Python で文字列から引用符を削除する
Azaz Farooq
2023年1月30日
Python
Python String
-
Python の
replace()
メソッドを使って文字列から引用符を削除する -
Python の
strip()
メソッドを用いた文字列からの引用符の削除 -
Python で
lstrip()
メソッドを使用しての文字列からの引用符の削除 -
Python で
rstrip()
メソッドを使用して文字列から引用符を削除する -
Python の
literal_eval()
メソッドを用いた文字列からの引用符の削除
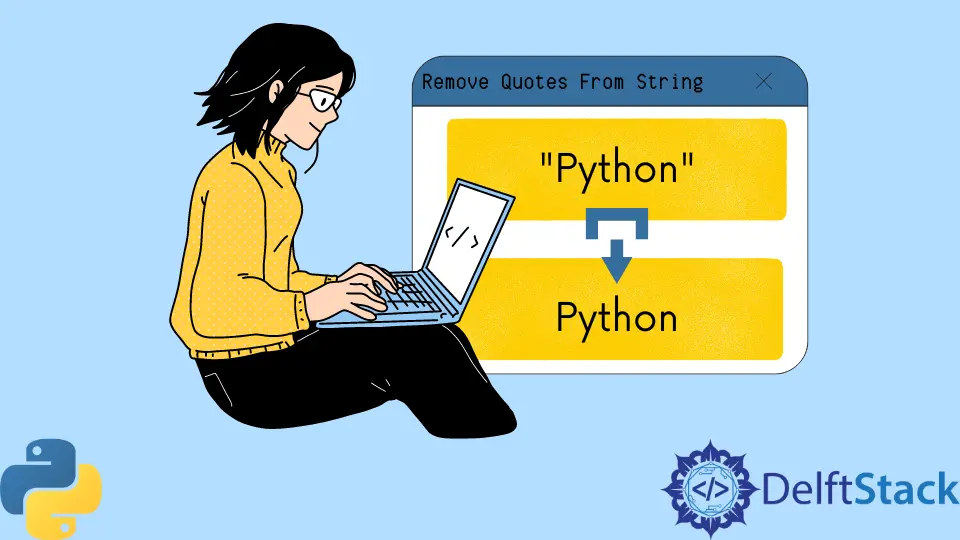
単一の ''
または二重の ""
引用符で囲まれた文字の組み合わせは、文字列と呼ばれます。この記事では、Python で文字列から引用符を削除するさまざまな方法を紹介します。
Python の replace()
メソッドを使って文字列から引用符を削除する
このメソッドは 2つの引数を取ります。古い文字列を '""'
とし、新しい文字列を ""
(空の文字列) とした replace()
を呼び出すことで、すべての引用符を削除することができます。
完全なサンプルコードは以下の通りです。
old_string = '"python"'
new_string = old_string.replace('"', "")
print("The original string is - {}".format(old_string))
print("The converted string is - {}".format(new_string))
出力:
The original string is - "python"
The converted string is - python
Python の strip()
メソッドを用いた文字列からの引用符の削除
このメソッドでは、文字列の両端から引用符を削除します。この関数の引数に引用符 '""'
を渡すと、古い文字列の両端から引用符を削除し、引用符のない new_string
を生成します。
完全なサンプルコードは以下の通りです。
old_string = '"python"'
new_string = old_string.strip('"')
print("The original string is - {}".format(old_string))
print("The converted string is - {}".format(new_string))
出力:
The original string is - "python"
The converted string is - python
Python で lstrip()
メソッドを使用しての文字列からの引用符の削除
このメソッドは、文字列の先頭に引用符が現れた場合に引用符を削除します。文字列の先頭に引用符がある場合に適用されます。
完全なサンプルコードは以下の通りです。
old_string = '"python'
new_string = old_string.lstrip('"')
print("The original string is - {}".format(old_string))
print("The converted string is - {}".format(new_string))
Output.
The original string is - "python
The converted string is - python
Python で rstrip()
メソッドを使用して文字列から引用符を削除する
このメソッドは、文字列の最後に引用符が表示された場合、引用符を削除します。パラメータが渡されていない場合に削除されるデフォルトの末尾文字は空白です。
完全なサンプルコードは以下の通りです。
old_string = 'python"'
new_string = old_string.rstrip('"')
print("The original string is - {}".format(old_string))
print("The converted string is - {}".format(new_string))
出力:
The original string is - python"
The converted string is - python
Python の literal_eval()
メソッドを用いた文字列からの引用符の削除
このメソッドは、Python リテラルまたはコンテナビュー式ノード、Unicode または Latin-1 エンコードされた文字列をテストします。与えられた文字列やノードは、以下の Python リテラル構造体のみから構成されます: 文字列、数値、タプル、リスト、辞書、ブーリアンなど。これは、信頼されていない Python の値を含む文字列を、値自体を調べることなく安全にテストします。
完全なサンプルコードは以下の通りです。
string = "'Python Programming'"
output = eval(string)
print(output)
出力:
Python Programming
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe