Python Regex group() Function
-
Use the
re.MatchObject.group()
Function to Provide the Exact Subgroup in Python -
Use
re.match()
to Find the Match Among the Subgroups in Python
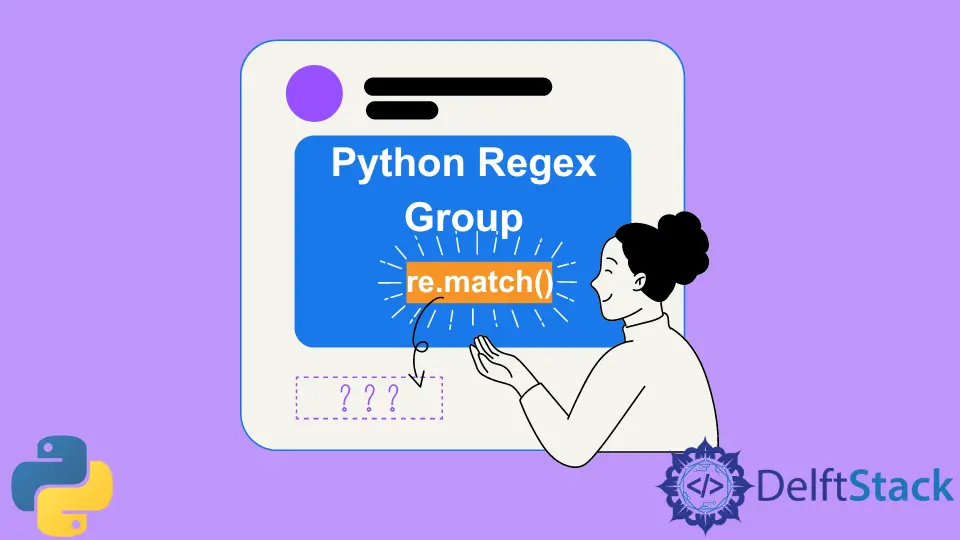
The regex group function, or the re.MatchObject.group()
function, is a function that provides the exact subgroup as a result by matching it with the argument passed in the function.
This tutorial discusses the regex group function and how to implement it in Python.
Use the re.MatchObject.group()
Function to Provide the Exact Subgroup in Python
The re
library needs to be imported to the Python code to utilize this function. The re
library, which is an abbreviation of the term Regular Expression
, is a library that is utilized to deal with Regular Expressions in Python.
The syntax for the re.MatchObject.group()
function is described below for ease of understanding.
re.MatchObject.group([group])
The function contains only one optional parameter that takes in the argument, which is utilized to match with the subgroups. The given function has a default value of zero.
It can return three possible outcomes, which are:
- If a valid argument is taken, it returns the complete subgroup that has been matched with the argument(s).
- If the group number is taken in as the argument is either greater than the number of groups or negative, the output would be an
IndexError
. - If it cannot find a pattern that matches the argument, then an
AttributeError
is raised.
The following example code shows how to implement the regex group function in Python.
Use re.match()
to Find the Match Among the Subgroups in Python
import re
matchobj1 = re.match(r"(\w+)@(\w+)\.(\w+)", "admin@delftstack.com")
print(matchobj1.group(1))
print(matchobj1.group(2))
print(matchobj1.group(3))
Output:
admin
delftstack
com
In the above code, the re.match()
is utilized to find the matches among the subgroups of the given text admin@delftstack.com
.
The w
sign in the above code indicates that a letter out of the English alphabet is to be considered for the matching purpose, while the +
sign indicates that the search is for continuous characters.
It is a case where the process successfully gets completed, and a match is found. However, that is not always the case.
The below code is an example of how an IndexError
may happen sometimes.
import re
matchobj1 = re.match(r"(\w+)@(\w+)\.(\w+)", "admin@delftstack.com")
print(matchobj1.group(5))
Output:
Traceback (most recent call last):
File "<string>", line 3, in <module>
IndexError: no such group
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn