.literal_eval(string)
Where:
string
: The string to be evaluated.
In the context of removing quotes, if the supplied string is a valid Python expression enclosed in quotes, literal_eval()
will return the evaluated expression without the quotes.
Below is an example demonstrating the use of ast.literal_eval()
to remove quotes:
import ast
expression_with_quotes = "'Pythonic Wisdom'"
result = ast.literal_eval(expression_with_quotes)
print("Original expression: {}".format(expression_with_quotes))
print("Expression without quotes: {}".format(result))
In this example, we first import the ast
module and use the literal_eval()
function to evaluate the string expression_with_quotes
. The string contains a valid Python expression enclosed in single quotes.
Here, the literal_eval()
function safely evaluates the expression, effectively removing the quotes and returning the result.
Output:
Original expression: 'Pythonic Wisdom'
Expression without quotes: Pythonic Wisdom
It’s important to note that this method is particularly useful when dealing with strings that represent valid Python literals.
Conclusion
The diverse methods explored in this article offer flexibility when it comes to removing quotes from strings. Depending on your needs and the context of your project, you can choose a method that aligns with the complexity of the task at hand.
Whether you opt for string slicing, regular expressions, or ast.literal_eval()
, the goal remains the same: to efficiently and cleanly extract content from strings, leaving you with processed data ready for further analysis or manipulation.
With these techniques, you are well-equipped to handle a variety of scenarios involving strings and quotes in Python.